Essentials: Pointer Power! - Computerphile
TLDRIn this educational video script, Professor Brailsford and Steve Bagley explore the concept of pointers in computer science, using LEGO to illustrate linked lists. They explain the structure of a 'THING' in C language, including pointers to strings and the next 'THING'. The script covers the basics of pointer manipulation, special cases in list insertion, and the importance of checking for NULL pointers. It also hints at a 'top-secret trick' involving double pointers, promising a deeper dive in a future video.
Takeaways
- π The script discusses the fundamental concept of pointers in computer science, using the example of linked lists to illustrate their use.
- π§© The lecturer introduces a LEGO model to visually represent linked lists, aiming to make the concept more accessible for beginners.
- π The script includes a brief introduction to C programming, specifically how to declare and manipulate structures using pointers.
- π The 'typedef' keyword is used to simplify the declaration of a structure, allowing the shorthand 'THING' to be used instead of the full struct definition.
- π The 'item' and 'next' members within the 'THING' structure represent a pointer to a string and a pointer to the next structure in the list, respectively.
- π Recursion is subtly introduced as the 'next' member is a pointer to the same type of structure, allowing for the creation of linked lists.
- π― The importance of checking for NULL pointers is emphasized to avoid segmentation faults in programming.
- π The script touches on special cases in list manipulation, such as inserting at the head or end of a list and the need for careful pointer management.
- π The concept of a 'roving' pointer is introduced for traversing the list to find the correct position for inserting new elements.
- π The script suggests that understanding and correctly implementing pointer manipulation is crucial for successful linked list operations.
- π§ The lecturer hints at a 'top-secret trick' involving pointers to pointers, suggesting it will be covered in a future video to simplify list operations.
Q & A
What is the main concept discussed by Professor Brailsford in the video?
-The main concept discussed by Professor Brailsford is the idea of a pointer in computer science, particularly its use in linked lists.
How does Professor Brailsford plan to illustrate the concept of linked lists?
-Professor Brailsford plans to illustrate the concept of linked lists using a LEGO model that he and Sean have developed.
What does Professor Brailsford use to represent a 'THING' in the LEGO model?
-In the LEGO model, a 'THING' is represented by a grey base plate with a red box on top, which holds a pointer to a string of characters.
What is the significance of the 'item' and 'next' in the context of the C programming language?
-In the context of the C programming language, 'item' and 'next' are members of a structure, where 'item' is a pointer to a string of characters and 'next' is a pointer to the next 'THING' in the linked list.
Why does Professor Brailsford use a 'typedef' declaration in the C code?
-Professor Brailsford uses a 'typedef' declaration to define a shorthand for the structure of type 'THING', allowing him to use 'THING' as a type instead of the full structure definition.
What does the term 'char' stand for in the C programming language?
-In the C programming language, 'char' stands for character, and it is used to define a pointer to a single character or a string of characters.
How does Professor Brailsford handle the insertion of a new 'THING' into the linked list?
-Professor Brailsford handles the insertion by using a roving pointer to traverse the list, find the correct alphabetical position, and then manipulate the pointers to insert the new 'THING'.
What is the importance of checking for NULL when traversing a linked list?
-Checking for NULL is important to prevent the program from following a NULL pointer, which would result in a segmentation violation or crash.
Why does Professor Brailsford emphasize not using the 'start' pointer as a roving pointer?
-He emphasizes this because moving the 'start' pointer away from the head of the list could result in losing reference to the list, making it impossible to find again.
What special cases does Professor Brailsford mention regarding the insertion into a linked list?
-Professor Brailsford mentions special cases such as inserting at the end of the list where NULL is present, and inserting at the head of the list, which requires updating the 'start' pointer.
What concept does Professor Brailsford introduce at the end of the script to make list manipulation easier?
-Professor Brailsford introduces the concept of a pointer to a pointer to a 'THING' (a THING **), which can simplify the process of list manipulation by allowing for more flexible pointer operations.
Outlines
π Introduction to Pointers and Linked Lists
In this segment, Professor Brailsford introduces the concept of pointers as a fundamental construct in computer science, emphasizing their utility in linked lists. He uses a LEGO model to visually explain how pointers link together elements in a list. The discussion revolves around the idea of a 'THING', represented by a LEGO baseplate, which contains a pointer to a string (item) and a pointer to the next 'THING' (next). The professor also introduces the C language's `typedef` to simplify the structure definition and explains the importance of pointers in managing data in a linked list.
π Understanding Linked List Structure and Memory Allocation
This paragraph delves deeper into the structure of linked lists, highlighting the need for memory allocation to store strings within the 'item' field of each 'THING'. Professor Brailsford discusses the challenges of managing memory for variable-length strings and the importance of pointers in linking these strings to their respective 'THING' structures. He also touches on the practical aspects of coding linked lists in C, including the use of NULL pointers to indicate the end of a list and the potential for errors in pointer manipulation.
π Traversing and Inserting in a Linked List
The focus of this paragraph is on the process of traversing a linked list to find the correct position for inserting a new 'THING'. Professor Brailsford explains the use of a roving pointer to navigate through the list and determine the alphabetical order of items. He illustrates the careful manipulation of pointers required to insert a new item, such as 'burgers', between existing items like 'beer' and 'chips'. The importance of checking for NULL pointers to avoid segmentation faults is also emphasized.
π Special Cases in Linked List Management
In this final paragraph, Professor Brailsford addresses special cases in linked list management, such as inserting items at the head or end of the list. He discusses the complexities involved in updating the 'start' pointer when a new item becomes the head of the list, and the need for careful handling of NULL pointers when inserting at the end. The professor also hints at a future discussion on a 'top-secret trick' involving pointers to pointers, which could simplify the process of managing linked lists.
Mindmap
Keywords
π‘Pointer
π‘Linked List
π‘LEGO Model
π‘C Language
π‘typedef
π‘Struct
π‘NULL
π‘Recursion
π‘Barbecue Items
π‘Traversal
π‘Insertion
π‘Segmentation Violation
π‘Type Theory
π‘Cliffhanger
Highlights
Introduction to the concept of pointers in computer science, particularly in the context of linked lists.
Use of LEGO to illustrate the concept of linked lists, making it accessible for beginners.
Explanation of pointers as 'firemen's hoses' or 'connectors' in LEGO models.
Discussion on the components of a 'THING' in the context of a linked list, including a grey baseplate, a red box, and a blue box.
Introduction to the C language structure and the use of `typedef` for shorthand notation.
Explanation of `char *item` as a pointer to a string of characters in C.
Recursion in the definition of a linked list, where a `struct _thing` refers back to itself.
Discussion on the importance of the `next` member in a linked list and its role in connecting elements.
Illustration of how to declare and initialize a singly-linked list in C, including handling of NULL pointers.
Introduction of a roving pointer `p` for traversing the linked list and finding insertion points.
Explanation of how to insert a new element into a linked list while maintaining alphabetical order.
Highlight of the need for careful pointer manipulation to avoid segmentation faults and maintain list integrity.
Discussion on special cases in linked list insertion, such as inserting at the head or the end of the list.
Introduction of the concept of a pointer to a pointer to a THING (`THING **`) and its potential benefits.
Emphasis on the importance of type safety in C and the distinction between different levels of pointers.
Teaser for a future video that will reveal a top-secret trick to simplify linked list operations using pointers to pointers.
Transcripts
Browse More Related Video

the brightest laser pointer in the world!
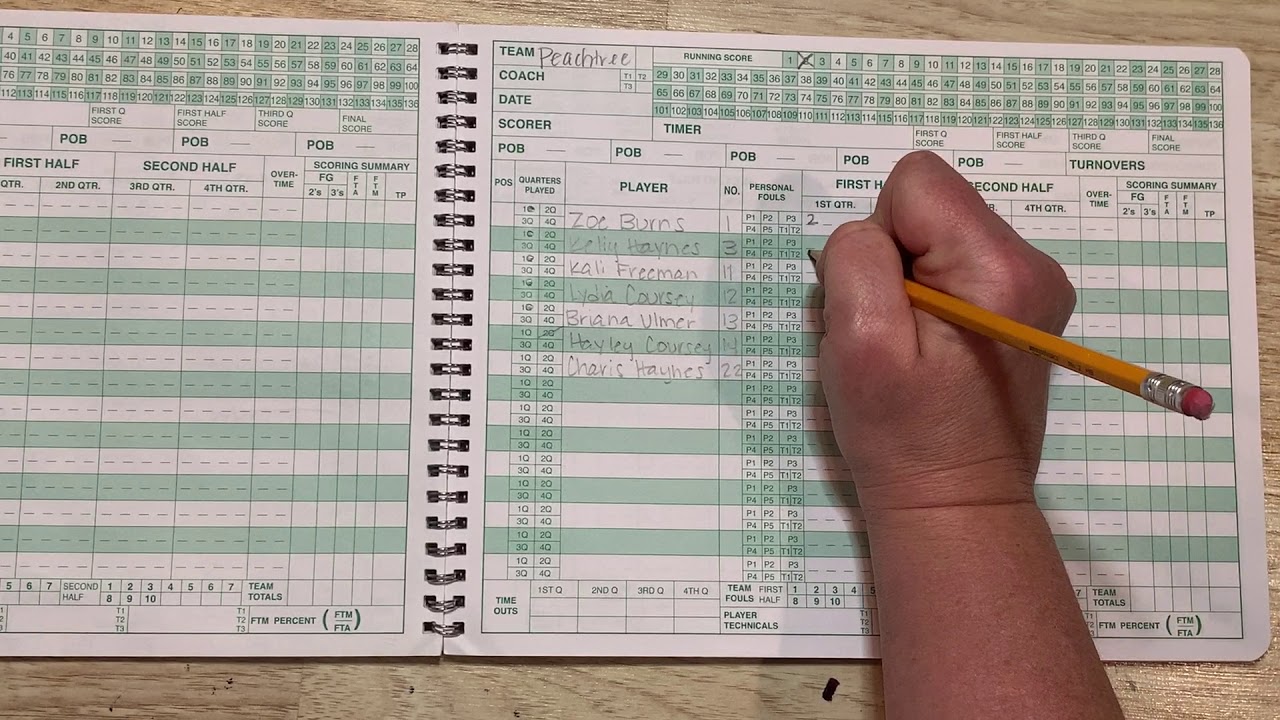
Keeping a Basketball Scorebook
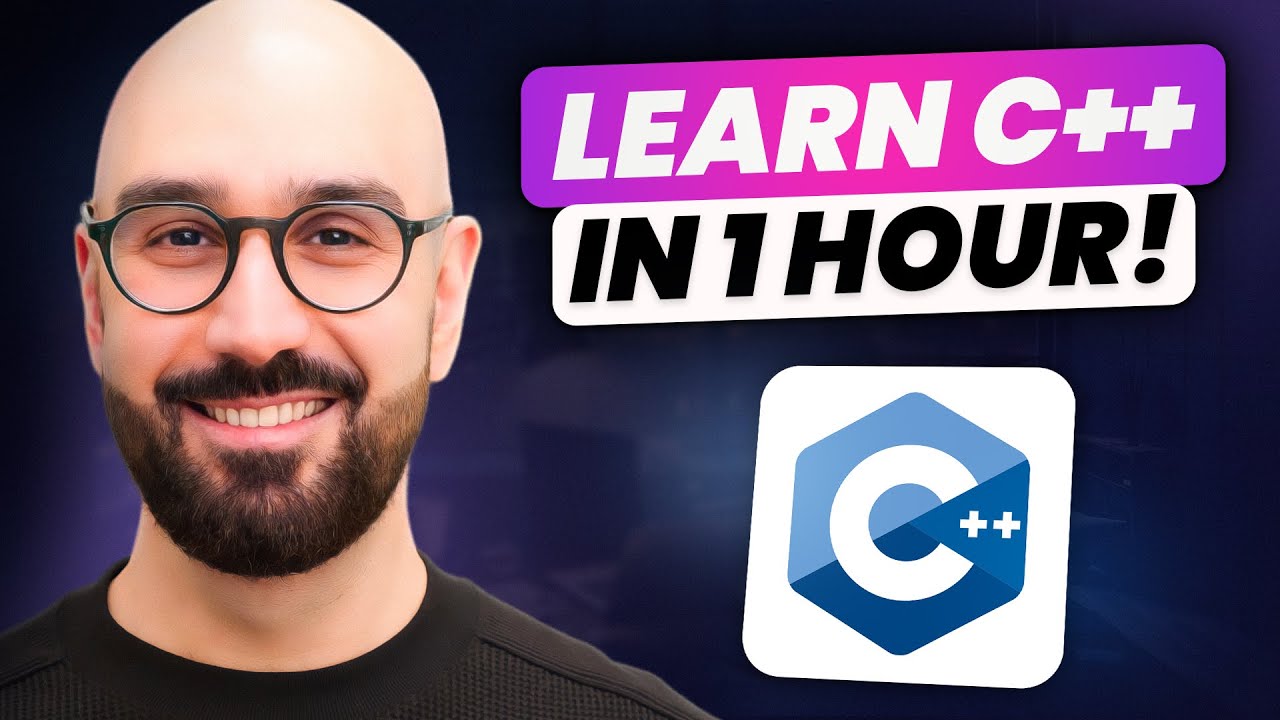
C++ Tutorial for Beginners - Learn C++ in 1 Hour

How NBA shooting has evolved | Let's talk about practice!
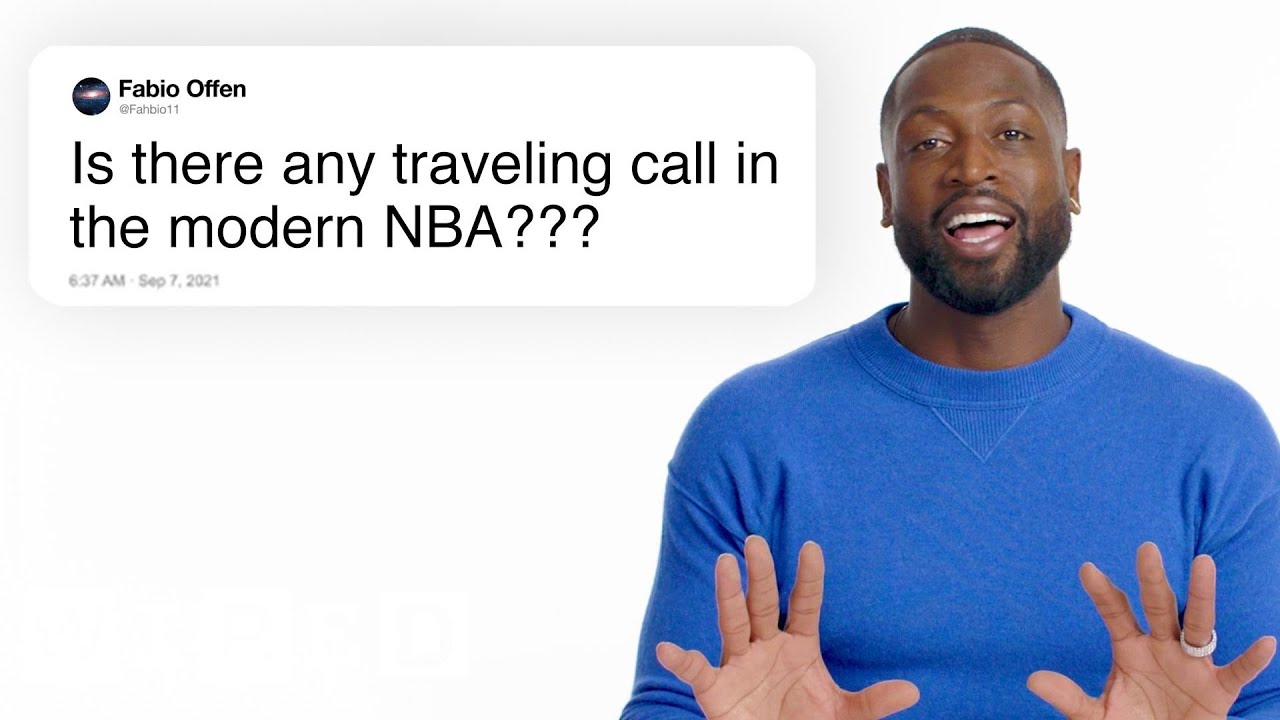
Dwyane Wade Answers Basketball Questions From Twitter | Tech Support | WIRED
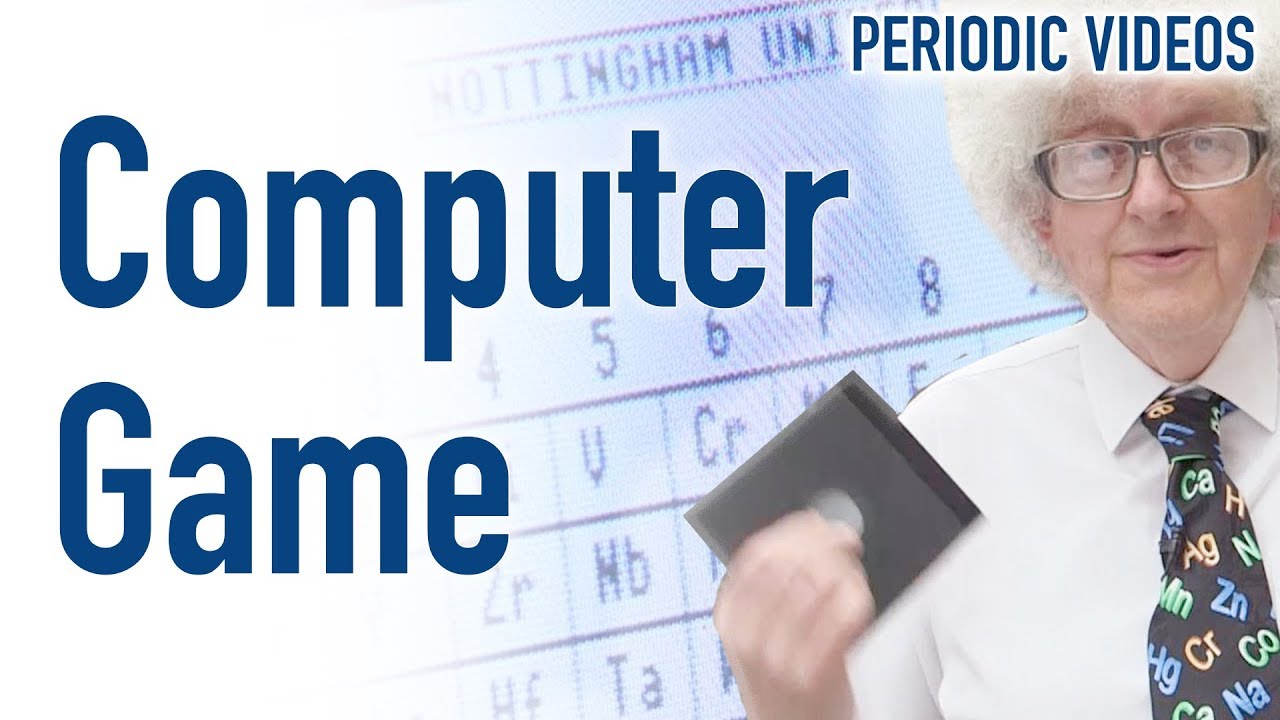
Vintage Chemistry Computer Game - Periodic Table of Videos
5.0 / 5 (0 votes)
Thanks for rating: