Y combinator and recursion in haskell (implement fib without recursion)
TLDRIn this video, the speaker explains the Y combinator, a higher-order function that can determine the fixed point of any given function. They illustrate how the Y combinator allows for recursion without explicitly using recursive definitions in Haskell. The video includes a step-by-step demonstration of defining a factorial function using the Y combinator, showing how it can perform recursion indirectly. The speaker emphasizes the power and flexibility of the Y combinator in creating recursive functions, even in languages that do not natively support explicit recursion.
Takeaways
- π§ββοΈ The video discusses the concept of the Y combinator, a higher-order function that can compute a fixed point for any given function.
- π The Y combinator is used to define recursion in languages that do not support it natively, allowing the creation of recursive functions.
- π€ The script poses the question of how the Y combinator can produce a fixed point for any function, given its definition.
- π The script suggests a recursive definition where the Y combinator takes a function 'f' and finds its fixed point.
- π’ The Y combinator has a type signature that allows it to take a function from type 'a' to 'a' and return a fixed point.
- π€¨ The script highlights a paradox where the Y combinator can find a fixed point for functions like the square root, despite the type system's limitations.
- π The script explores the challenge of defining recursive functions like factorial without explicit recursion, using the Y combinator.
- π The factorial function is redefined to avoid direct recursion, instead using the Y combinator to achieve the same result.
- π The script demonstrates that the factorial function can be redefined to take an additional argument 'F', which is used in the recursive step.
- π The Y combinator is shown to be a powerful tool that can enable recursion in languages that do not support it, with a few lines of code.
- π The video concludes by emphasizing the simplicity and power of the Y combinator, which can implement any recursive function with a small amount of code.
Q & A
What is the Y combinator?
-The Y combinator is a function in functional programming that allows the creation of recursive functions without explicit recursion. It takes any function and returns its fixed point, effectively enabling recursion through function composition.
What is a fixed point in the context of the Y combinator?
-A fixed point, in the context of the Y combinator, refers to a function that, when applied to itself, returns the same function. The Y combinator finds this fixed point for any given function.
How does the Y combinator enable recursion?
-The Y combinator enables recursion by taking a function and applying it to itself in a way that simulates the behavior of a recursive function. It achieves this through a recursive definition that does not rely on explicit recursion.
What is the type signature of the Y function?
-The type signature of the Y function typically takes a function from a type A to A and returns a function of the same type. This is denoted as \( \lambda a.((a \rightarrow a) \rightarrow a) \rightarrow a \).
Why is the Y combinator considered magical or strange?
-The Y combinator is considered magical or strange because it can produce a fixed point for any given function, even though it does not know anything about the function itself. This ability to generate a recursive function from any function is counterintuitive and powerful.
How can the Y combinator be used to define the factorial function?
-The Y combinator can be used to define the factorial function by creating a function that takes itself as an argument and uses pattern matching to handle the base case (factorial of 0) and the recursive case (factorial of n).
What is the problem with defining a recursive function without recursion?
-Defining a recursive function without recursion is problematic because you cannot reference the function itself within its definition. This is typically solved by using the Y combinator, which allows the function to reference itself indirectly.
Why does the factorial function need two arguments when defined with the Y combinator?
-When defining the factorial function with the Y combinator, it needs two arguments: the number for which the factorial is being calculated and the function itself (which is provided by the Y combinator). This is necessary to enable the recursive behavior.
How does the Y combinator enable the creation of recursive functions in a language without explicit recursion?
-In a language without explicit recursion, the Y combinator enables the creation of recursive functions by providing a way for a function to reference itself indirectly. It achieves this by taking a function as an argument and applying it in a way that simulates recursion.
What is the significance of the Y combinator in functional programming?
-The significance of the Y combinator in functional programming is that it allows for the creation of recursive functions in a language that does not support explicit recursion. This is particularly useful in languages like Haskell, where recursion is typically handled through other means.
Outlines
π§ββοΈ Introduction to Y Combinator and Fixed Points
The speaker begins by delving into the concept of the Y Combinator, a function in untyped lambda calculus that can compute the fixed point of any function. They explain that the Y Combinator takes a function 'f' and returns its fixed point, which is a value that remains unchanged when 'f' is applied to it. The speaker also touches on the idea of defining functions recursively and how the Y Combinator can be used to achieve recursion without explicit recursive definitions in the language.
π Exploring Recursion without Recursion
In this paragraph, the speaker discusses the challenge of defining recursive functions like factorial without the use of recursion. They illustrate this by attempting to define the factorial function using the Y Combinator, which requires a different approach due to the lack of self-reference in the original definition. The speaker shows how to redefine the factorial function to work with an additional argument 'F', which is then used to simulate recursion through pattern matching and function application.
π’ Implementing Factorial Using Y Combinator
The speaker concludes by demonstrating how the factorial function can be implemented using the Y Combinator. They explain that by providing the Y Combinator with a function 'F' that represents the factorial operation, the function can be applied to itself to achieve the recursive behavior. The speaker shows that with just a few characters and the Y Combinator, complex recursive functions can be implemented without explicit recursion, highlighting the power and flexibility of this approach.
Mindmap
Keywords
π‘Magic
π‘Fixed Point
π‘Combinator
π‘Y Combinator
π‘Recursive Definition
π‘Type Signature
π‘Factorial Function
π‘Multi-line Mode
π‘Pattern Matching
π‘Type Constraints
π‘Equality Type Constraint
Highlights
Introduction to the Y combinator and its ability to find the fixed point of any function.
Explanation of how the Y combinator can be defined by itself without relying on recursion.
Discussion on the type signature of the Y function and its ability to handle any types.
An intuitive challenge presented by the Y combinator's capability to find the fixed point of the square root function.
The problem of defining recursive functions like factorial without explicit recursion in a language like HCAL.
A creative workaround to define factorial using the Y combinator in a language without recursion.
The importance of the Y combinator in enabling recursion in languages that do not support it natively.
A detailed example of redefining the factorial function to work with the Y combinator.
The revelation that the Y combinator requires two arguments for the factorial function to work.
The successful compilation of the redefined factorial function using the Y combinator.
The type signature of the Y combinator and its implications for using it with the factorial function.
The demonstration of the factorial function working as expected when applied with the Y combinator.
The philosophical and practical implications of using simple functions like the Y combinator to achieve complex recursive behaviors.
The comparison between the traditional recursive approach and the Y combinator method for defining functions.
The efficiency of using the Y combinator, requiring only a few characters to define complex recursive functions.
The overall impact of the Y combinator on programming languages and the ability to create recursive functions without explicit recursion support.
Transcripts
Browse More Related Video

Essentials: Functional Programming's Y Combinator - Computerphile

Factorial with lambda calculus
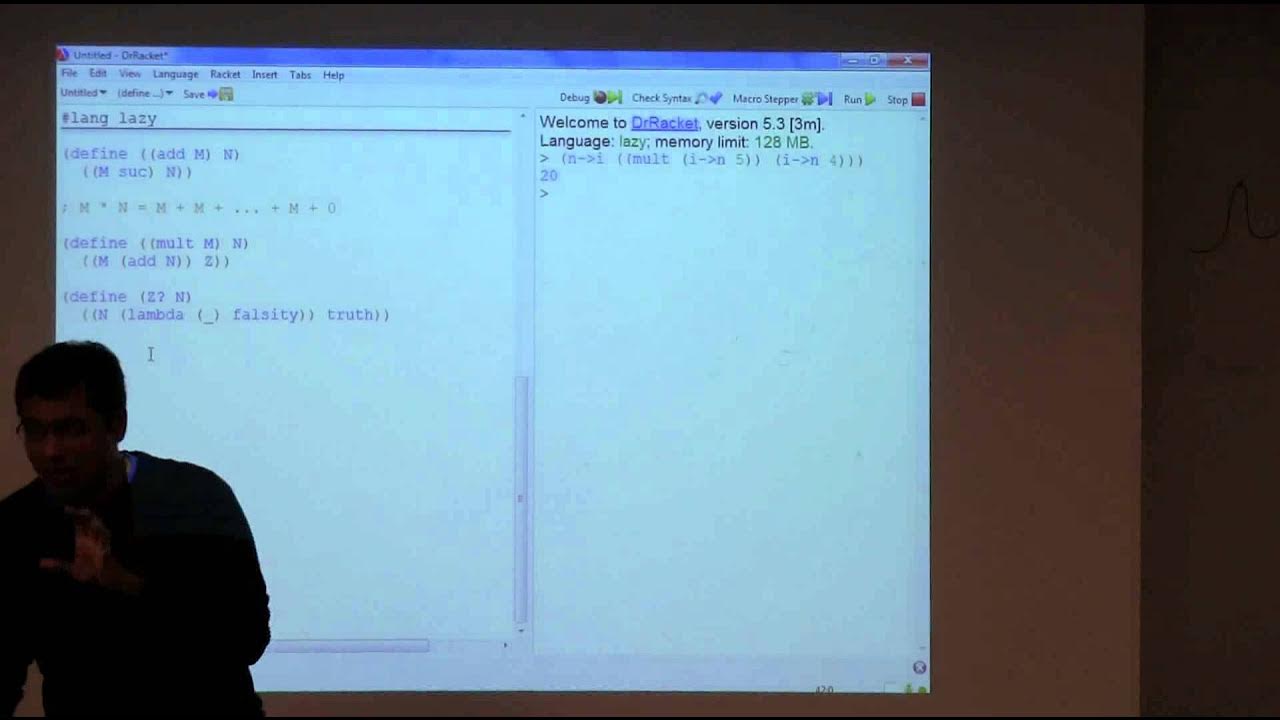
Programming Languages - The Lambda Lecture
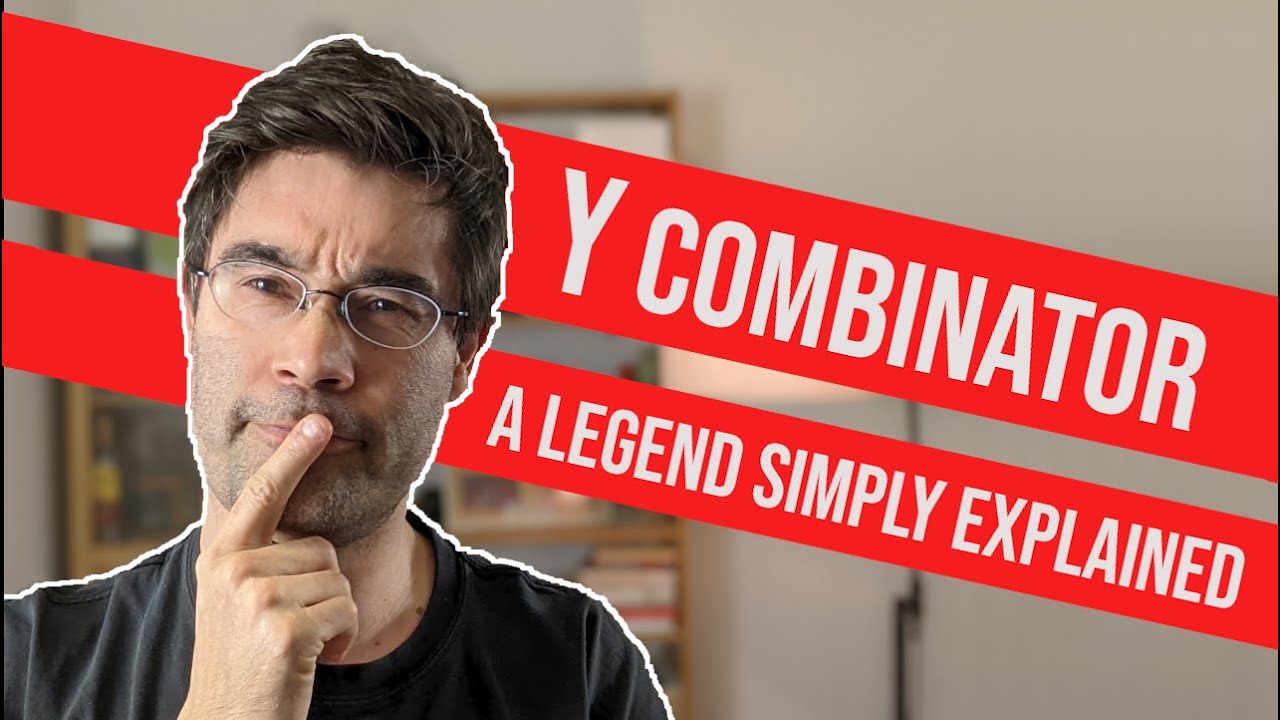
The most intriguing discovery of Computer Science: the Y combinator demystified.
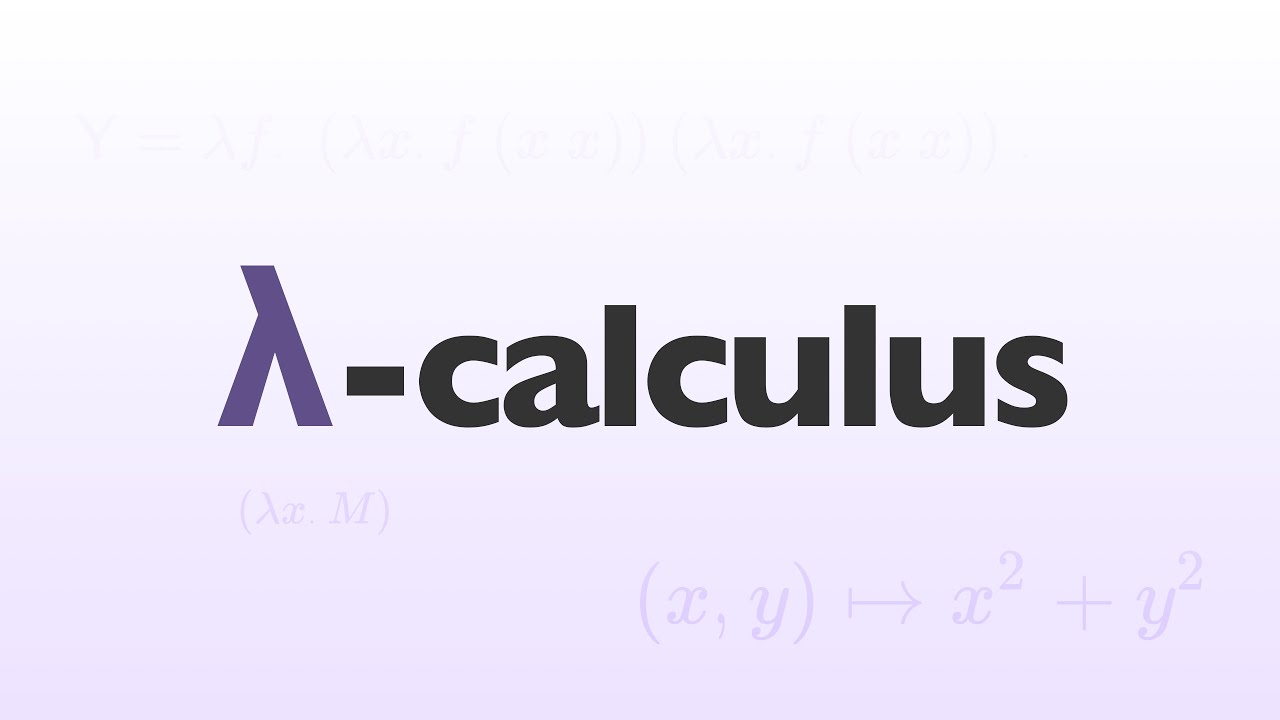
Lambda Calculus: The foundation of functional programming, and the simplest programming language

Introduction to Combinatory Logic βΒ #SoME2
5.0 / 5 (0 votes)
Thanks for rating: