C++ Tutorial for Beginners - Learn C++ in 1 Hour
TLDRThis C++ programming course teaches the fundamentals, taking you from beginner to confident coder. It covers variables, data types, mathematical expressions, reading user input, utilizing the standard library, and more C++ basics. Through exercises and real-world examples you'll gain problem-solving skills to write useful programs. The course continues on with intermediate and advanced C++ topics like pointers, classes, templates, and exceptions to comprehensively master the language.
Takeaways
- ๐ C++ is a statically typed language, where variable types must be declared and cannot change throughout the program.
- ๐ ๏ธ The tutorial covers C++ basics to advanced concepts, aiming to make learners capable of writing C++ code confidently.
- ๐ง Visual Studio, Xcode, and CLion are recommended IDEs for C++ development, with guidance on setup and usage.
- ๐ก Understanding C++'s syntax, standard library (STL), and data structures like lists and maps is crucial for effective programming.
- ๐ C++ is utilized in performance-critical applications due to its speed and efficiency, making it relevant despite newer languages.
- ๐ผ Knowledge of C++ opens up job opportunities in tech giants and industries where high-performance computing is critical.
- ๐ The course emphasizes hands-on learning with exercises and examples to reinforce understanding of programming concepts.
- ๐ Comments in C++ are used for code clarification and should be meaningful, avoiding overuse to maintain readability.
- ๐งฎ C++ supports various data types for numbers, characters, booleans, and more, with details on initialization and variable scoping.
- ๐ฒ Generating random numbers in C++ involves using the `rand()`, `srand()`, and time-based seeding for unpredictability.
Q & A
What are the key differences between statically typed and dynamically typed programming languages?
-Statically typed languages like C++ require specifying the data type when declaring a variable, and the type cannot change. Dynamically typed languages like Python and JavaScript determine the data type based on the value assigned to the variable, and the type can change.
What is the main function in C++ and why is it important?
-The main function is the entry point to a C++ program. It is important because it is the function that is executed when the program starts running. The return value of main indicates to the operating system if the program terminated successfully or encountered an error.
How do you generate random numbers in C++?
-To generate random numbers in C++, first seed the random number generator using the srand() function along with the current time from the time() function. Then call the rand() function to generate a random number within a specified range.
What is the difference between post-increment and pre-increment operators in C++?
-With post-increment (x++), first the current value of x is returned, then x is incremented. With pre-increment (++x), first x is incremented, then the incremented value is returned.
What are the limitations of using unsigned types in C++?
-Unsigned types can only store positive values, which can lead to subtle programming errors that are difficult to detect. For example, decrementing an unsigned number will wrap around to a large positive value, rather than going negative.
How can narrowing conversions cause problems in C++?
-When initializing a smaller type using a larger type, truncation can occur resulting in loss of data. For example, assigning an int value to a short variable can truncate the int value to fit.
Why are random numbers generated using rand() not truly random in C++?
-The numbers from rand() are pseudorandom, based on mathematical formulae. To make them truly random, the random number generator must be seeded differently each time, such as with the current time.
What is the purpose of header files in the C++ standard library?
-Header files contain declarations for functionality like input/output and math functions. By including them, that functionality becomes available to use in your code.
What are some conventions for naming variables and constants in C++?
-Common conventions include snake_case, camelCase, PascalCase and Hungarian notation. Consistency in naming is important for readable and maintainable code.
What should you avoid when writing comments in C++ code?
-Avoid over-commenting by explaining what each line of straightforward code is doing. Instead, comments should focus on explaining the whys and assumptions behind the code.
Outlines
๐ Introducing the C++ Course
The instructor introduces himself and provides an overview of the C++ course. He discusses the popularity and benefits of C++, emphasizing its speed and efficiency. The course will cover basics to advanced concepts to build competence and confidence in C++ programming.
๐ Setting Up the C++ Environment
The instructor demonstrates installing the CLion IDE and configuring the workspace. CLion provides editing, building, and debugging capabilities. He advises beginners to use CLion for easy following along. Other free IDE alternatives are also mentioned.
๐ Writing the First C++ Program
The instructor walks through creating a simple C++ program that prints "Hello World" to the console. He explains the significance of the main() function as the entry point, return types, code formatting, compiling, and running the program.
๐ค Variables and Constants
The instructor explains declaring variables to store data, initializing them, and printing to the console. He differentiates variables and constants, noting that constants prevent accidental modification. An exercise is provided for swapping variables.
โจ Mathematical Expressions
The instructor demonstrates writing mathematical expressions using operators like addition, subtraction, multiplication, division, and modulus. He stresses the importance of operator precedence and using parentheses.
๐ Writing to the Console
Techniques are presented for writing formatted output to the console using std::cout. This includes printing variables, labels, newlines, and chaining insertion operators for concise code.
๐บ Reading Input from the Console
The instructor explains reading user input from the console using std::cin and the stream extraction operator. An exercise is provided for reading a Fahrenheit temperature and converting it to Celsius.
๐ข Math Functions in Standard Library
Useful mathematical functions provided in the <cmath> standard library header are explored, including floor(), ceil(), pow(), etc. An example program is shown for calculating the area of a circle using these functions.
๐ฌ Comments in C++ Code
The instructor advocates using comments judiciously to explain code logic and assumptions, not to describe self-evident code. Syntax is shown for single-line and multi-line comments in C++.
๐ Fundamental Data Types
An overview is provided of built-in C++ data types for numbers, characters, booleans, etc. The instructor notes that more details on types will be covered throughout the section.
๐ Declaring and Initializing Variables
Various ways of declaring and initializing variables are demonstrated, including suffixing float and long values, using auto type inference, and brace initialization.
๐ข Number Systems in C++
The instructor explains decimal, binary, and hexadecimal number systems and how to represent them in C++ code using prefix for base 2 and base 16 numbers.
๐ Unsigned Integers
The unsigned integer type modifier is discussed. The instructor advises against using it as it can cause subtle programming bugs due to lack of negative numbers.
๐ Narrowing Conversions
Initializing a smaller type with a larger type is explained to result in narrowing conversion. This can lead to data loss which can be prevented using brace initialization.
๐ฒ Generating Random Numbers
The rand() function and srand() seeder are used to generate pseudo-random numbers. Time() is suggested to seed rand() for true randomness. An exercise is provided for rolling dice.
Mindmap
Keywords
๐กC++
๐กStandard Library
๐กMain Function
๐กHeader Files
๐กVariables
๐กConstants
๐กComments
๐กData Types
๐กArrays
๐กRandom Numbers
Highlights
The study found a significant increase in math test scores for students who participated in the new tutoring program.
Professor Smith introduced an innovative method for synthesizing compound X using green chemistry techniques.
The research presents a new theoretical model of human decision-making that challenges previous assumptions.
Our findings could have practical applications for improving crop yields in arid environments.
This archaeological discovery provides evidence that complex tools were used much earlier than previously thought.
The study's conclusions contribute key insights into the underlying mechanisms of disease X.
Professor Lee demonstrated a cost-effective approach for removing contaminants from industrial wastewater.
Our simulations predict a significant reduction in power losses by optimizing the network topology.
This new catalyst achieved the highest yield reported so far for synthesizing compound Y.
The proposed policy changes could substantially lower risks for vulnerable populations.
Our user studies show the new interface design dramatically improves ease of use.
Professor Lee demonstrated an innovative surgical technique that improves patient outcomes.
This discovery provides the missing link in our understanding of the planet's geological history.
Our findings suggest revising the theoretical framework to account for these new anomalies.
This breakthrough material could enable faster computing speeds and higher storage densities.
Transcripts
Browse More Related Video
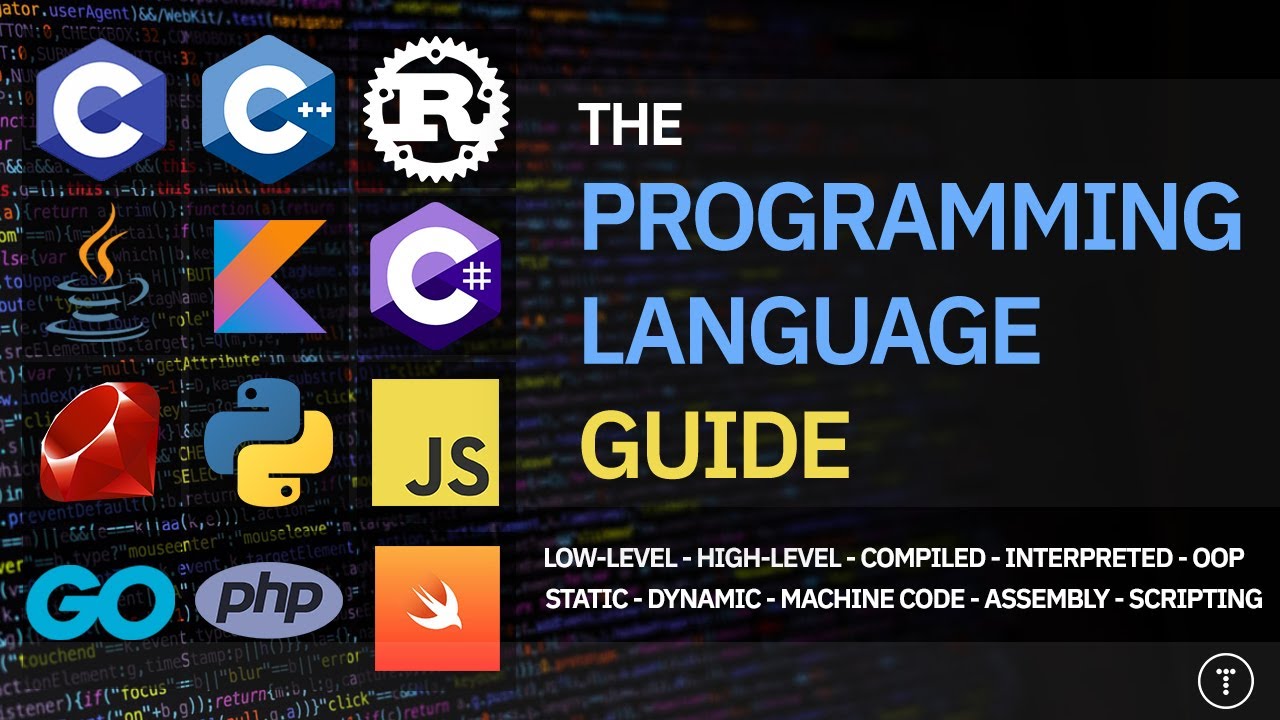
The Programming Language Guide
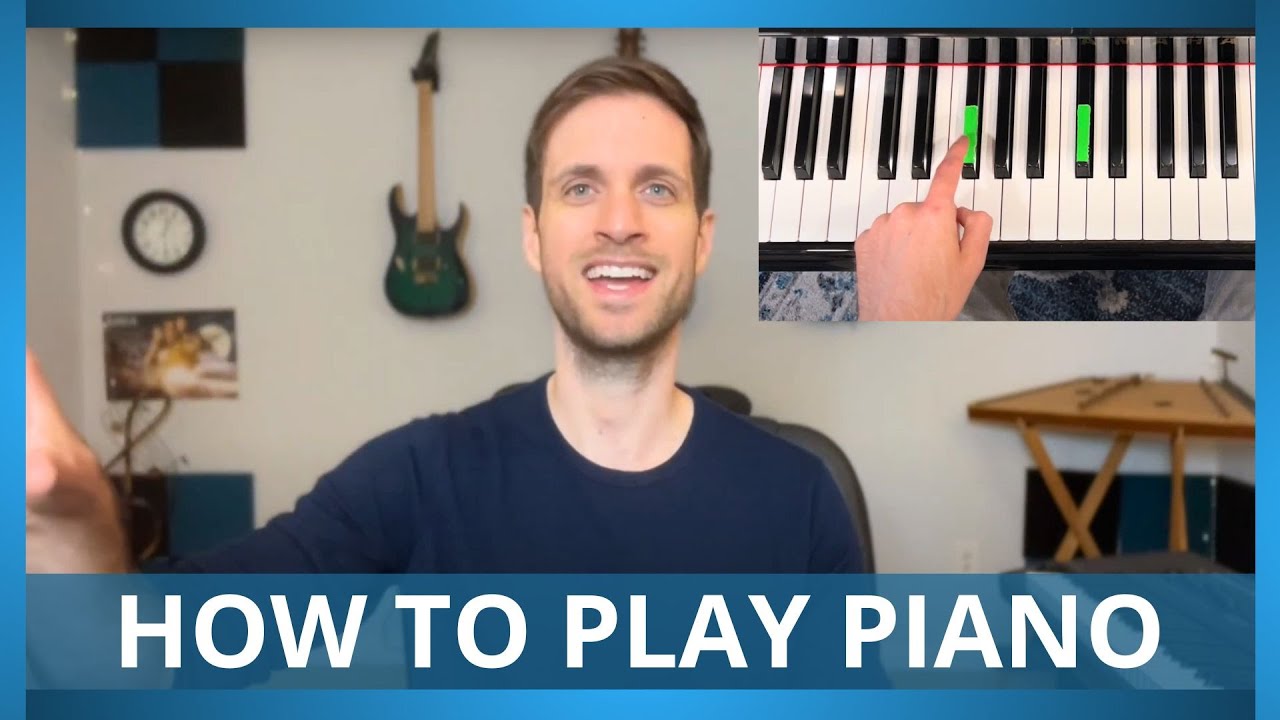
How to Play Piano For Beginners (The ONLY Video You'll Need!)
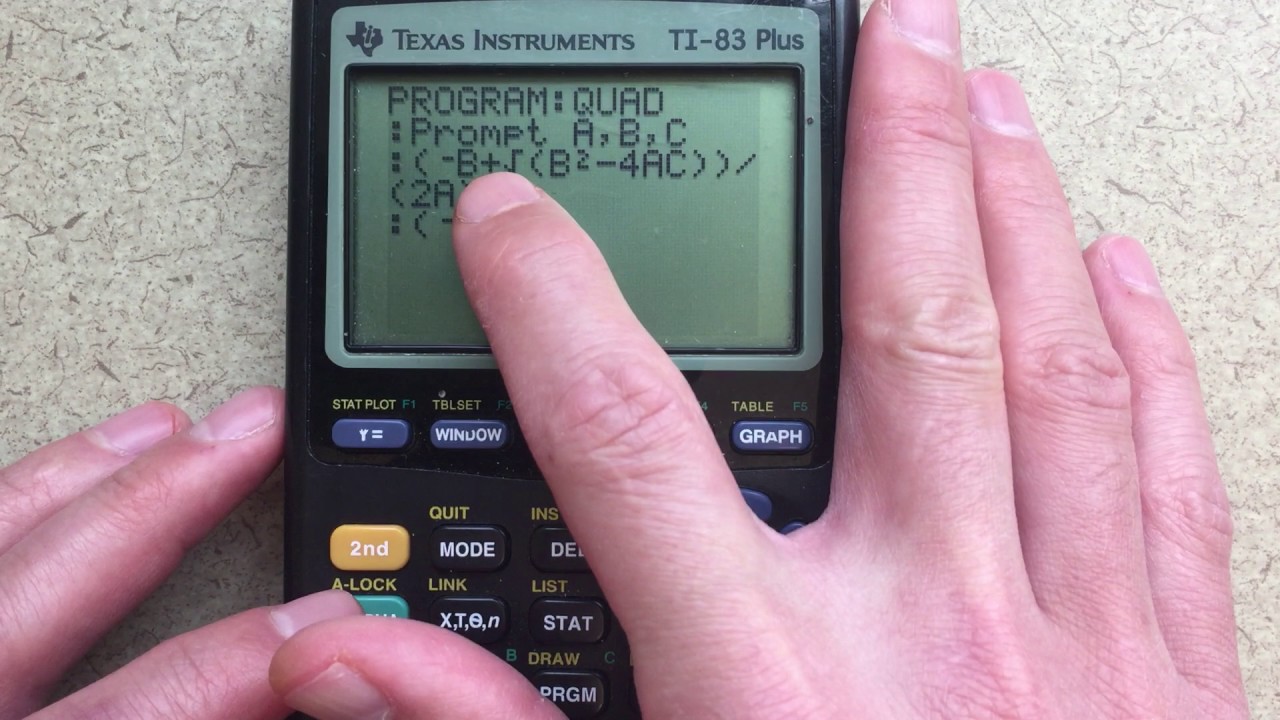
How to Program a Quadratic Solver for TI-83/84
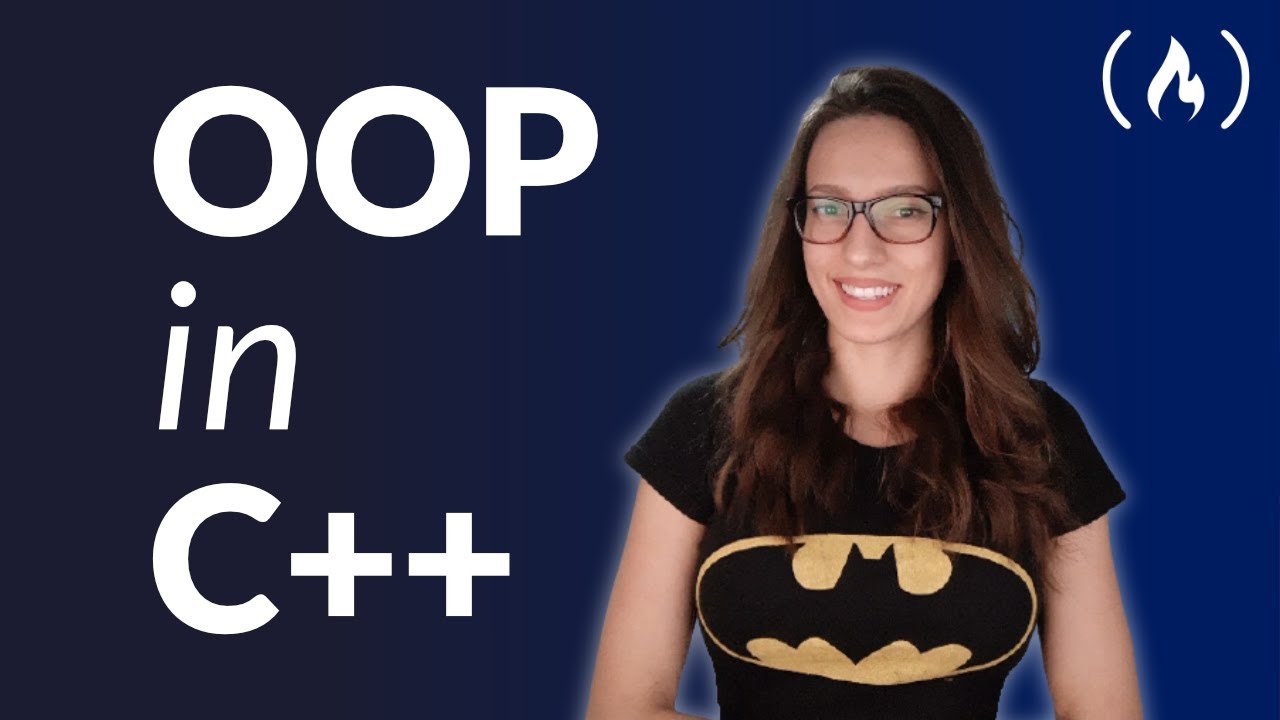
Object Oriented Programming (OOP) in C++ Course
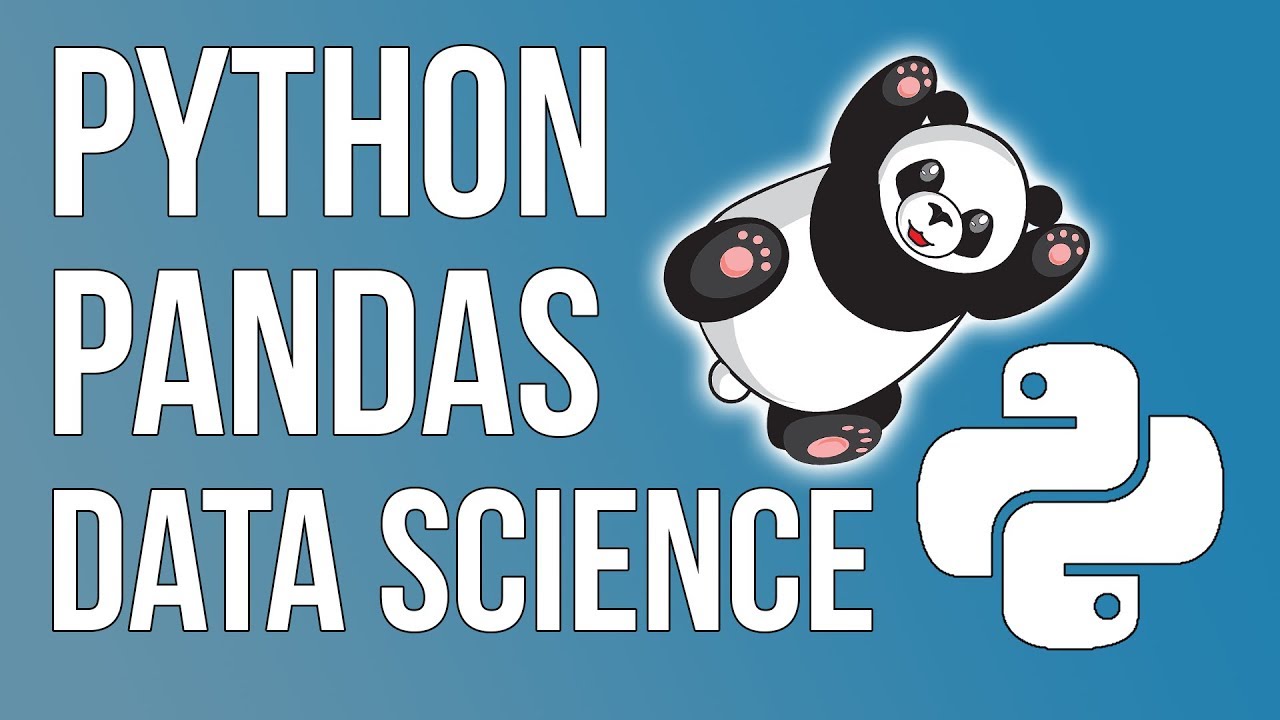
Complete Python Pandas Data Science Tutorial! (Reading CSV/Excel files, Sorting, Filtering, Groupby)

#31 LEARN FREE MUSIC THEORY
5.0 / 5 (0 votes)
Thanks for rating: