Python Tutorial: Web Scraping with BeautifulSoup and Requests
TLDRThe video script offers a comprehensive guide on web scraping using the BeautifulSoup library in Python. It explains the process of parsing website content to extract specific information, such as headlines, summaries, and video links. The tutorial demonstrates how to install necessary libraries, navigate HTML structure, and handle various tags and attributes. It also covers error handling and the importance of being considerate when scraping to avoid overloading servers. The example provided involves scraping a personal website and saving the extracted data into a CSV file, showcasing BeautifulSoup's practical application.
Takeaways
- π Web scraping involves parsing website content to extract specific information.
- π The BeautifulSoup library in Python simplifies the process of web scraping.
- π To scrape data, it's helpful to understand the structure of HTML and how information is tagged.
- π The 'find' and 'find_all' methods in BeautifulSoup are used to locate and extract specific elements from HTML.
- π οΈ The 'requests' library is used to make HTTP requests to fetch website content.
- ποΈ Use 'pip install beautifulsoup4' and 'pip install requests' to install the necessary libraries for web scraping.
- π Attributes like 'class', 'id', and 'href' are crucial for identifying and selecting specific tags in the HTML.
- π Handle missing data gracefully using try-except blocks to avoid breaking the scraper.
- π Data scraped can be saved in various formats like CSV for further analysis or usage.
- π Public APIs are preferred for large websites like Twitter, Facebook, or YouTube to access data efficiently and responsibly.
- π Always be considerate when scraping to avoid overloading servers or violating website terms of service.
Q & A
What does web scraping involve?
-Web scraping involves parsing the content from websites and extracting specific information in a structured and readable format.
What is BeautifulSoup and how does it help in web scraping?
-BeautifulSoup is a Python library that helps in web scraping by making it easier to parse and navigate HTML and XML documents. It allows users to extract data efficiently from web pages.
What are some potential uses of web scraping?
-Potential uses of web scraping include pulling headlines from news sites, grabbing sports scores, monitoring item prices in online stores, and extracting video summaries and links from personal websites.
What are the differences between parsers used in BeautifulSoup and when might they matter?
-Different parsers have slight variations in how they handle HTML parsing. They might matter when dealing with imperfectly formed HTML, as they attempt to fill in missing information differently. BeautifulSoup documentation suggests using the LXML parser for most use cases.
How does one install BeautifulSoup and the required parsers?
-To install BeautifulSoup, use the pip install command with 'beautifulsoup4'. For parsers, 'lxml' can be installed using 'pip install lxml', and 'html5lib' can be installed using 'pip install html5lib'.
What is the role of the 'requests' library in web scraping?
-The 'requests' library is used to make HTTP requests to fetch websites' content. It is popular for fetching web pages due to its simplicity and ease of use.
How does one structure a Python script to scrape information from a website?
-A typical web scraping script involves first installing necessary libraries, fetching the web page content using 'requests', parsing the HTML with BeautifulSoup, and then navigating and extracting the required information using BeautifulSoup's methods.
How can you handle missing data when scraping with Python?
-To handle missing data, you can use try-except blocks to catch exceptions that arise when data is not found. Within the except block, you can set the variable to 'None' or apply other fallback measures to ensure the script continues running.
What is an API and how does it relate to web scraping?
-An API (Application Programming Interface) is a set of rules that allows different software applications to communicate with each other. Some large websites offer public APIs to provide data in a structured and efficient manner, which can be a preferable alternative to manual web scraping.
What are some considerations to keep in mind when scraping websites?
-When scraping websites, it's important to be considerate of the website's server load, as scraping can generate a high volume of requests. Additionally, respect the website's terms of service and legal boundaries regarding data extraction.
How can scraped data be saved and utilized after extraction?
-Extracted data can be saved in various formats such as CSV, JSON, or databases for further analysis or usage. Python provides libraries like 'csv' for handling CSV files, making it easy to store and organize scraped data.
Outlines
π Introduction to Web Scraping with BeautifulSoup
This paragraph introduces the concept of web scraping, explaining that it involves parsing website content to extract specific information. The speaker provides an example of scraping their personal website for post titles, summaries, and video links, and demonstrates a finished scraper that outputs this data in both the terminal and a CSV file. The paragraph also discusses the BeautifulSoup library and its utility in simplifying the parsing process, as well as the request library for making web requests.
π Understanding HTML Structure for Web Scraping
The speaker explains the importance of understanding HTML structure for effective web scraping. Using a basic HTML file as an example, the paragraph details how information is contained within specific tags. It covers the concept of opening and closing tags, nested tags, and the use of classes for CSS styling and JavaScript identification. The speaker also demonstrates how to use BeautifulSoup to parse a simple HTML file and extract information like article headlines and summaries.
π Locating and Extracting Data with BeautifulSoup
This paragraph delves into the mechanics of using BeautifulSoup for data extraction. It explains how to use the 'find' and 'find_all' methods to locate specific tags and attributes within the HTML structure. The speaker illustrates the process with examples of extracting the title of an HTML page, searching for tags with specific classes, and parsing out article headlines and summaries from a sample website.
π οΈ Parsing Real Website Data with BeautifulSoup
The speaker transitions from the example of a simple HTML file to parsing a real website. The paragraph focuses on obtaining the source code of the website using the request library and BeautifulSoup for parsing. It describes the process of inspecting website elements to identify the HTML structure containing the desired data, such as video headlines, summaries, and embedded videos. The speaker demonstrates how to extract this information from the website's HTML and presents a method for handling missing data with try-except blocks.
π Saving Scraped Data to a CSV File
In this paragraph, the speaker discusses how to save the scraped data to a CSV file. After explaining the process of writing data to a file, the speaker provides a step-by-step guide on using the CSV module to open a file, write headers, and append the scraped data for each article. The paragraph concludes with a demonstration of how the data appears in a spreadsheet application, emphasizing the utility of organizing scraped data for further analysis or use.
π€ Ethical Considerations and Conclusion
The speaker concludes the tutorial by discussing ethical considerations when scraping websites. They mention the importance of being considerate with the number of requests sent to avoid overloading servers and the potential use of public APIs for larger websites. The paragraph also encourages viewers to ask questions, share the video, and support the content creator through likes, shares, and Patreon contributions.
Mindmap
Keywords
π‘Web Scraping
π‘BeautifulSoup
π‘HTML
π‘Parsing
π‘Request Library
π‘CSV
π‘Python
π‘Data Extraction
π‘Try-Except Block
π‘API
Highlights
The video introduces the concept of web scraping and its applications, such as extracting headlines, sports scores, or online store prices.
BeautifulSoup is a Python library used for parsing HTML and XML documents, making it easier to scrape web content.
The video demonstrates how to install BeautifulSoup and the necessary parsers (lxml and html5lib) using pip commands.
An example scraper called 'CMS scrape pie' is showcased, which can extract titles, summaries, and video links from a personal website and output the data in a CSV file.
The video explains the importance of understanding HTML structure and tags when scraping web pages.
The process of using the 'requests' library to fetch a website's HTML content is detailed.
A step-by-step guide on parsing a website's HTML structure using BeautifulSoup is provided, including finding tags by attributes and classes.
The video demonstrates how to extract specific information from a webpage, such as article headlines and summaries, using BeautifulSoup's 'find' and 'find_all' methods.
A practical example of scraping a real website (the presenter's personal website) is given, showing how to extract video titles, summaries, and YouTube links.
The video addresses the potential issue of missing data and demonstrates how to handle it using try-except blocks to prevent script failure.
The process of saving scraped data to a CSV file is explained, including handling and writing data to the file.
The video emphasizes the importance of being considerate when scraping websites to avoid overloading servers and potential blocking by the website.
Public APIs for larger websites like Twitter, Facebook, and YouTube are mentioned as a more efficient and preferred method for data extraction.
The video concludes with a call to action for viewers to like, share, and support the content for future tutorials.
Transcripts
Browse More Related Video

Web Scraping to CSV | Multiple Pages Scraping with BeautifulSoup
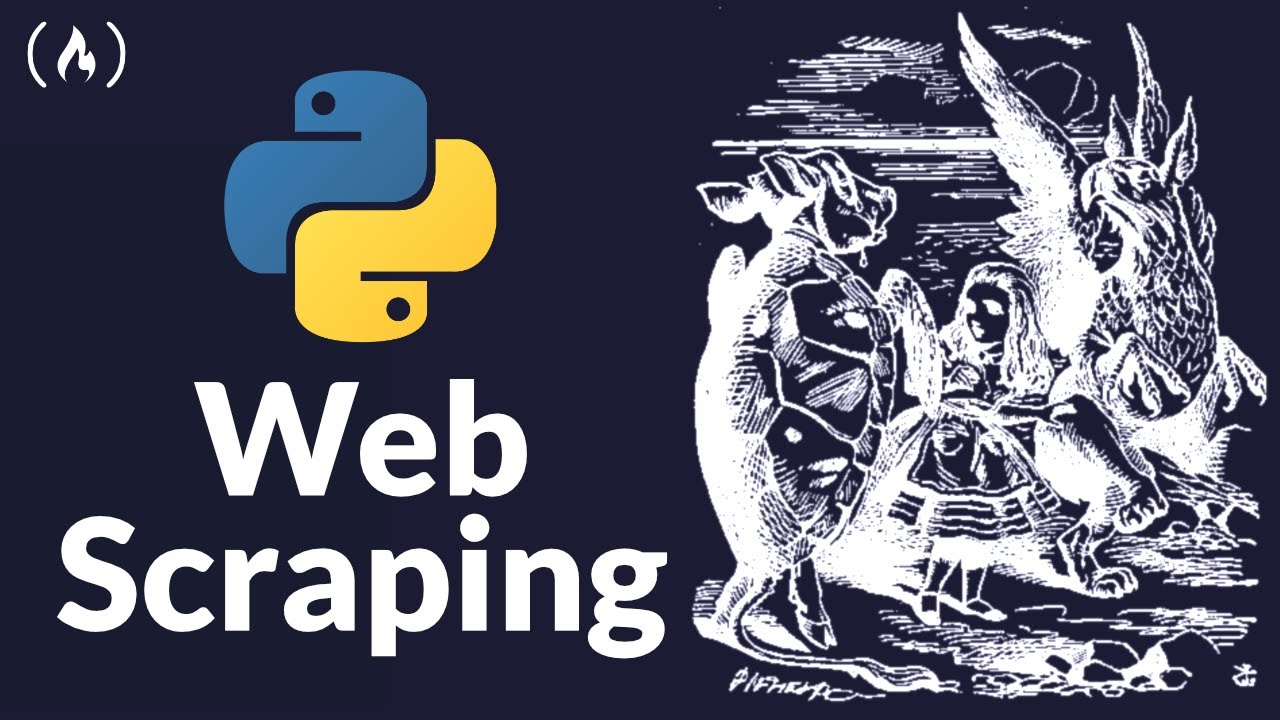
Web Scraping with Python - Beautiful Soup Crash Course

Beautiful Soup 4 Tutorial #1 - Web Scraping With Python
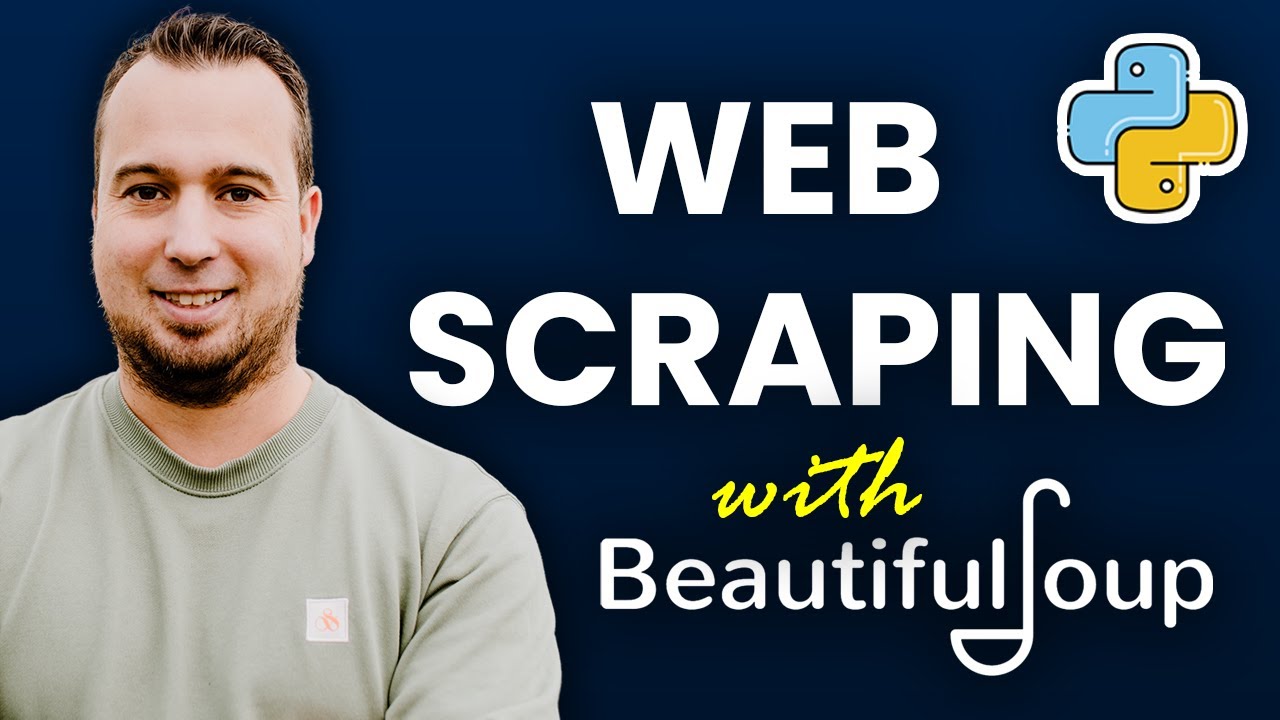
Web Scraping with Python and BeautifulSoup is THIS easy!
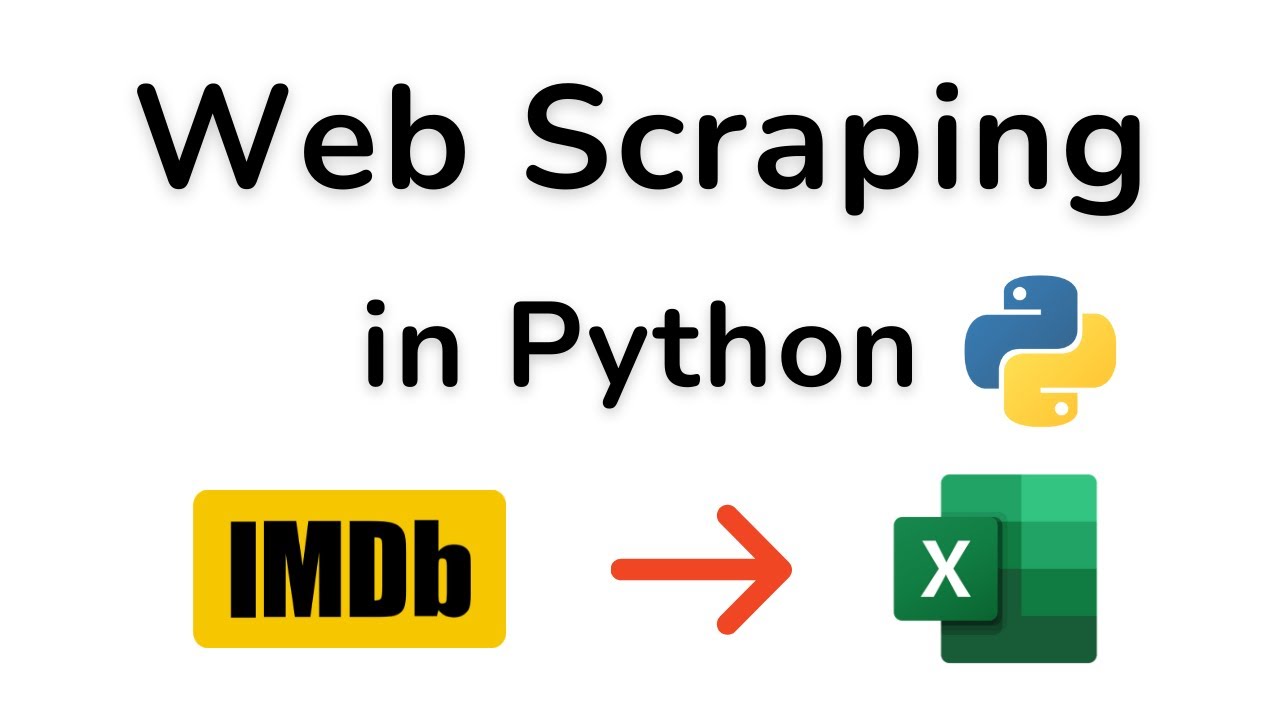
Web Scraping in Python using Beautiful Soup | Writing a Python program to Scrape IMDB website

Find and Find_All | Web Scraping in Python
5.0 / 5 (0 votes)
Thanks for rating: