Essentials: Functional Programming's Y Combinator - Computerphile
TLDRThis video script delves into the Y combinator, a fascinating and counterintuitive concept in computer science that enables recursion in languages devoid of looping mechanisms. It begins with a refresher on recursion, using the factorial function as an example, and then transitions into the Lambda Calculus, a minimalistic language for defining functions. The script explores how to encode recursion within this framework, introducing the Y combinator as a method to achieve self-application of functions. The video also discusses the broader implications of the Y combinator, including its metaphorical application to a startup incubator and its historical roots with mathematician Haskell Curry.
Takeaways
- π The Y combinator is a concept in computer science that allows for recursion in languages that do not inherently support it.
- π€ Recursion is defined as the process of defining something in terms of itself, as seen in the factorial function example.
- π The factorial function is a classic example of recursion, where the factorial of a number n is n multiplied by the factorial of n-1.
- π§ The Lambda Calculus is a minimal language for defining functions using only variables, lambda notation, and function application.
- π The top comment on a previous video prompted the discussion on the Y combinator, highlighting its significance in the field.
- π The Y combinator is based on the concept of self-application, where a function applies itself to its input, creating a loop.
- π The simplest recursive definition in Lambda Calculus is a program that loops indefinitely, such as 'loop = loop'.
- π€Ή The Y combinator can be used to encode any recursive function, making it a powerful tool in Lambda Calculus.
- π‘ The Y combinator was invented by Haskell Curry, a mathematician, and gives its name to the Haskell programming language.
- π’ The Y combinator is also the name of a startup incubator company, symbolizing the idea of programs running other programs, akin to their role in helping startups.
Q & A
What is the Y combinator?
-The Y combinator is a way of doing recursion in a language which doesn't have any recursion or looping mechanism. It is one of the most interesting and weirdest ideas in computer science.
What is recursion?
-Recursion is the idea of defining things in terms of themselves. It involves a function calling itself in order to solve a problem by breaking it down into smaller instances of the same problem.
Can you provide an example of a recursive function?
-A factorial function is a classic example of a recursive function. It calculates the product of an integer and all the integers below it, with the factorial of 1 being defined as 1.
What is the Lambda Calculus?
-The Lambda Calculus is a minimal language for defining functions. It consists of variables, lambda notation for building functions, and a way of applying functions. It encodes all other features in terms of these three basic elements.
How are logical values True and False represented in the Lambda Calculus?
-In the Lambda Calculus, True is represented as a function that takes two arguments and returns the first one, while False is represented as a function that takes two arguments and returns the second one.
What is the simplest recursive definition in the Lambda Calculus?
-The simplest recursive definition in the Lambda Calculus is a program that loops indefinitely, such as 'loop equals loop'. This can be encoded using self-application, where a function is applied to itself.
What is the general form of recursion in computer science?
-The general form of recursion in computer science is represented by a function that applies another function to itself infinitely, such as 'rec(f) = f(rec(f))'. This is known as general recursion.
How can the Y combinator be used to encode recursion in the Lambda Calculus?
-The Y combinator is a function that encodes recursion by applying a function to itself in a way that mimics the behavior of a recursive function. It is defined in a self-referential manner, similar to the loop definition in the Lambda Calculus.
Who invented the Y combinator?
-The Y combinator was invented by Haskell Curry, a mathematician from the United States, who also gave his name to the Haskell programming language.
What is the significance of the Y combinator in the context of the company Y Combinator?
-The company Y Combinator chose its name as a metaphor for what they do. Just as the Y combinator in computer science is a way of having programs run other programs, Y Combinator is a startup incubator that helps launch other companies.
Outlines
π§ Introduction to the Y Combinator and Recursion
This paragraph introduces the concept of the Y combinator in the context of computer science, specifically within the Lambda Calculus. It discusses the importance of recursion, a fundamental concept where functions are defined in terms of themselves, exemplified by the factorial function. The factorial function is explained as a countdown and multiplication process that results in the product of all whole numbers up to a given number. The paragraph sets the stage for a deeper exploration of the Y combinator, which enables recursion in languages that do not inherently support it, and invites viewers to revisit the basics of recursion and Lambda Calculus.
π The Concept of Self-Application and Recursive Encoding
The second paragraph delves into the encoding of recursion within the Lambda Calculus, a system that lacks built-in support for looping or recursion. It introduces the idea of self-application, where a function applies to itself, as a method to achieve recursion. The paragraph provides a simple example of a 'loop' that perpetually refers to itself, illustrating the concept of infinite recursion. It then extends this idea to a more general form of recursion with the 'rec' function, which applies a given function to itself repeatedly. The 'rec' function is highlighted as a means to encode any recursive function within the Lambda Calculus. The paragraph concludes with exercises for the viewer to practice encoding a loop and the factorial function using the 'rec' function, thereby deepening their understanding of recursion in the Lambda Calculus.
π― The Y Combinator: Encoding General Recursion
The final paragraph presents the Y combinator, a key element for encoding general recursion in the Lambda Calculus. It explains that the Y combinator, despite not being recursive itself, enables the encoding of recursive functions by utilizing the concept of self-application previously introduced. The paragraph provides the formal definition of the Y combinator and draws a parallel between its structure and the simpler 'loop' example. It emphasizes the Y combinator's significance as a tool for achieving recursion in languages without native support for it. The origin of the Y combinator is attributed to Haskell Curry, after whom the Haskell programming language is named. The paragraph concludes with a mention of the Y Combinator company, a startup incubator, which chose its name as a metaphor for its role in nurturing new companies, mirroring the self-application concept of the Y combinator in programming.
Mindmap
Keywords
π‘Y combinator
π‘Recursion
π‘Lambda Calculus
π‘Factorial function
π‘Self application
π‘Base case
π‘True and False
π‘Function application
π‘General recursion
π‘Haskell Curry
π‘Start-up incubator
Highlights
The Y combinator is one of the most interesting and one of the weirdest ideas in Computer Science.
The Y combinator allows for recursion in a language without any looping mechanism.
Recursion is defined as the process of defining things in terms of themselves, exemplified by the factorial function.
The factorial function is naturally defined recursively, multiplying a number by the factorial of its predecessor.
Lambda Calculus is a minimal language for defining functions with only variables, lambda notation, and function application.
Logical values True and False are encoded in Lambda Calculus as functions that choose between two options.
The concept of self-application, applying a function to itself, is key to implementing recursion in Lambda Calculus.
A simple recursive program in Lambda Calculus can be represented as a loop that spins on the spot without doing anything.
The general form of recursion in Computer Science is represented by the function 'rec', applying a function infinitely.
The Y combinator is a method to encode recursion in Lambda Calculus, despite it not having native support for recursion.
The Y combinator is structurally similar to the loop definition, utilizing self-application with a function in between.
The Y combinator was invented by Haskell Curry, after whom the Haskell programming language is named.
The Y combinator is not only a theoretical concept but also has practical applications, such as in the startup incubator company named Y Combinator.
The company Y Combinator uses the metaphor of the Y combinator to represent their role in helping to start other companies.
Alonzo Church, who invented the Lambda Calculus, was interested in the notion of a function and its foundational role in mathematics and computer science.
Exercises are provided to the audience to understand how to implement loop and factorial functions using the Y combinator in Lambda Calculus.
The Y combinator demonstrates the power of expressing complex computational patterns using simple Lambda Calculus constructs.
Transcripts
Browse More Related Video

Y combinator and recursion in haskell (implement fib without recursion)
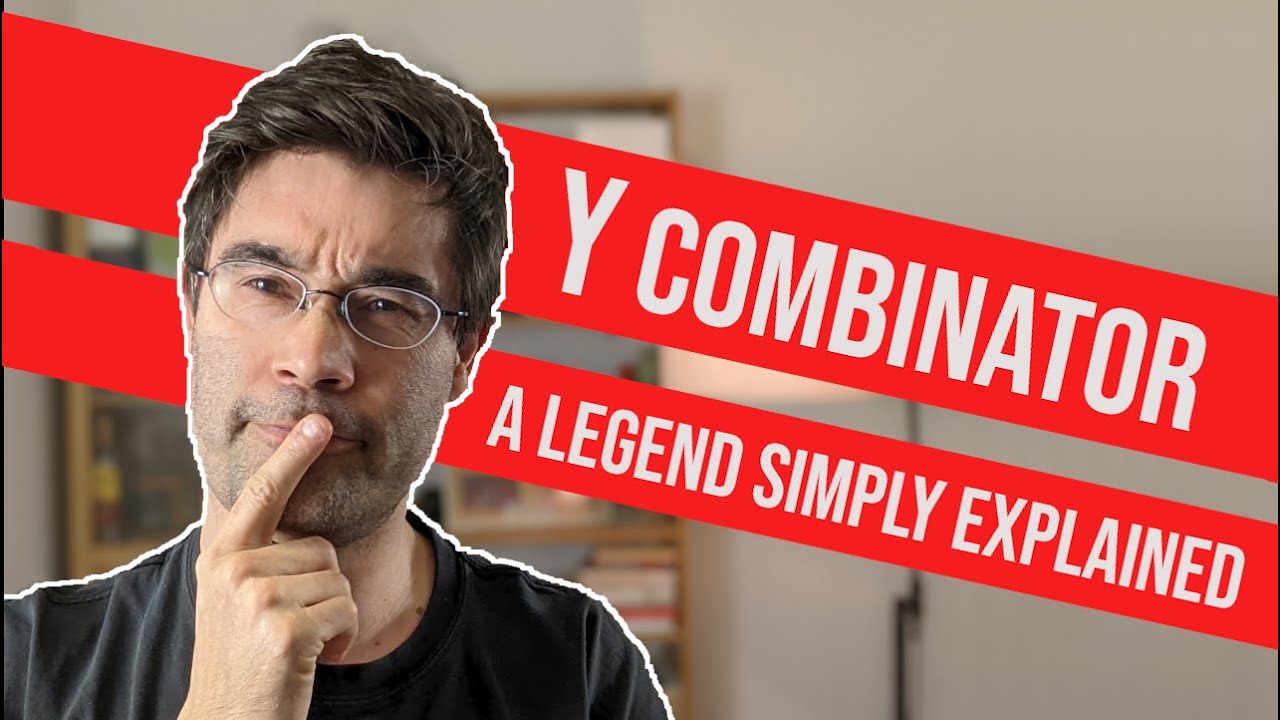
The most intriguing discovery of Computer Science: the Y combinator demystified.
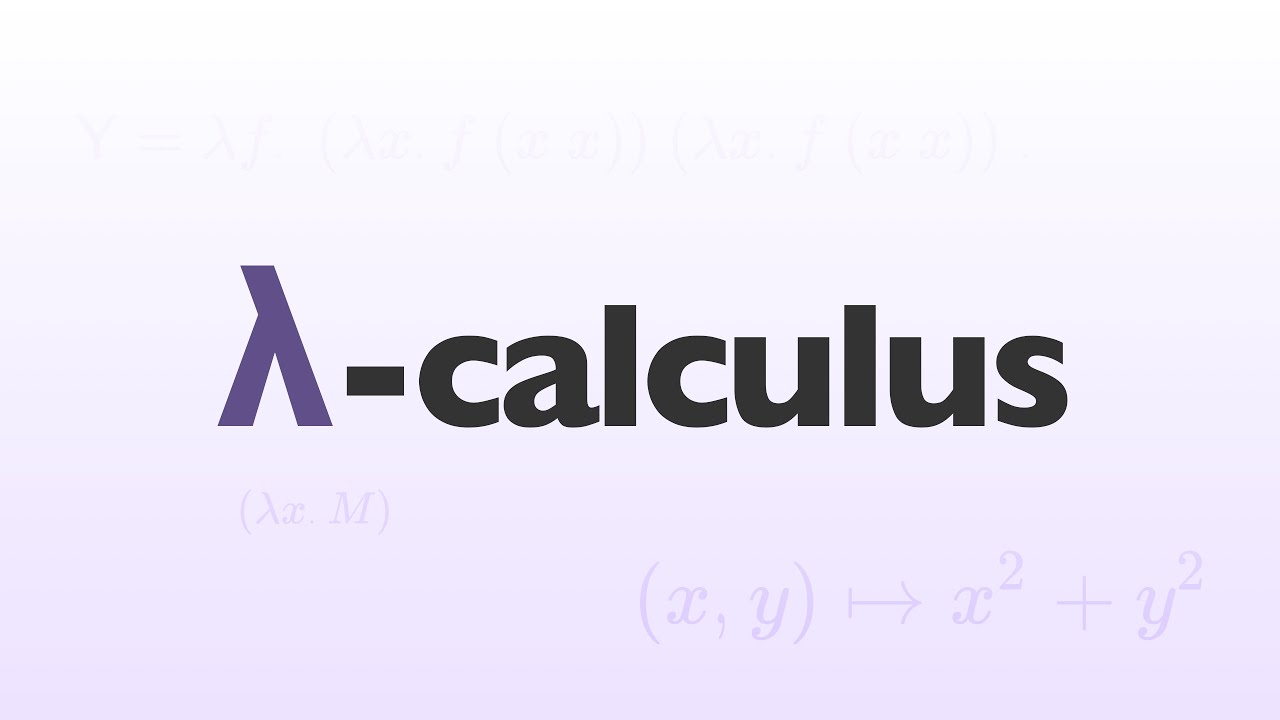
Lambda Calculus: The foundation of functional programming, and the simplest programming language

Factorial with lambda calculus
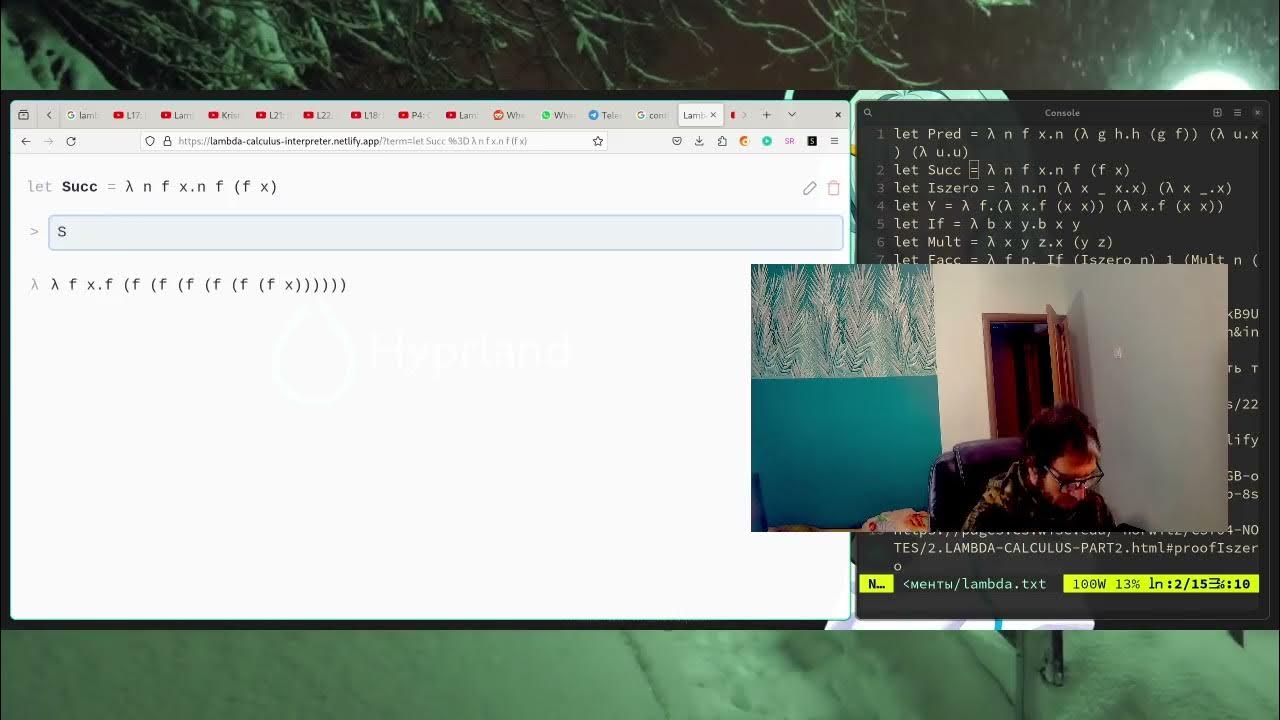
Lambda Calculus Calculator
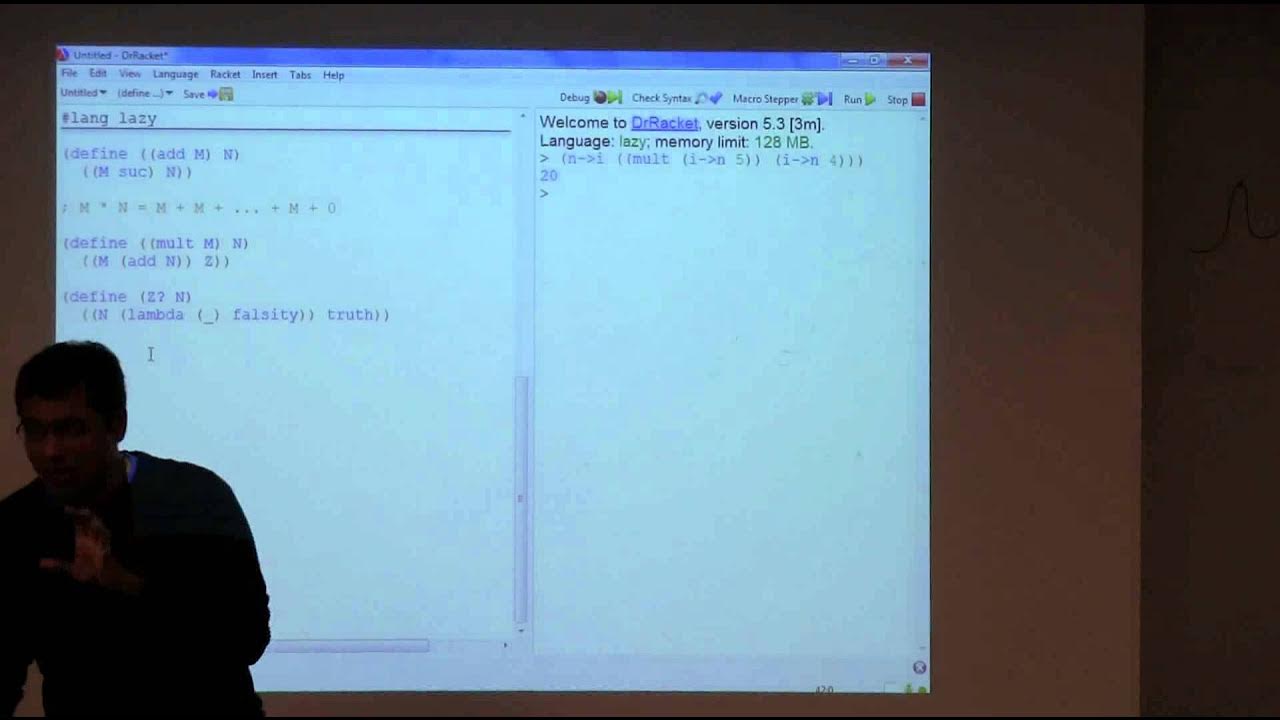
Programming Languages - The Lambda Lecture
5.0 / 5 (0 votes)
Thanks for rating: