A Flock of Functions: Combinators, Lambda Calculus, & Church Encodings in JS - Part II
TLDRThis script delves into the fascinating world of lambda calculus and functional programming, exploring how to represent numbers, arithmetic operations, and data structures without traditional numerical systems. It demonstrates Church numerals for representing integers and constructs like the successor function, addition, multiplication, and exponentiation using function composition. The talk also covers advanced concepts such as the Phi combinator for creating a predecessor function and touches on implementing boolean logic and comparisons. The presenter skillfully guides through these complex topics, aiming to deepen the understanding of functional programming paradigms and their mathematical foundations.
Takeaways
- π The script introduces the concept of Church numerals, a way to represent numbers using functions in lambda calculus.
- π’ It demonstrates how basic arithmetic operations like addition, multiplication, and exponentiation can be expressed using these numerals.
- π€ The script explores the idea of representing 'zero' as a function that returns the second argument, effectively acting as a false value in boolean contexts.
- π The concept of function composition is highlighted as a key method for creating new functions from existing ones, which is crucial in lambda calculus.
- π― The 'B Combinator' is identified as a significant concept for function composition, often mentioned more frequently than even the 'Y Combinator'.
- π The script explains how to define a 'successor' function in lambda calculus, which increments a Church numeral by one.
- π It delves into the creation of a 'predecessor' function, allowing for subtraction by one, which is a complex concept in a system without traditional arithmetic.
- π¦ The transcript discusses data structures like the 'Vireo' or pair, which is a fundamental way to hold two values together in a purely functional context.
- π The 'Phi Combinator' is introduced as a method to shift and increment values within a pair, which can be used to create a predecessor function.
- π The script touches on the implementation of comparison operations like less than, greater than, and equality using Church numerals and combinators.
- π Finally, the script concludes with a discussion on how lambda calculus forms the backbone of functional programming languages, even though they use more efficient methods for handling numbers.
Q & A
What is the main topic discussed in the video script?
-The main topic discussed in the video script is the exploration of lambda calculus, specifically focusing on Church numerals, function composition, and the creation of arithmetic operations without traditional numerical systems.
What are Church numerals and how are they used in the script?
-Church numerals are a way of representing numbers in lambda calculus using functions. In the script, they are used to demonstrate the encoding of numbers as functions, and how operations like addition, multiplication, and exponentiation can be performed using these representations.
What is the significance of the 'successor' function in the context of Church numerals?
-The 'successor' function is crucial as it allows for the creation of the next number in a Church numeral sequence. It is defined in such a way that when applied to a Church numeral, it adds one more application of the function to the argument, effectively incrementing the numeral by one.
How is function composition used in the script to represent multiplication of Church numerals?
-Function composition is used to represent multiplication by creating a series of function applications. The script shows that multiplying two Church numerals can be achieved by composing the functions represented by these numerals, effectively applying one function to the other a certain number of times.
What is the 'Bluebird' Combinator mentioned in the script, and what does it represent?
-The 'Bluebird' Combinator, also known as the B Combinator, represents function composition in lambda calculus. It takes two functions and applies one after the other to an argument, resulting in the composition of the two functions.
How does the script demonstrate the concept of exponentiation using lambda calculus?
-The script demonstrates exponentiation by using the concept of function composition multiple times. It explains that raising a number to a power in lambda calculus involves composing the base function with itself the number of times indicated by the exponent.
What is the 'Phi' Combinator mentioned in the script, and what is its purpose?
-The 'Phi' Combinator is a function that takes a pair and generates a new pair, where the first element of the new pair is the second element of the old pair, and the second element is the successor of the second element of the old pair. It is used in the script to create a predecessor function, which allows for subtraction by one.
How are data structures like pairs represented in lambda calculus as discussed in the script?
-Data structures like pairs are represented in lambda calculus using functions that hold onto two arguments. The script introduces the 'Vario', which takes two arguments and then waits for a function to apply to those arguments, effectively creating a closure that acts as a pair.
What is the significance of the 'Blackbird' Combinator in the context of the script?
-The 'Blackbird' Combinator is used in the script to represent binary function composition, where the rightmost function takes two inputs. It is used to define operations like 'greater than', which cannot be expressed simply with the 'Bluebird' Combinator due to the need for two arguments.
How does the script conclude the discussion on lambda calculus and its relation to functional programming?
-The script concludes by emphasizing that while lambda calculus provides a foundational understanding of functional programming concepts, real functional programming languages use more efficient methods for arithmetic operations and data structures. It also highlights the importance of being comfortable with currying, higher-order functions, and combinators for effective functional programming.
Outlines
π Introduction to Church Numerals and Lambda Calculus
The speaker begins by introducing the concept of Church numerals, a way of representing numbers in lambda calculus without using traditional arithmetic operators or equality. They explain how to represent the numbers 0, 1, 2, and 3 using adverbs like 'once', 'twice', and 'thrice', which apply a function a certain number of times to an argument. The speaker also demonstrates how to use these numerals to perform basic operations like identity and negation, setting the stage for building a number system within lambda calculus.
π’ Successor Function and Dynamic Number Generation
The speaker discusses the challenge of dynamically generating numbers in lambda calculus and introduces the successor function, which takes a Church numeral and returns the next number in the sequence. They illustrate how the successor function works by incrementing the numerals, showing that it adds an extra application of a function to the argument. The concept of function composition is touched upon, and the speaker begins to build a system for converting Church numerals to JavaScript numbers for practical demonstration.
πΌ Function Composition and the Bluebird Combinator
The speaker delves into function composition, a fundamental concept in functional programming, and introduces the Bluebird (B) Combinator, which represents right-to-left function composition. They demonstrate how function composition can be used to create new functions by chaining existing ones. The speaker also shows how the successor function can be expressed as a composition of functions and how this concept can be applied to create more complex operations.
β Binary Addition with Church Numerals
Exploring the theme of adding two Church numerals together, the speaker constructs a lambda calculus function for addition. They explain that adding two numbers can be achieved through n-fold successive applications of the successor function on the other number. The concept of using a combinator to express this addition is introduced, and the speaker provides examples of adding different Church numerals, showcasing the power of function composition in creating arithmetic operations.
βοΈ Multiplication and Exponentiation in Lambda Calculus
The speaker tackles multiplication by explaining how it can be seen as a series of function compositions, specifically the two-fold composition of a three-fold composition of a function. They extend this idea to exponentiation, showing how it can be represented as a nested composition of functions, and introduce a new combinator for exponentiation. The speaker emphasizes the elegance of representing complex arithmetic operations through simple function compositions.
π Inversion and Predecessor Function Using Phi Combinator
In this section, the speaker introduces the concept of inversion in lambda calculus, focusing on creating a predecessor function using the Phi combinator. They demonstrate how the Phi combinator can shift and increment elements of a pair, effectively allowing for the subtraction of one from a Church numeral. The speaker provides a step-by-step explanation of how this combinator can be used to build a predecessor function, showcasing the depth of lambda calculus in performing arithmetic operations.
π Advanced Lambda Calculus: Subraction and Data Structures
The speaker advances the discussion to subtraction and the creation of data structures in lambda calculus. They explain how subtraction can be achieved by applying the predecessor function a certain number of times and introduce the concept of equality and inequality using these arithmetic operations. The speaker also touches on the creation of simple data structures like pairs using the Vireo combinator, laying the groundwork for more complex data structures.
π Conclusion: Implications and Further Exploration
In the concluding part, the speaker reflects on the implications of using lambda calculus for arithmetic and data structure representation. They discuss how real functional programming languages utilize the backbone of lambda calculus while taking shortcuts for practicality. The speaker encourages further exploration of these concepts, emphasizing their value in understanding functional programming paradigms and the potential for creating new functional languages.
π Final Thoughts and Audience Engagement
The speaker wraps up the session by acknowledging the audience's engagement and interest in the topic. They highlight the importance of understanding the foundational concepts of lambda calculus and its role in enhancing one's ability to think functionally. The speaker also invites questions and further discussion, emphasizing the interactive and exploratory nature of learning functional programming and lambda calculus.
Mindmap
Keywords
π‘Lambda Calculus
π‘Church Numerals
π‘Function Application
π‘Identity Function
π‘Successor Function
π‘Function Composition
π‘Combinator
π‘Pair (Vario)
π‘Constant Function
π‘Boolean Logic
π‘Data Structures
Highlights
Introduction to teaching numbers without traditional arithmetic using lambda calculus.
Using adverbs 'once', 'twice', 'thrice' as a substitute for numerical operations.
Exploring the concept of 'once' as a function that applies an argument a single time.
Explanation of 'twice' as applying a function to the result of the function applied to an argument.
Discussion on the creation of a number system using Church encodings.
Demonstration of 'zero' as a function that returns the second argument without applying the function.
Insight on 'one' being represented as the identity function in lambda calculus.
Introduction of the successor function as a method to dynamically generate numbers.
Explanation of how to add numbers using function composition in lambda calculus.
Introduction of the B Combinator as a function for composition, likened to the Bluebird.
The discovery that multiplication in lambda calculus is essentially function composition.
Introduction of exponentiation using Church numerals and combinators.
Explanation of how to check if a Church numeral is zero using the constant false function.
Introduction of the Phi Combinator for creating a predecessor function for subtraction.
Discussion on building data structures like pairs in lambda calculus using closures.
Explanation of how to perform subtraction using the predecessor function.
Introduction of the Blackbird Combinator for binary function composition.
Conclusion emphasizing the practical applications of lambda calculus in functional programming languages.
Encouragement for further exploration into building functional programming languages and data structures.
Transcripts
Browse More Related Video
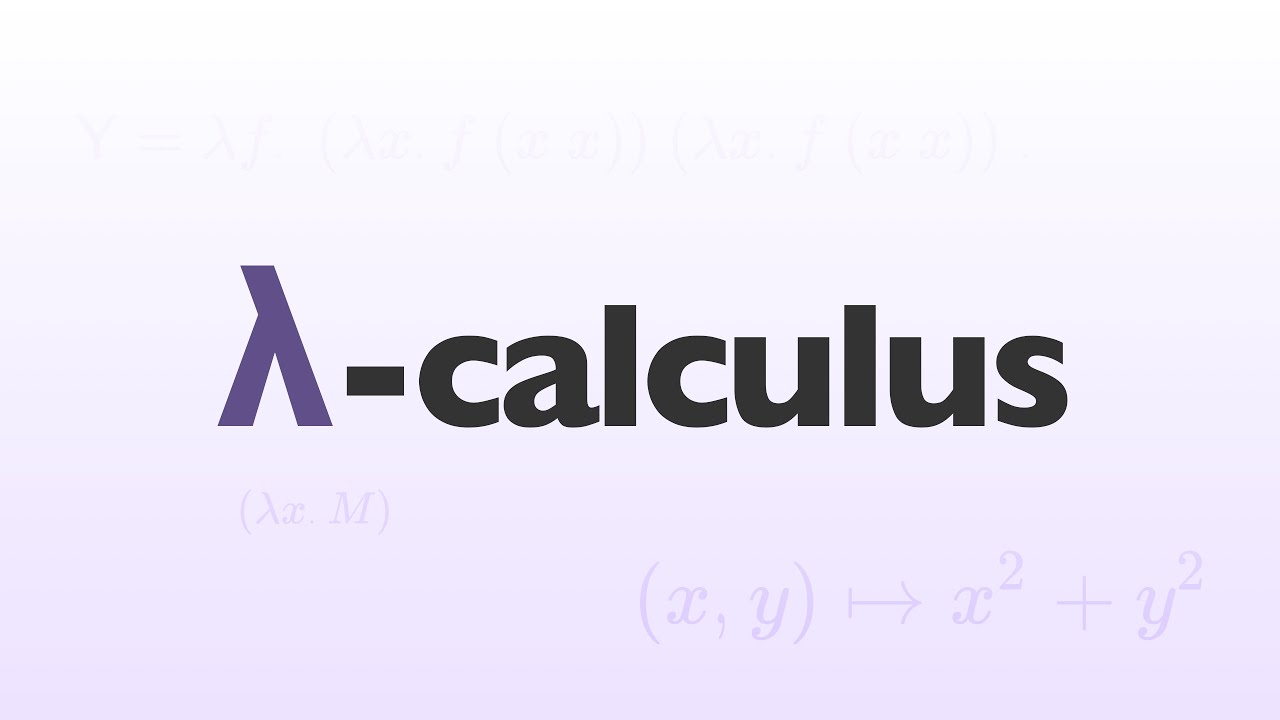
Lambda Calculus: The foundation of functional programming, and the simplest programming language
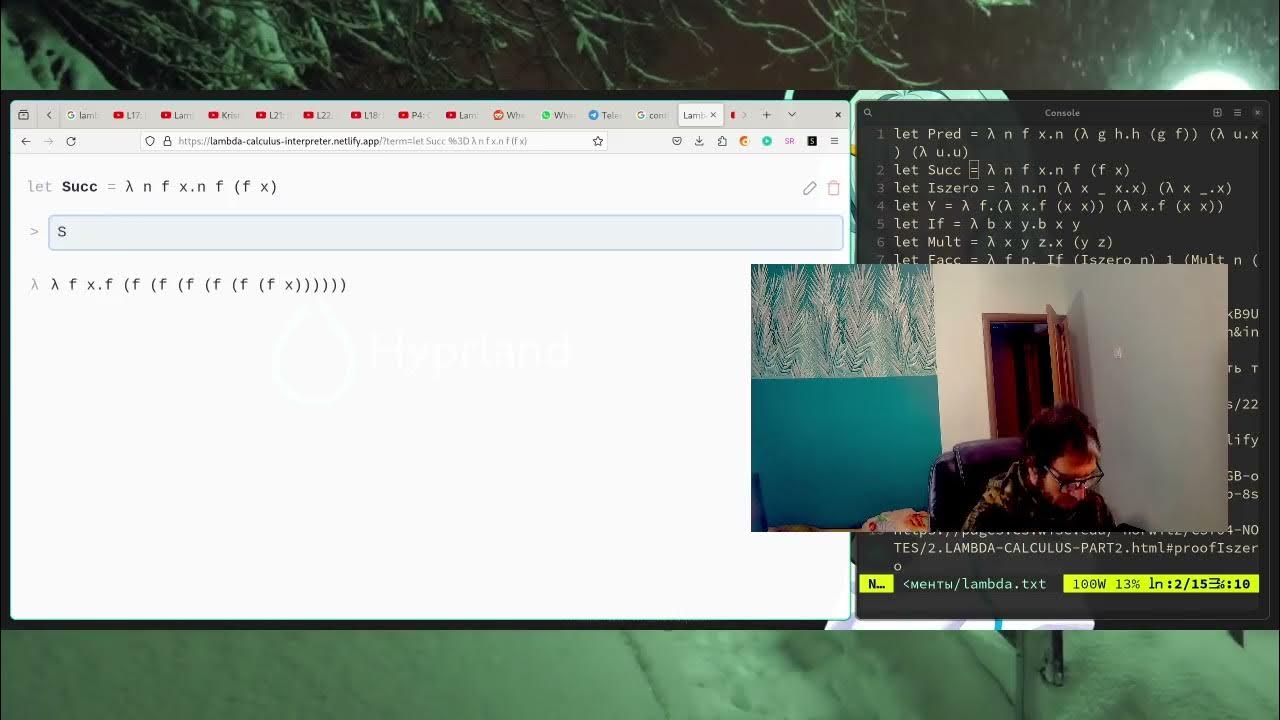
Lambda Calculus Calculator
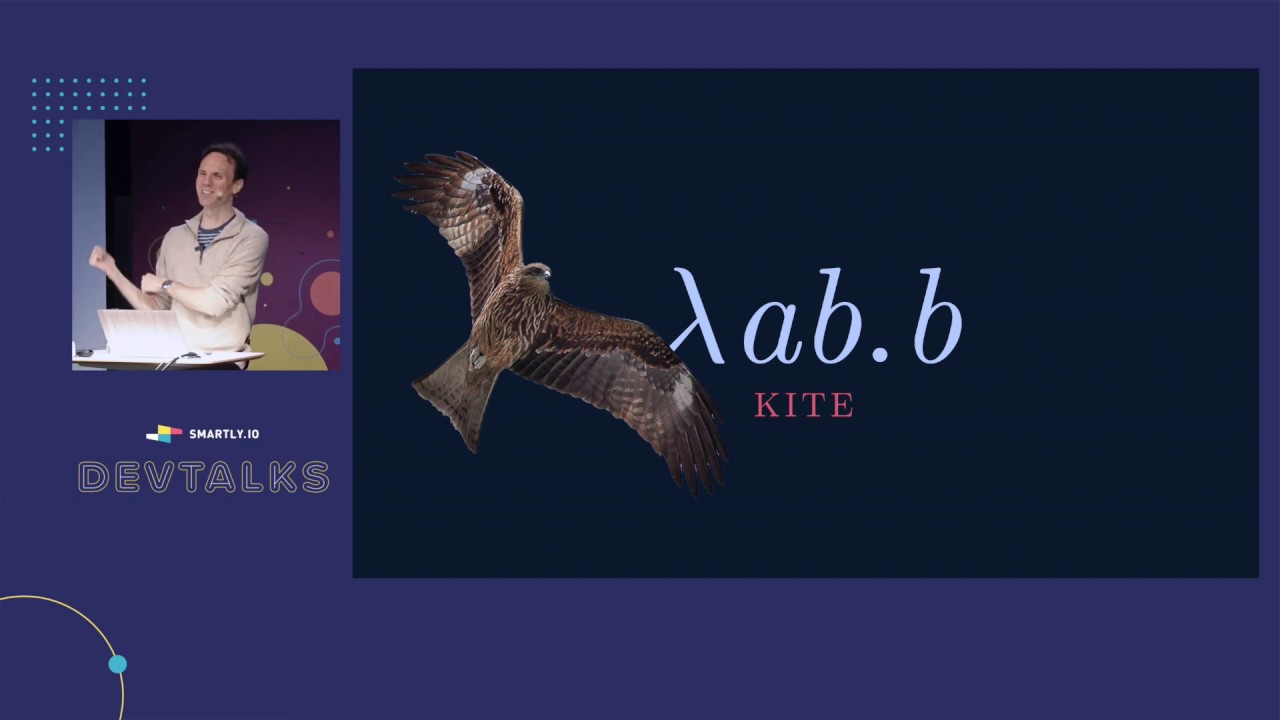
A Flock of Functions: Lambda Calculus and Combinatory Logic in JavaScript | Gabriel Lebec @ DevTalks
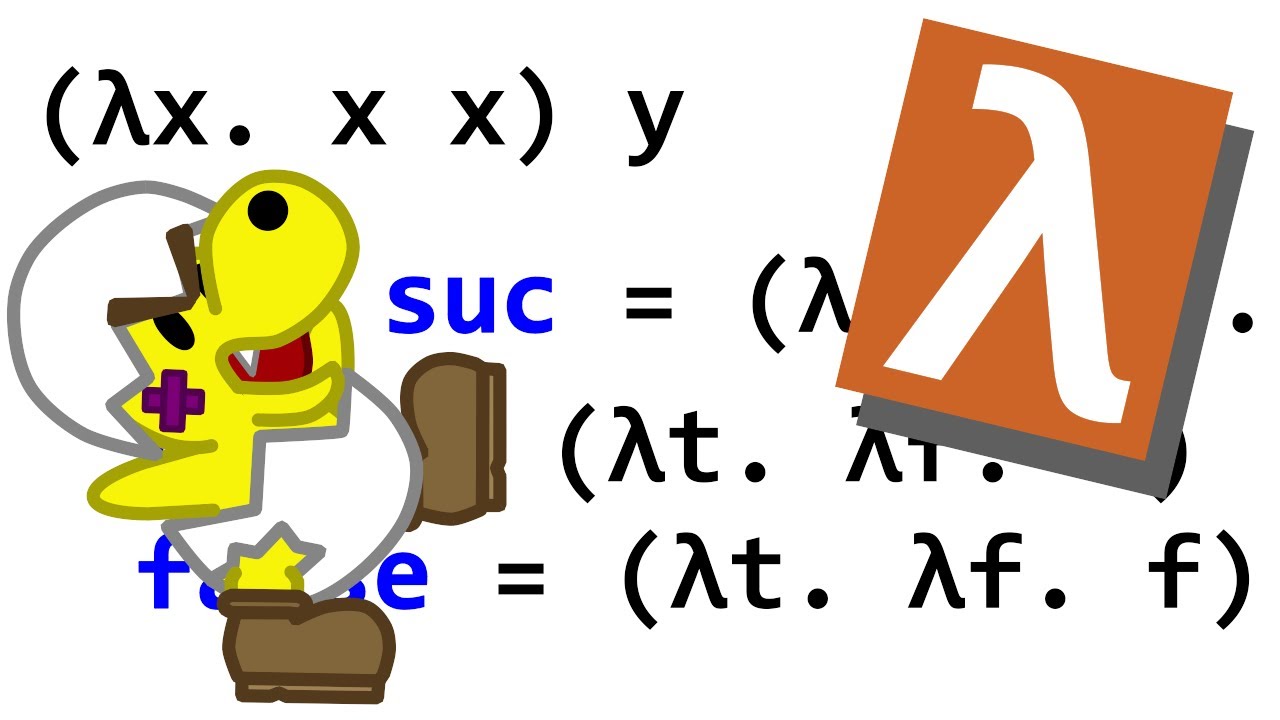
Lambda Calculus!

PLT: Lambda Calculus - Basics 3 (Operations on church numerals)
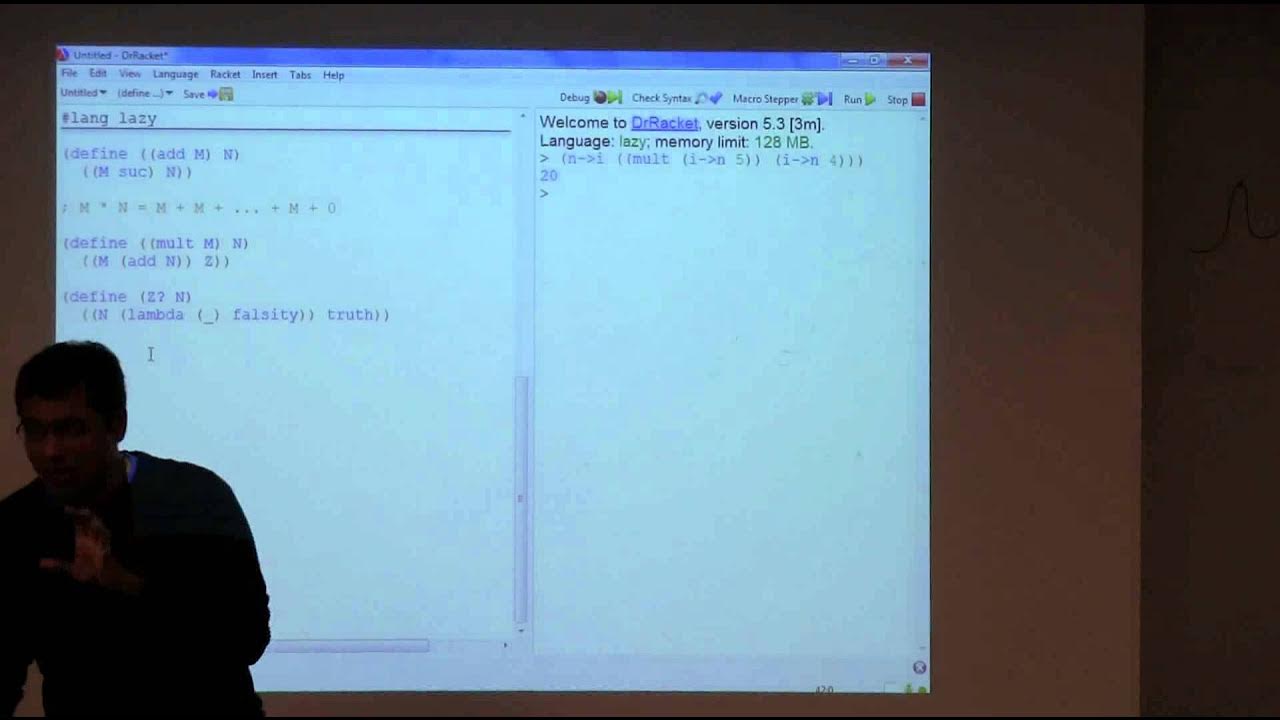
Programming Languages - The Lambda Lecture
5.0 / 5 (0 votes)
Thanks for rating: