A Flock of Functions: Lambda Calculus and Combinatory Logic in JavaScript | Gabriel Lebec @ DevTalks
TLDRThis talk delves into the foundational concepts of lambda calculus and combinatory logic, exploring their role in defining computation and their impact on programming languages. The speaker, a former instructor at Full-Stack Academy and a current Google employee, explains the identity combinator, first-class functions, and higher-order functions. They demonstrate how functions can be arguments and return values, leading into an interactive discussion on lambda notation, function evaluation, and the Church-Turing thesis. The talk also touches on the historical development of these concepts and their practical applications in functional programming, showcasing the elegance and power of functional abstractions.
Takeaways
- 😀 The speaker introduces the concept of 'identity' in lambda calculus, a function that takes an input and returns the same input, highlighting the foundational nature of functions in programming.
- 🎓 The speaker discusses the importance of first-class functions, where functions can be passed as arguments to other functions or returned as values, a key feature of functional programming languages.
- 🔍 The script explains the lambda notation and how it abstracts functions in a concise manner, emphasizing the role of abstraction in programming to handle multiple use cases through parameters.
- 📚 It covers the concept of 'currying', where functions with multiple arguments are transformed into a sequence of functions that each take a single argument, allowing for partial application.
- 🔄 The script introduces the idea of function evaluation in lambda calculus through 'beta reduction', which simplifies expressions by replacing bound variables with their corresponding values.
- 🤔 The Mockingbird combinator is presented as an interesting function that calls its argument on itself, leading to discussions about infinite loops and the limitations of computation models.
- 🔧 The Kestrel (K) combinator is explained as a building block that takes two arguments and returns the first, demonstrating the creation of more complex behaviors from simple functions.
- 🔄 The concept of 'extensional equality' is introduced, where two functions are considered equal if they produce the same output for the same input, regardless of their internal structure.
- 📈 The historical context of lambda calculus and its relation to the work of Alonzo Church and Alan Turing is provided, emphasizing the significance of these theories in the development of computer science.
- 🛠️ The script explores the practical implications of lambda calculus, suggesting that understanding its principles can enhance one's ability to think functionally and work with functional programming languages.
- 🎨 Lastly, the speaker views the study of lambda calculus as an art form, appreciating it for its intellectual beauty and the mental exercise it provides, rather than solely for its practical applications.
Q & A
What is the identity combinator in lambda calculus?
-The identity combinator is a function that takes an input 'a' and returns 'a'. It's a straightforward function that demonstrates the concept of first-class functions in lambda calculus, where functions can be passed as arguments to other functions.
What does the term 'first-class functions' refer to in programming?
-First-class functions refer to the ability of functions to be treated like any other value in a programming language. This means they can be passed as arguments to other functions, returned from functions, and assigned to variables.
What is the significance of the identity function in JavaScript and Haskell?
-In JavaScript and Haskell, the identity function is a utility that simply returns its input value. It's often used as a default function in libraries or as a placeholder for more complex operations, demonstrating how functions can be used in a higher-order manner.
What is lambda notation and how is it used in programming?
-Lambda notation is a way of defining anonymous functions in lambda calculus and functional programming languages. It typically starts with the Greek letter 'lambda', followed by a parameter, a dot, and then an expression that the function returns. It abstracts a function through a parameter, allowing for concise and flexible code.
Can you explain the concept of immutability in lambda calculus?
-In lambda calculus, all variables are immutable, meaning once a variable is assigned a value, it cannot be changed. This is a fundamental aspect of functional programming and helps avoid side effects and make programs easier to reason about.
What is the Mockingbird combinator and what does it do?
-The Mockingbird combinator is a function that takes a value and then calls that value on itself. If the value is a function, it will call the function on itself, which can lead to interesting behaviors like infinite loops or recursive calls.
What is the K combinator and how does it work?
-The K combinator is a function that takes two arguments and always returns the first one, regardless of the second argument. It's a constant function that demonstrates the concept of returning a specific value regardless of input in combinatory logic.
What is the concept of currying in functional programming?
-Currying is the process of transforming a function with multiple arguments into a sequence of functions, each accepting a single argument. This allows for partial application, where a function can be called with some of its arguments first, returning a new function that takes the remaining arguments.
What is the significance of the Church-Turing thesis in computer science?
-The Church-Turing thesis is the idea that any function that can be computed by an algorithm can be computed by a Turing machine, and vice versa. It's a foundational concept in computer science that establishes the limits of computation and the equivalence of different computational models.
What are Church encodings and how are they used in lambda calculus?
-Church encodings are a way of representing data types such as booleans and numbers using functions in lambda calculus. They allow for the manipulation of these data types through function application and abstraction, without the need for specific data type syntax.
What is the role of the S, K, and I combinators in combinatory logic?
-The S, K, and I combinators are fundamental building blocks in combinatory logic. The S combinator can be used to create other combinators, the K combinator returns the first of two arguments, and the I combinator is the identity function. Together, they can be used to construct a wide range of computational behaviors.
How can you represent boolean logic in lambda calculus without specific boolean values?
-Boolean logic can be represented in lambda calculus by using functions that mimic the behavior of boolean operations. For example, the K combinator can represent 'true' by always returning its first argument, and the KI combinator can represent 'false' by always returning its second argument. Operations like 'and', 'or', and 'not' can be defined using these combinators.
What is the purpose of the Y combinator in lambda calculus?
-The Y combinator is used to enable recursion in lambda calculus, which does not have built-in support for named functions or recursion. It allows for the creation of functions that can call themselves, thus enabling recursive computations.
Outlines
😀 Introduction to Lambda Calculus and Combinatory Logic
The speaker introduces the topic of lambda calculus and combinatory logic, explaining the concept of the identity combinator and how functions can serve as arguments to other functions. They clarify that the talk is not affiliated with their employer, Google, and highlight the educational intent of the content. The speaker also mentions the smartly improved version of the talk and provides information on where to find the slides and social media accounts.
🔧 Functions as First-Class Citizens and Lambda Notation
This paragraph delves into the idea that functions in lambda calculus are first-class citizens, meaning they can be passed as arguments and returned from other functions. The speaker illustrates this with JavaScript arrow functions and explains the syntax of lambda calculus, including function application and abstraction. They also discuss the immutability of variables and the concept of abstraction, contrasting it with concrete use cases.
📚 The Grammar of Lambda Calculus and Function Evaluation
The speaker outlines the basic components of the lambda calculus language, which includes variables, function application, abstractions, and the use of parentheses for controlling evaluation order. They explain the process of function evaluation, known as beta reduction, which involves symbol replacement to simplify expressions. The paragraph also touches on the concept of currying, where functions can return other functions.
🕊️ Exploring the Mockingbird Combinator and Infinite Loops
The Mockingbird combinator is introduced, which is a function that calls its argument on itself. The speaker demonstrates the Mockingbird with the identity function and discusses the potential for infinite loops, such as when the Mockingbird is applied to itself. They also mention the concept of Turing completeness and the inherent issues with infinite loops in computational systems.
🎓 Historical Context of Lambda Calculus and Mathematical Foundations
The speaker provides a brief history of lambda calculus, mentioning mathematicians like Alonzo Church, David Hilbert, and Kurt Gödel, and their contributions to the foundations of mathematics. They discuss the quest for a unified axiomatic system and the development of lambda calculus as a simple notation system for symbol replacement that has been used to solve complex mathematical problems.
🔄 The Church-Turing Thesis and Equivalence of Computational Systems
This section discusses the Church-Turing thesis, which posits that lambda calculus and Turing machines define computation in a fundamental way. The speaker explains how both systems are capable of simulating each other, establishing their equivalence in computational power. They also introduce the concept of combinators, which are functions without free variables.
🛠️ Building Abstractions with Combinators and Lambda Calculus
The speaker illustrates how to construct complex functions from simpler ones using combinators like K, I, and C. They demonstrate how these basic building blocks can be combined to create new functions with unique behaviors, such as the Kite combinator, which is formed by combining K and I. The paragraph emphasizes the power of abstraction in lambda calculus.
📈 The Evolution of Programming Languages and Computational Thinking
The speaker discusses the evolution of programming languages from low-level machine code to high-level languages that abstract away machine-specific details. They highlight the shift towards functional programming and languages like Haskell, which are based on lambda calculus, and the benefits of thinking in terms of pure functions rather than mutable state.
🔢 Inventing Numbers and Data Structures with Lambda Calculus
The speaker embarks on creating numbers and data structures from scratch using lambda calculus. They introduce Church encoding, a method of representing data types like booleans and natural numbers as functions. The paragraph demonstrates how to define boolean logic, such as AND, OR, and NOT, using lambda expressions and combinators.
🔄 Recursion in Lambda Calculus and the Power of Functions
The speaker hints at the topic of recursion in lambda calculus, which is a method of defining functions that call themselves. They mention the Y Combinator, a famous combinator that enables recursion in lambda calculus, and encourage the audience to explore this concept further in the second part of the video.
🎉 Conclusion and the Joy of Lambda Calculus
In conclusion, the speaker reflects on the beauty and joy of lambda calculus, not for its practical applications, but as an intellectual pursuit and a form of mental exercise. They emphasize the value of understanding foundational concepts in computer science and the role of lambda calculus in functional programming languages.
Mindmap
Keywords
💡Lambda Calculus
💡Combinatory Logic
💡First-class Functions
💡Identity Function
💡Currying
💡Beta Reduction
💡K Combinator
💡Mockingbird Combinator
💡Church Encodings
💡Turing Completeness
💡I Combinator
Highlights
Introduction to the concept of identity in lambda calculus and its application in programming languages.
Exploration of first-class functions and their role in lambda calculus and combinatory logic.
Demonstration of how functions can be arguments to other functions, showcasing the power of higher-order functions.
Introduction to lambda notation and its equivalence to function definitions in other programming languages.
Explanation of the immutable nature of variables in lambda calculus and its impact on function definitions.
Discussion on the syntax of lambda calculus and its simplicity compared to other programming languages.
Introduction to the Mockingbird combinator and its behavior when applied to itself, leading to infinite loops.
Explanation of the Kestrel combinator and its role in returning the first argument of a function.
Introduction to the concept of currying and its application in lambda calculus.
Discussion on the evaluation process in lambda calculus, focusing on beta reduction and symbol replacement.
Introduction to the Kestrel combinator and its utility in creating constant functions.
Explanation of how combinators can be combined to create new functions with different behaviors.
Introduction to the history of lambda calculus and its role in the foundations of mathematics.
Discussion on the Church-Turing thesis and its implications for the nature of computation.
Introduction to the concept of Church encodings and their application in representing boolean logic.
Explanation of how lambda calculus can be used to invent numbers and perform arithmetic operations.
Introduction to the SNK combinators and their role in covering all possible computations in lambda calculus.
Discussion on the philosophical and practical implications of lambda calculus in programming and computer science.
Transcripts
Browse More Related Video

Lambda Calculus Then and Now
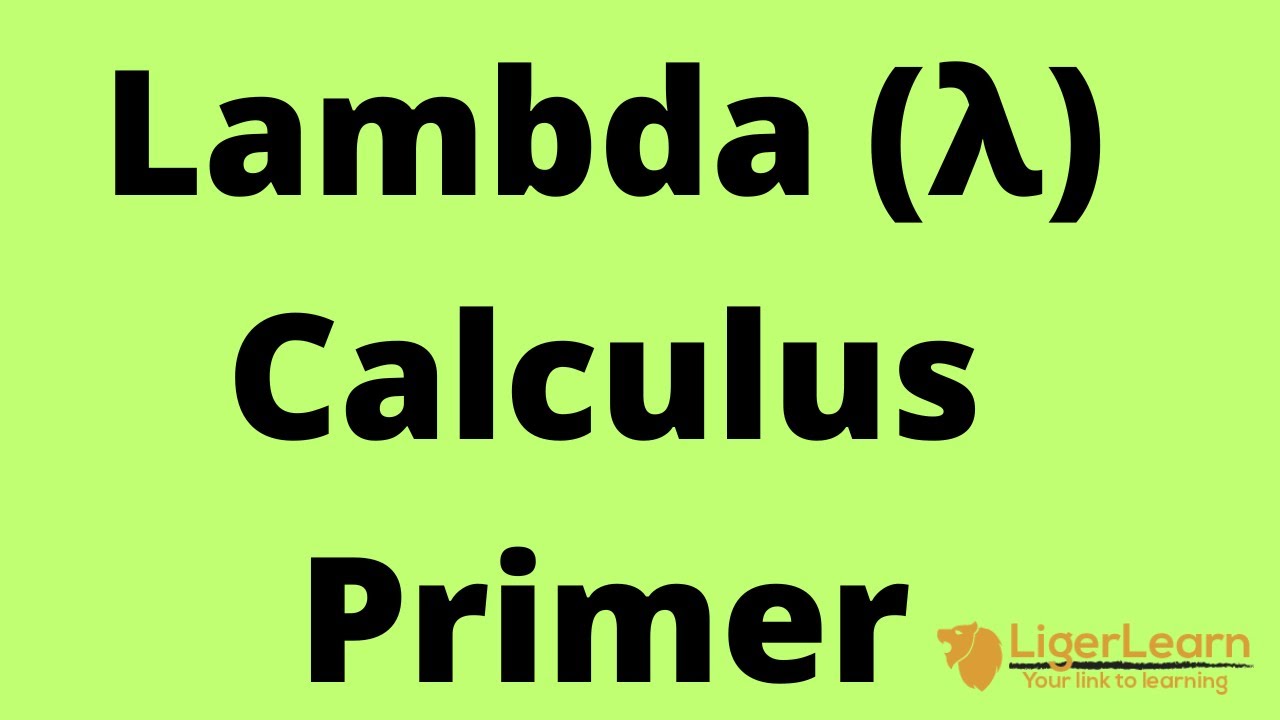
Lambda (λ) Calculus Primer
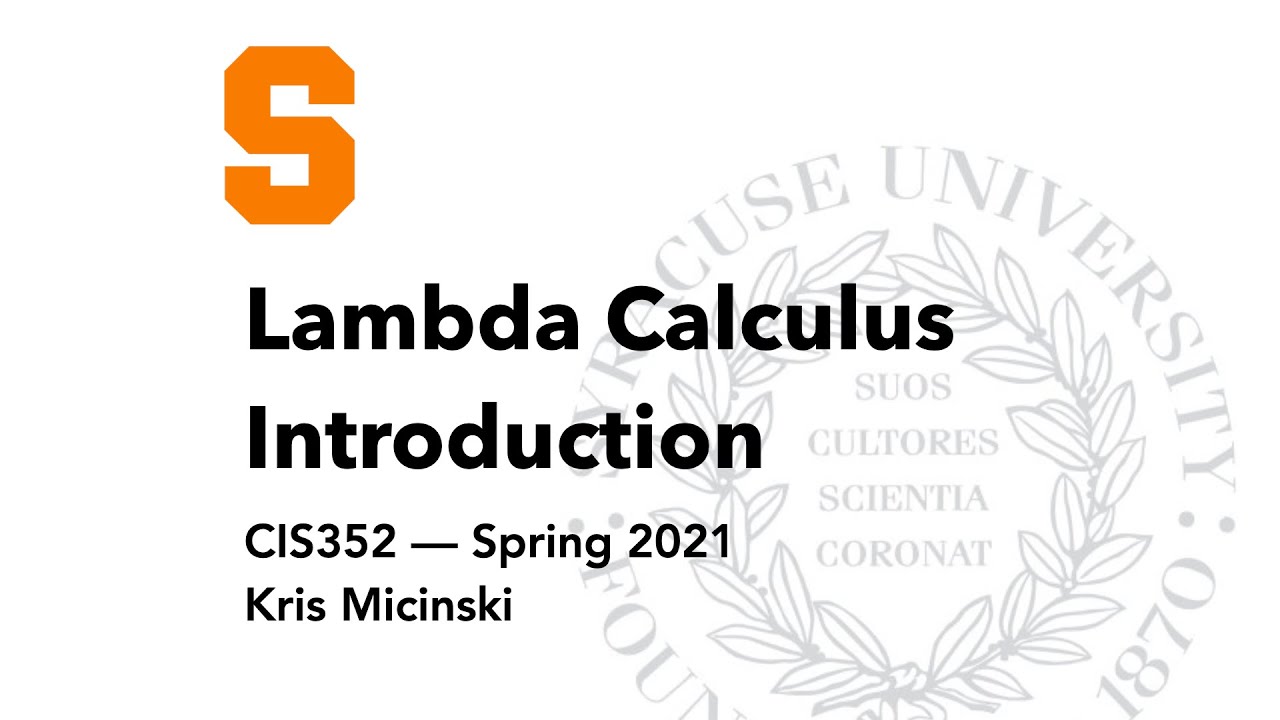
L16: Lambda Calculus Introduction
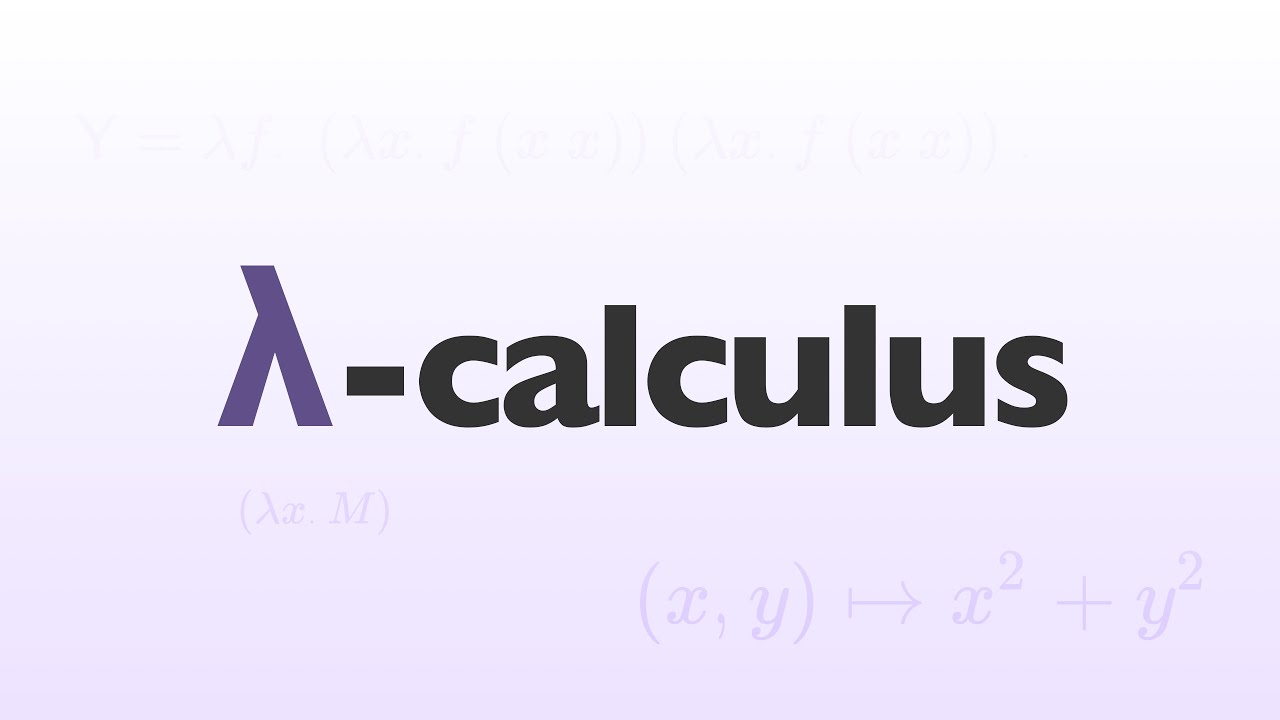
Lambda Calculus: The foundation of functional programming, and the simplest programming language
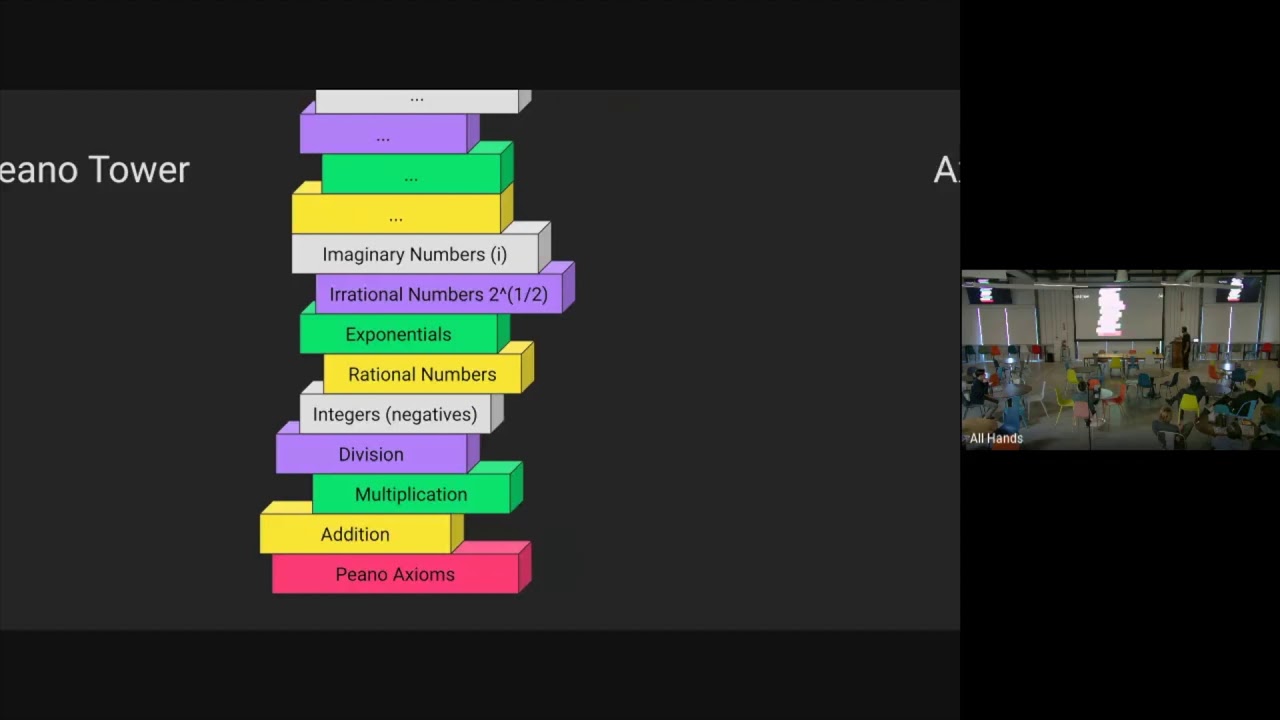
Lambda Calculus vs. Turing Machines (Theory of Computation)
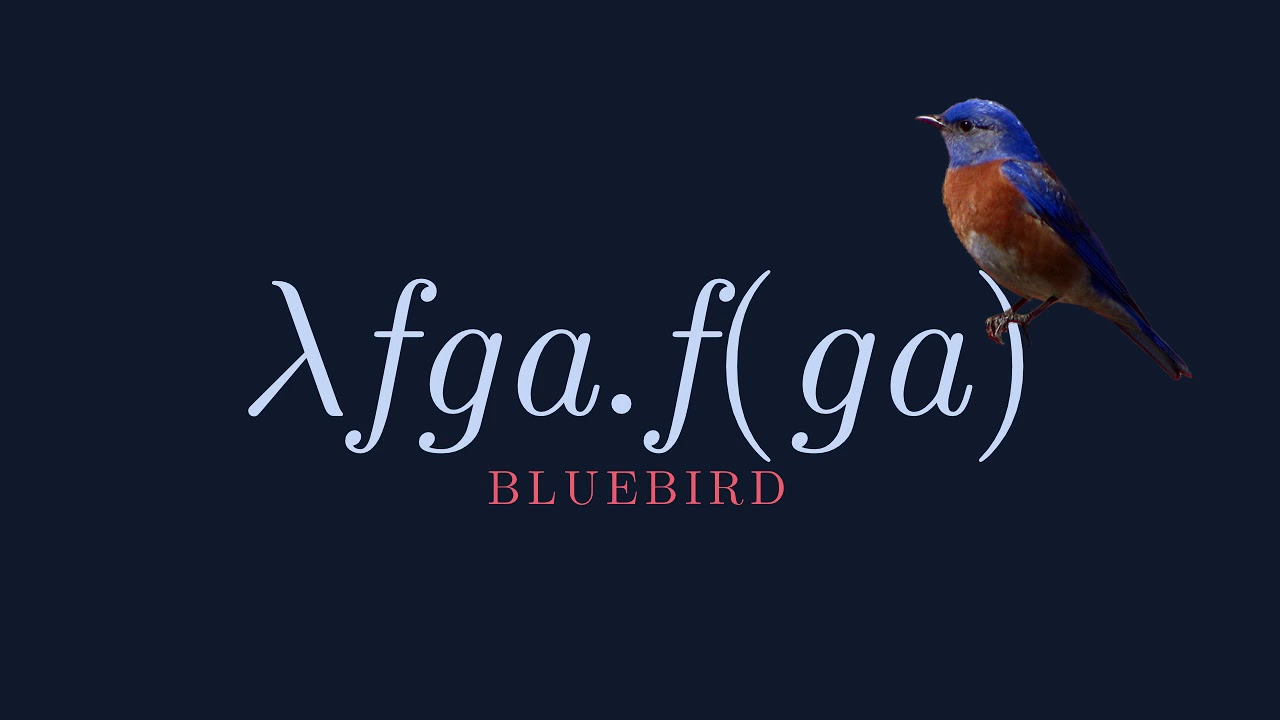
A Flock of Functions: Combinators, Lambda Calculus, & Church Encodings in JS - Part II
5.0 / 5 (0 votes)
Thanks for rating: