Web Scraping in Python using Beautiful Soup | Writing a Python program to Scrape IMDB website
TLDRThis video tutorial demonstrates how to perform web scraping in Python using the Beautiful Soup module. The presenter guides viewers through the process of writing a Python program to extract top-rated movies from IMDb and load the data into an Excel file. Key concepts include installing necessary modules, parsing HTML content, and handling data extraction with Beautiful Soup. The video is a practical guide for beginners interested in Python, web scraping, and data analytics.
Takeaways
- π Web scraping is the process of programmatically extracting data from websites.
- π§ The Python script uses the 'requests' module to access websites and 'BeautifulSoup' to parse HTML content.
- π The video demonstrates how to extract information from IMDb's 'Top Rated Movies' list and load it into an Excel file.
- π οΈ Basic knowledge of HTML is necessary to understand how data is structured within tags.
- π The script identifies and accesses specific HTML tags (e.g., 'tr', 'td', 'a', 'span', 'strong') to extract movie details.
- π A 'try-except' block is used to handle potential errors when accessing websites with the 'requests' module.
- π The 'BeautifulSoup' object is used to parse the HTML content and extract the desired data.
- π’ The script iterates through each 'tr' tag to collect the rank, movie name, year of release, and IMDb rating.
- π The 'openpyxl' module is used to create a new Excel file and append data to it.
- πΎ The final Excel file is saved with a specified name (e.g., 'imdb_movie_ratings.xlsx') after data extraction.
- πΊ The video provides a step-by-step guide, suitable for beginners interested in web scraping with Python.
Q & A
What is web scraping and how does it work?
-Web scraping is the process of programmatically extracting data from a website. It involves using a program to access a website, parse its HTML content, and retrieve specific pieces of information based on the structure of the webpage.
What are the two main Python modules required for web scraping as discussed in the video?
-The two main Python modules required for web scraping in this context are 'requests' and 'BeautifulSoup'. The 'requests' module is used to access the website, while 'BeautifulSoup' is used to parse the HTML content of the website.
How does the 'requests' module function in web scraping?
-The 'requests' module is used to send HTTP requests to a website. It can access the website's content and return a response object that contains the HTML source code of the webpage, which can then be parsed by 'BeautifulSoup'.
What is the purpose of the 'BeautifulSoup' module in web scraping?
-The 'BeautifulSoup' module is used to parse the HTML content that is retrieved by the 'requests' module. It simplifies the process of navigating and searching the parse tree, and it also provides methods to access different tags and their attributes within the HTML content.
How does one handle errors in web scraping, such as an invalid URL?
-To handle errors such as an invalid URL, it is recommended to use a 'try-except' block in Python. This allows the program to catch exceptions that may occur when the 'requests' module fails to access the website and prevents the program from crashing.
What is the significance of understanding HTML tags when performing web scraping?
-Understanding HTML tags is crucial for web scraping because data on websites is structured within these tags. Knowing how to identify and navigate through different tags and their attributes is essential for locating and extracting the desired information from the HTML content.
How does the video demonstrate the process of extracting movie details from IMDb?
-The video demonstrates the process by first accessing the IMDb website using the 'requests' module, then parsing the HTML content with 'BeautifulSoup'. It shows how to identify the specific HTML tags and their structure that contain the movie details such as rank, name, year of release, and IMDb rating. The video then provides a step-by-step guide on how to write Python code to extract this information and load it into an Excel file.
What is the role of the 'openpyxl' module in the context of this video?
-The 'openpyxl' module is used to create and manipulate Excel files in Python. In the video, it is used to create a new Excel file, add headings and rows with movie details, and then save the file, thus providing a way to store the scraped data in a structured format.
How does the video ensure that the scraped data is accurately loaded into the Excel file?
-The video ensures accurate data loading by iterating through each movie's details, extracting the relevant information using 'BeautifulSoup', and then appending it to the Excel file using the 'openpyxl' module. It also demonstrates the creation of column headers before loading the scraped data to ensure proper organization of the information.
What is the importance of using a 'try-except' block when saving the Excel file?
-Using a 'try-except' block when saving the Excel file is important to handle any potential errors that may occur during the saving process, such as file access issues or invalid file paths. This ensures that the program does not crash and provides a way to handle exceptions gracefully.
What are some best practices for web scraping demonstrated in the video?
-Some best practices for web scraping demonstrated in the video include handling exceptions to manage errors, using a 'try-except' block to catch errors, understanding the structure of the HTML content to accurately extract data, and saving the scraped data in a structured format like an Excel file for further analysis.
Outlines
π Introduction to Web Scraping with Python and Beautiful Soup
The paragraph introduces the concept of web scraping and outlines a step-by-step guide on how to perform it using Python and the Beautiful Soup module. The speaker explains that web scraping is the process of programmatically extracting data from websites. The video specifically demonstrates how to write a Python program to access and extract information from the IMDb website, such as top-rated movies, and load it into an Excel file. The prerequisites for this process are the installation of two modules: 'requests' for accessing the website and 'beautifulsoup4' for parsing the HTML content. The speaker emphasizes the ease of parsing HTML with Beautiful Soup and the basic understanding required of HTML tags to follow along with the tutorial.
π οΈ Setting Up the Environment for Web Scraping
This paragraph delves into the technical setup required for web scraping. It instructs the audience on how to install the necessary Python modules, 'requests' and 'beautifulsoup4', using pip3 on a Mac or pip on Windows. The speaker also explains the use of the 'requests' module to access a website and handle potential errors by using a try-except block with 'raise for status' to catch URL issues. The importance of error handling is emphasized to prevent the program from crashing when encountering an inaccessible URL. The paragraph also covers the extraction of the HTML source code using the 'requests' module and parsing it with Beautiful Soup to prepare for data extraction.
π Identifying and Extracting Data from IMDb
The speaker explains the process of identifying the specific HTML elements containing the desired data on the IMDb website. By using the browser's 'inspect' feature, the speaker demonstrates how to locate the HTML tags associated with the movie's rank, name, year of release, and IMDb rating. The paragraph details the use of Beautiful Soup to navigate and extract data from these tags. The speaker also provides a basic overview of HTML tags and attributes, emphasizing the need to understand how data is structured within tags to effectively perform web scraping. The paragraph sets the stage for writing the Python script to extract the top 250 movies from IMDb.
π Accessing and Iterating IMDb Data with Python
The paragraph describes the Python code used to access the IMDb website's data. It begins by using the 'requests' module to fetch the HTML content of the page and then parsing it with Beautiful Soup. The speaker outlines the process of finding the parent 'tbody' tag that contains all the movie information and then iterating through each 'tr' tag to access individual movie details. The paragraph explains the use of Beautiful Soup's 'find_all' method to locate multiple tags and the importance of understanding the HTML structure to accurately extract the required data. The speaker also discusses the use of loops to iterate over the extracted data and the subsequent steps to access specific information such as movie names, ranks, and ratings.
π¬ Extracting Individual Movie Details
This paragraph focuses on the detailed process of extracting specific movie details from the IMDb web page using Python. The speaker demonstrates how to access the 'td' tag with the class 'title column' to find the movie name and rank. The use of 'find' and 'text' methods from Beautiful Soup is explained to extract the exact movie name from the anchor 'a' tag within the 'td' tag. The paragraph also covers the extraction of the movie's year of release and IMDb rating by accessing the appropriate 'td' tags with the classes 'rating column imdb rating' and 'title column', respectively. The speaker provides a clear explanation of handling the text content, including stripping unnecessary characters and splitting text at specific delimiters to isolate the rank.
π Loading Scraped Data into an Excel File
The final paragraph discusses the process of loading the scraped movie data into an Excel file using the 'openpyxl' library in Python. The speaker guides the audience through creating a new Excel workbook, setting the active sheet, and renaming the sheet to 'Top Rated Movies'. The paragraph explains how to add column headers to the Excel sheet and subsequently append the scraped movie details to the sheet within a loop. The process concludes with saving the Excel file, resulting in a neatly organized spreadsheet with the extracted data. The speaker emphasizes the simplicity of creating and populating an Excel file with scraped data, providing a practical application of the web scraping techniques discussed in the video.
Mindmap
Keywords
π‘Web Scraping
π‘Python
π‘Beautiful Soup
π‘IMDb
π‘Excel File
π‘Request Module
π‘HTML
π‘Parsing
π‘Data Extraction
π‘Openpyxl
Highlights
The video demonstrates how to perform web scraping in Python using the Beautiful Soup module.
Web scraping is the process of extracting data from a website programmatically.
The video tutorial focuses on extracting the top-rated movies from the IMDb website and loading the data into an Excel file.
Two Python modules are required for this task: the request module and the Beautiful Soup module.
The request module is used to access the website, while Beautiful Soup is used to parse the HTML content.
Basic knowledge of HTML is sufficient to perform web scraping, focusing on understanding tags and their attributes.
The video provides a step-by-step guide on writing a Python program from scratch to perform the web scraping task.
The program begins by installing the necessary Python modules using pip3 install command for Mac or pip install for Windows.
The video emphasizes the importance of using try and except blocks to handle errors when accessing websites with the request module.
Beautiful Soup makes it easy to parse HTML by providing various methods to access different tags within the HTML content.
The video shows how to access and extract specific information, such as movie names, ranks, years of release, and IMDb ratings from the IMDb website.
The process involves identifying the correct HTML tags and attributes that contain the desired data by inspecting the web page's source code.
The video demonstrates how to loop through each movie entry, extract the required details, and print them for verification.
Finally, the video explains how to use the openpyxl module to create a new Excel file and load the scraped data into it.
The video concludes by showing the successfully created Excel file with the extracted movie data and encourages viewers to like and subscribe for more content.
Transcripts
Browse More Related Video

Beautiful Soup 4 Tutorial #1 - Web Scraping With Python
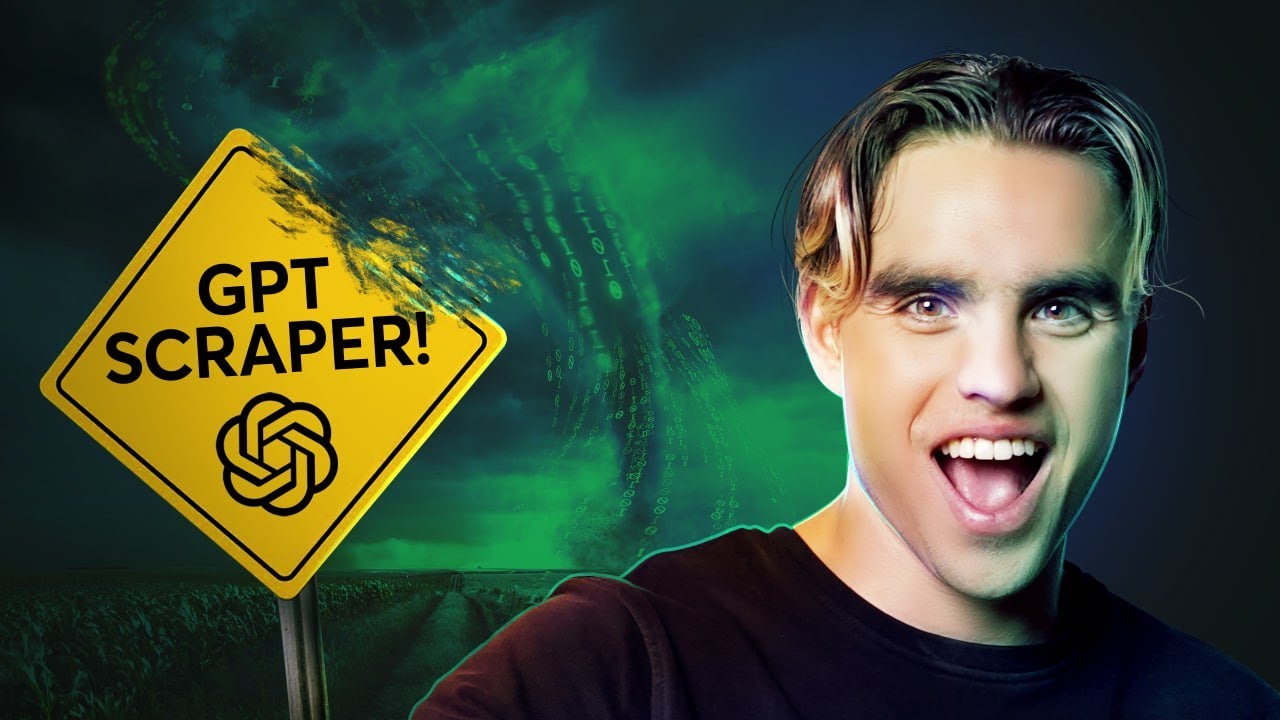
How To Scrape Websites With ChatGPT (As A Complete Beginner)
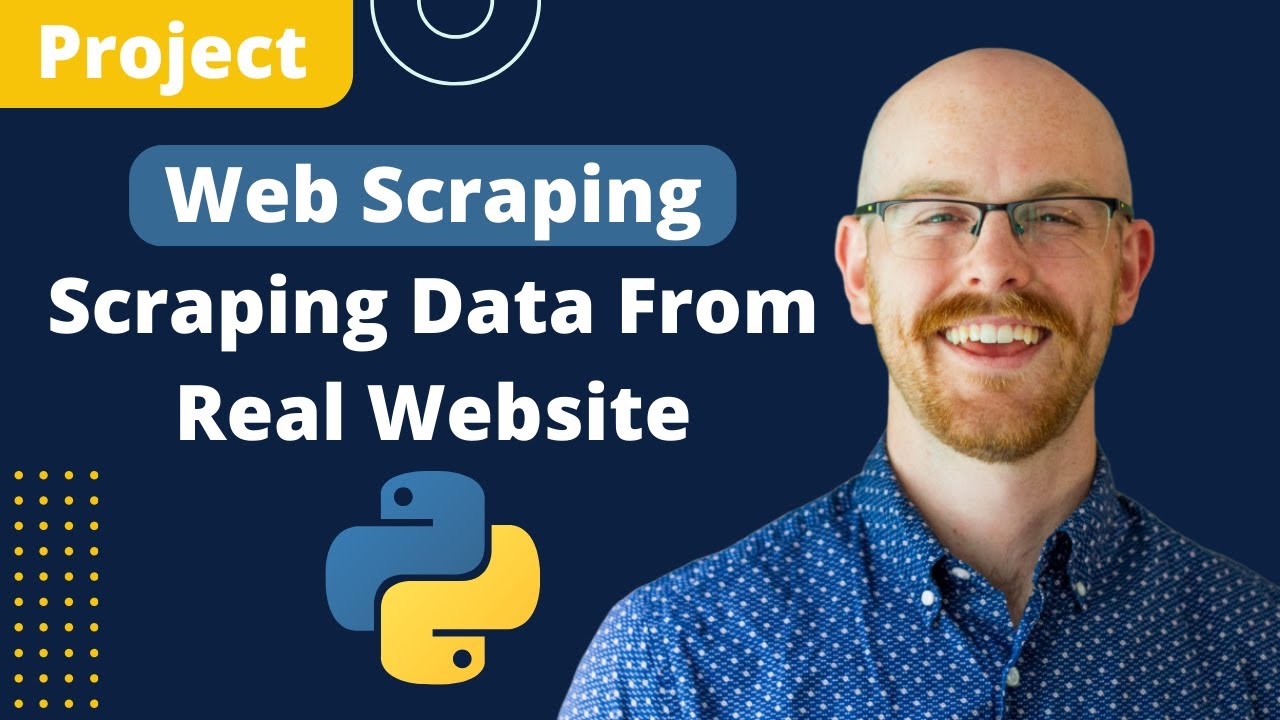
Scraping Data from a Real Website | Web Scraping in Python
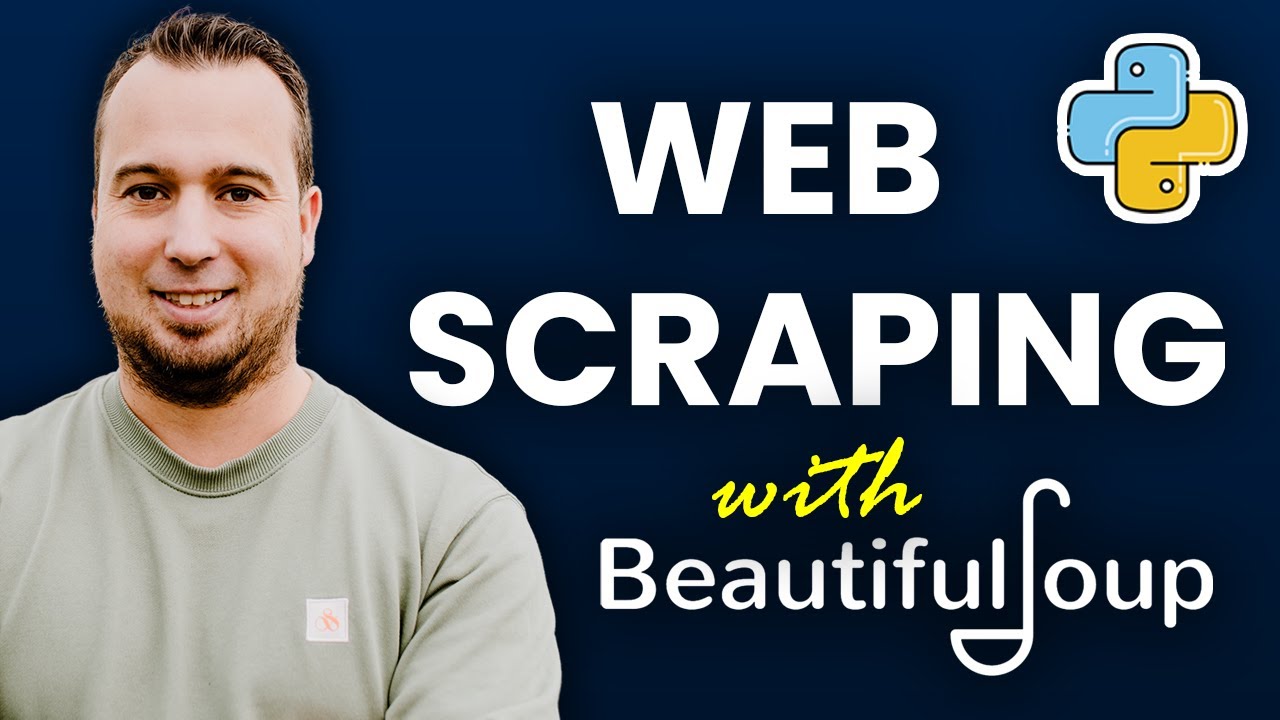
Web Scraping with Python and BeautifulSoup is THIS easy!

Web Scraping to CSV | Multiple Pages Scraping with BeautifulSoup
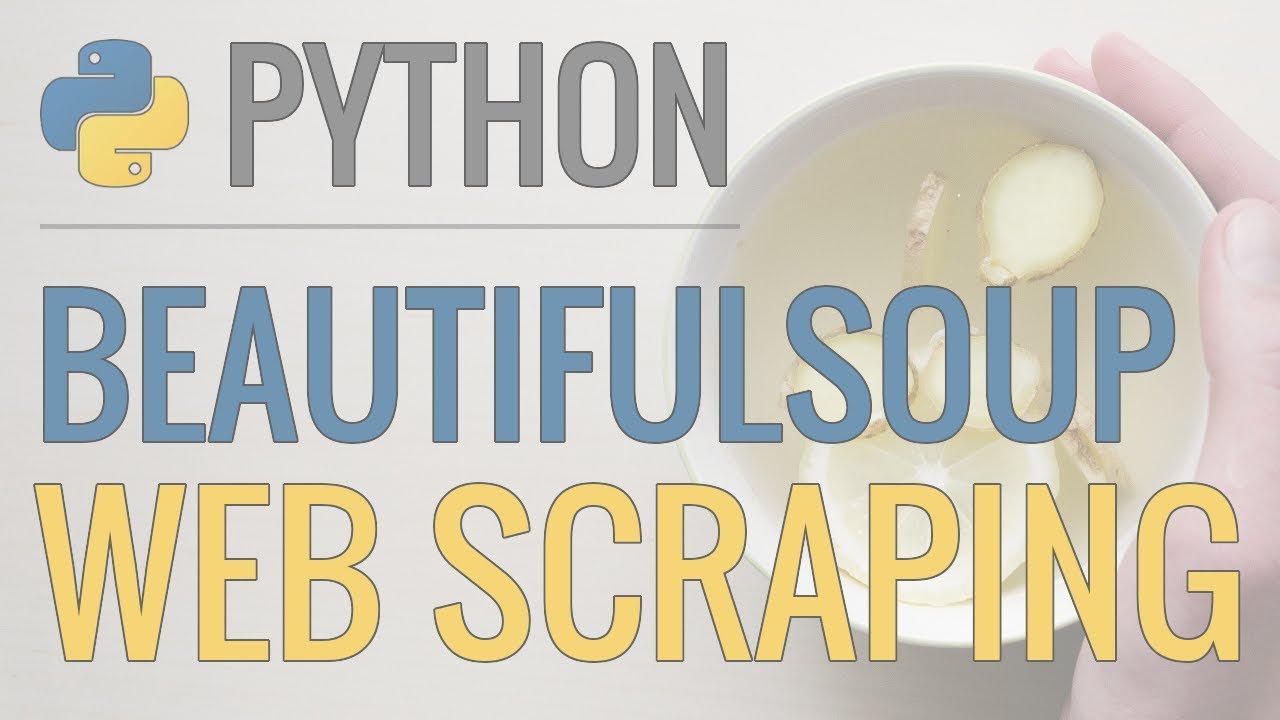
Python Tutorial: Web Scraping with BeautifulSoup and Requests
5.0 / 5 (0 votes)
Thanks for rating: