Python Tutorial for Absolute Beginners #1 - What Are Variables?
TLDRThe video introduces Python programming for beginners. It overviews installing Python and Jupyter Notebook, creating a first program, using the print function, variables for numbers and strings, reassigning variables, and swapping variable values. It aims to teach fundamental building blocks like comments, strings, numbers, print statements, and variables to allow viewers to start writing basic Python programs.
Takeaways
- π Python is a popular programming language used by many companies and universities
- π Jupyter Notebook provides a simple interface for writing and testing Python code
- π Variables allow you to store values like numbers and text in Python
- π You can swap the values of two variables without repeating their contents
- π₯ To run Python, install it along with Jupyter Notebook using Anaconda
- π» Launch Jupyter Notebook through Anaconda Navigator after installation
- π Jupyter Notebook lets you organize your code in folders and notebook files
- βοΈ Each code cell in a Jupyter notebook runs independently when executed
- π§ Fix errors carefully - small typos can prevent Python code from running
- π Master variables and swapping them to advance to more Python skills
Q & A
What is Python and what are some of its key advantages?
-Python is a popular programming language used by many companies and universities. Some of its key advantages are that it has simple syntax, is easy to learn, and can be used for website backend development, data analysis, scientific research, and more.
What is Jupyter Notebook and why is it used in this tutorial series?
-Jupyter Notebook is an environment for writing and testing Python code quickly. It is popular with the scientific community for data analysis. It is used in this tutorial series because it is simple and easy to install and use.
What are the two main components of Jupyter Notebook?
-The two main components are: 1) The Jupyter Notebook server, which is the core and runs in the background. 2) The browser interface, which is the user interface where you write and execute code. It connects to the server.
What happens when you assign a value to a variable in Python?
-When you assign a value to a variable in Python, such as `a = 1`, you are saying that the variable name 'a' now refers to or points to the value 1. The variable acts like a name tag, rather than a box.
Is it possible for two variables to refer to the same value in Python?
-Yes, it is possible for two or more variables in Python to point to or refer to the same value. For example, if `b = 2` and then `d = 2`, both `b` and `d` refer to the value 2.
How do you swap the values between two variables without repeating the actual values?
-You can use a temporary third variable. Set the temp variable to one of the original variables, then set one original variable to the other, then set the other original variable to the temp variable.
What causes a 'NameError' in Python?
-A NameError happens in Python when you try to use a variable that has not been defined yet. For example, if you print(x) but have not set x to anything, it will raise a NameError.
What options are there for Python development environments?
-The main options are using an Integrated Development Environment (IDE) like PyCharm or using Jupyter Notebook, which offers quick writing and testing of code.
What is the purpose of the print() function?
-The print() function is used to output text, variables, numbers, etc. to the screen. Anything passed inside the parentheses of print() will be displayed.
How can I best follow along with this Python tutorial series?
-The best way to follow along is to install Python and Jupyter Notebook on your own computer by downloading Anaconda. Then you can write and execute the example code shown in the videos.
Outlines
π¨βπ« Introduction to Python for Beginners
YK, the founder of CS Dojo and a former Google software developer, introduces a new Python tutorial series aimed at absolute beginners or those with some programming experience seeking to learn Python. This first video covers the basics of Python, including its applications, the simplicity of its syntax, and its popularity in both academic and professional settings. YK outlines the series' approach, emphasizing the use of Jupyter Notebook for code execution and Anaconda for easy installation of Python and Jupyter. The video promises a comprehensive beginner's guide to Python, from installation to executing basic print functions and understanding variables.
π₯οΈ Setting Up Python and Jupyter Notebook
This segment walks through the process of installing Python and Jupyter Notebook via Anaconda, emphasizing the choice of Python 3 over Python 2 for this course. YK provides step-by-step instructions for downloading and installing Anaconda, launching Jupyter Notebook, and creating a first Python program. The focus is on setting up a development environment that is beginner-friendly and conducive to learning Python programming. The use of Jupyter Notebook is highlighted for its ease of use and relevance to both the scientific community and data analysis.
π Basic Programming Concepts in Python
YK explains fundamental programming concepts using Python, starting with variables. Through examples, viewers learn how to assign values to variables and the importance of variable names in referring to data types like integers and strings. The concept of a variable as a name tag rather than a box is introduced, underscoring Python's flexibility in variable assignment. This section lays the foundation for understanding how variables work in Python, including the reassignment of variables and the distinction between variables referring to the same value.
π Understanding Variable Assignment and Errors
The tutorial continues with more advanced examples of variable assignment, including reassigning variables to different data types and understanding name errors when referencing undefined variables. YK demonstrates the process of variable reassignment, highlighting Python's dynamic type system that allows variables to refer to different types of data throughout a program's execution. This part of the tutorial aims to deepen the understanding of variables, addressing common errors and how to troubleshoot them.
π Swapping Variable Values in Python
In this final segment, YK tackles a common programming task: swapping the values of two variables without directly reassigning them. He presents a problem-solving approach that avoids repeating code and adapts to changes in variable content. The tutorial explores solutions using temporary variables, demonstrating a key programming pattern for value swapping. This practical exercise serves to consolidate the viewer's understanding of variables and prepares them for more complex programming challenges.
Mindmap
Keywords
π‘Python
π‘Variable
π‘IDE
π‘Jupyter Notebook
π‘Anaconda
π‘Print Function
π‘Data Type
π‘String
π‘Swapping Variables
π‘Syntax
Highlights
Introduction of the Python Tutorial series for absolute beginners by YK, a former Google software developer and founder of CS Dojo.
Explanation of Python's popularity and its use in various fields such as web development, data analysis, and scientific research.
Guide on installing Python and setting up a development environment using Jupyter Notebook through Anaconda.
Overview of Jupyter Notebook's components: the command line interface server and the browser window user interface.
Introduction to basic Python syntax and the print function.
Explanation of variables in Python and how to assign values to them.
Demonstration of how to swap the contents of two variables.
Clarification on the use of single and double quotes for strings in Python.
Introduction to data types in Python, including strings and numbers.
Explanation of how variables can refer to different types of data, including numbers and strings.
Insight into Python's handling of variables and memory allocation, contrasting with languages like C and C++.
Discussion on the possibility of multiple variables referring to the same value.
Explanation of reassigning variables and the importance of variable names as references to data.
Tutorial on using a temporary variable to swap the values of two other variables.
Encouragement for viewers to practice coding through exercises provided in the video and further resources available online.
Transcripts
Browse More Related Video

π©βπ» Python for Beginners Tutorial
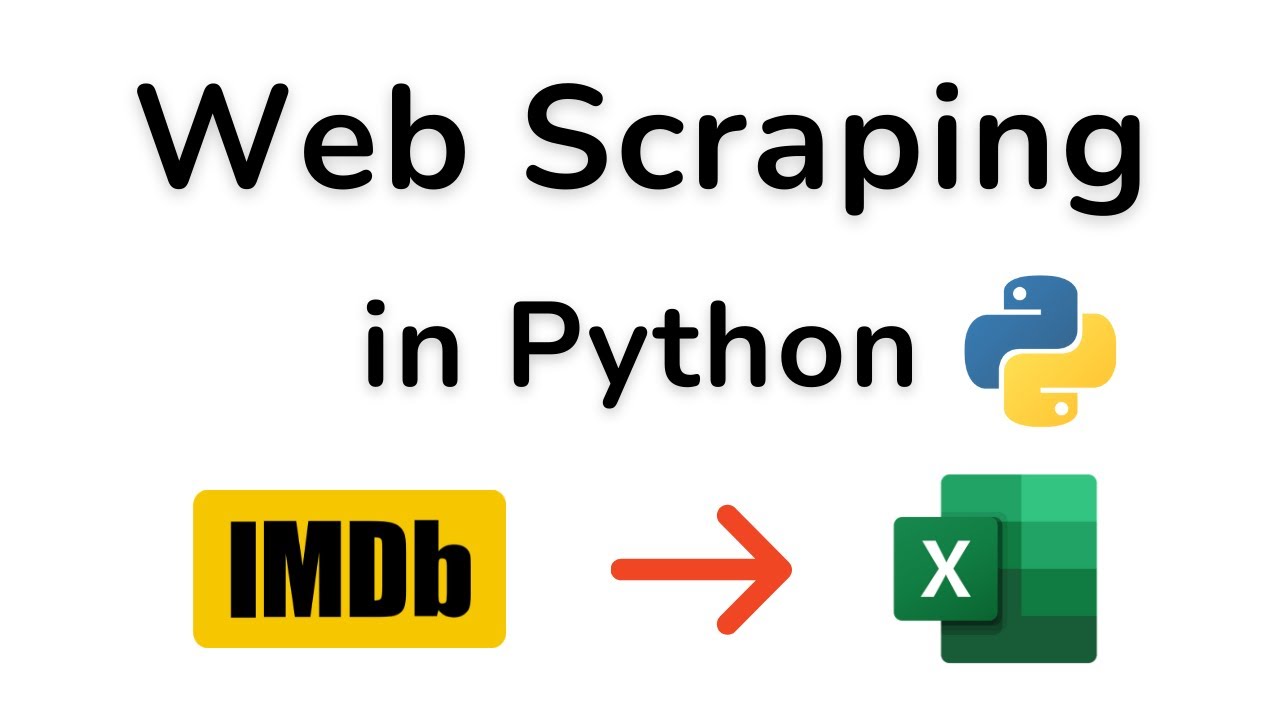
Web Scraping in Python using Beautiful Soup | Writing a Python program to Scrape IMDB website

What Programming Language Should I Learn First?
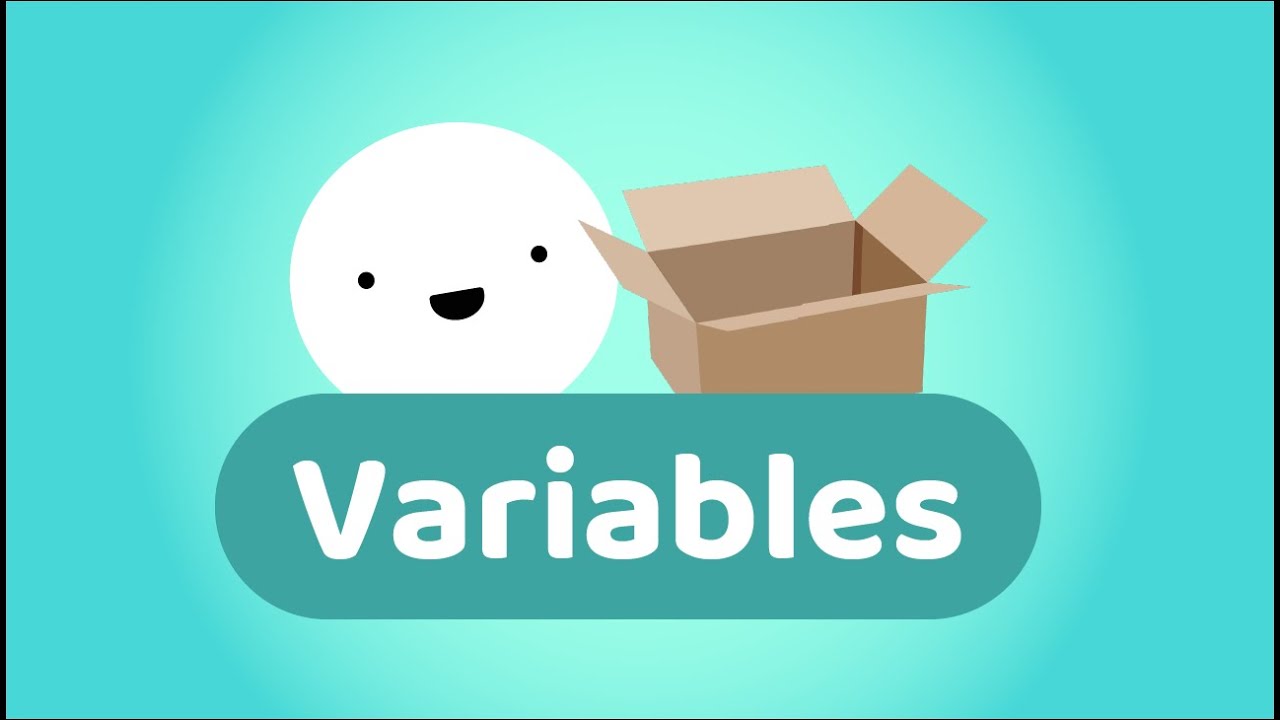
Coding Basics: Variables | Programming for Beginners |
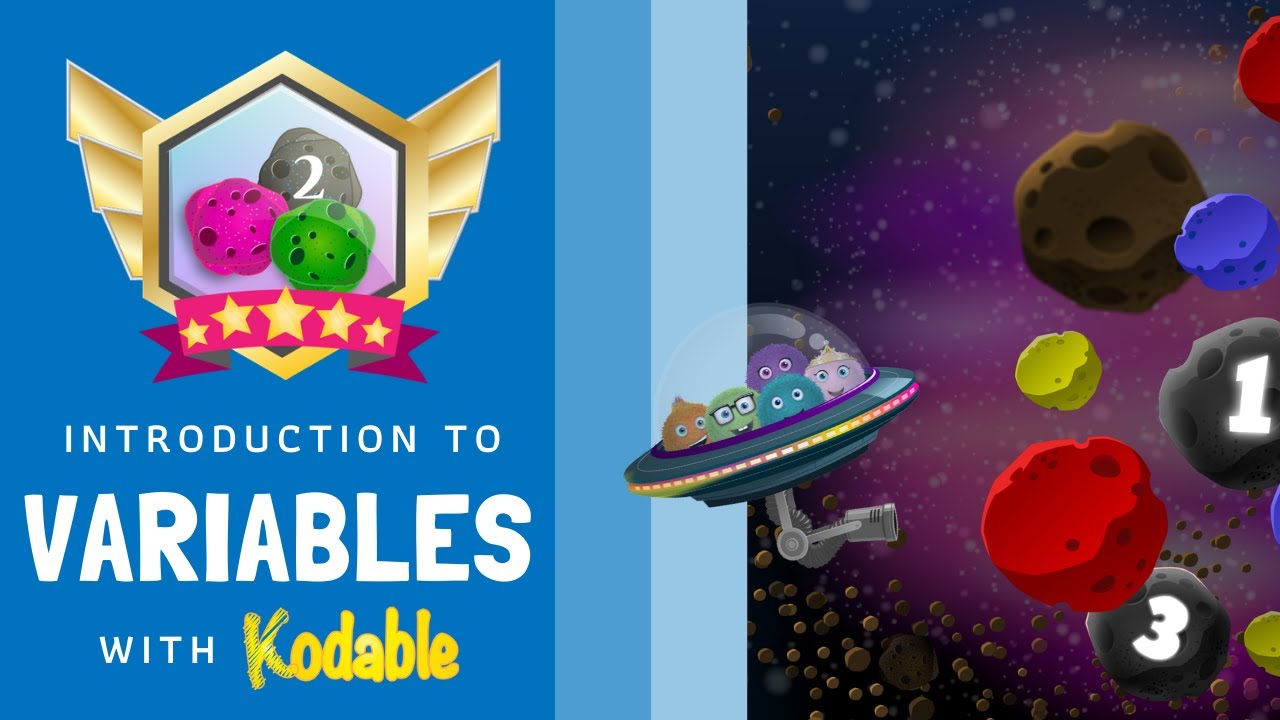
What are Variables? Coding for Kids | Kodable

Introducing the TI 84 Plus CE - Getting Started Series
5.0 / 5 (0 votes)
Thanks for rating: