JSON Parser 100% From Scratch in Haskell (only 111 lines)
TLDRIn this detailed tutorial, the presenter embarks on a journey to implement a JSON parser in Haskell from scratch, leveraging the language's standard library and avoiding third-party dependencies. They introduce the concept of parser combinators and demonstrate how to construct parsers for various JSON data types, such as nulls, booleans, numbers, strings, arrays, and objects. The stream is interactive, with the audience contributing to troubleshooting and testing the parser with real-world JSON data, showcasing Haskell's powerful type system and functional programming capabilities.
Takeaways
- π The video demonstrates building a JSON parser in Haskell from scratch, emphasizing the use of parser combinators and the standard library.
- π οΈ It highlights the ease of implementing compilers and parsers in Haskell due to its strong support for these tasks.
- π The idea for the project originated from implementing a parser for a DSL on a boat development stream, showcasing the versatility of the approach.
- π The presenter encourages avoiding third-party dependencies to increase code maintainability, although it may result in a slower parser.
- π The process involves creating an Abstract Syntax Tree (AST) to define the structure of JSON data, including types for null, boolean, numbers, strings, arrays, and objects.
- π’ The parser supports only integers and not floating-point numbers for simplicity, but the code will be open-sourced for community contributions.
- π The video walks through the creation of a Haskell project folder, setting up the development environment, and writing the initial code for the parser.
- π The implementation of the parser involves defining a 'Parser' type, utilizing 'Maybe' for error handling, and creating small parsers for individual JSON components.
- π§ The video discusses the use of 'Functor', 'Applicative', and 'Alternative' type classes in Haskell to build complex parsers from simpler ones.
- π The presenter demonstrates the incremental development of the parser, starting with simple types like 'null' and 'boolean', and gradually adding support for more complex types.
- π» The final result is a fully functional JSON parser implemented in under 111 lines of code, capable of parsing a real-world JSON dataset after removing escape characters for simplicity.
Q & A
What is the main goal of the video script?
-The main goal of the video script is to demonstrate how to implement a JSON parser in Haskell from scratch using only the base standard library, without any third-party dependencies.
What programming concept is central to the implementation of the JSON parser discussed in the script?
-The central programming concept is 'parser combinators,' which are used to build complex parsers from simpler ones in a functional programming context.
Why is the idea of implementing a JSON parser in Haskell appealing to the script's author?
-The author finds the idea appealing because Haskell makes it relatively easy to implement compilers and parsers due to its strong support for these tasks, and the author was surprised by how easy it was to create a basic JSON parser.
What is the significance of using 'parser combinators' in Haskell for implementing the JSON parser?
-Parser combinators allow for the construction of complex parsers in a modular way, making the code easier to understand and maintain. They also help in avoiding third-party dependencies, thus increasing the maintainability of the code.
How does the script's author handle the challenge of not using third-party libraries like 'parsec'?
-The author tackles this challenge by implementing their own basic parser combinators to parse JSON, despite the potential for the parser to be less efficient or feature-complete than 'parsec'.
What is the 'AST' mentioned in the script, and why is it important for the JSON parser?
-The 'AST' stands for Abstract Syntax Tree, which is a tree representation of the structure of JSON data. It is important because it defines the types that the parser will construct while parsing JSON, allowing for a structured interpretation of the data.
Why does the script mention 'maintainability' in the context of not using third-party dependencies?
-The script mentions 'maintainability' because having full control over the code without third-party dependencies means that changes can be made quickly and directly without needing to wait for external library updates or reviews.
What is the role of 'functor', 'applicative', and 'alternative' in the context of the Haskell JSON parser implementation?
-These typeclasses play a crucial role in the implementation of the parser. 'Functor' allows for value transformation within the parser, 'applicative' provides a way to apply functions within the parser, and 'alternative' lets the parser choose between multiple parsing options, enhancing the flexibility of the parser combinators.
How does the script's author handle the complexity of implementing a JSON parser?
-The author manages complexity by breaking down the task into smaller, manageable functions and using Haskell's powerful type system and features like 'holes' to guide the implementation process.
What is the final outcome of the script's demonstration?
-The final outcome is a working JSON parser implemented in Haskell that can parse a variety of JSON data types, including objects, arrays, strings, numbers, booleans, and null values, all from scratch and without third-party dependencies.
Outlines
π Introduction to Building a JSON Parser in Haskell
The video begins with the presenter's intention to create a JSON parser from scratch in Haskell without relying on third-party dependencies, using only the base library. The idea originated from a development stream and aims to demonstrate the simplicity of implementing parsers in Haskell, specifically parser combinators. The presenter emphasizes the benefits of not depending on external libraries for increased maintainability and control over the code.
π Setting Up the Haskell JSON Parser Project
The presenter proceeds to set up a new Haskell project specifically for the JSON parser, navigating through the file system to create a dedicated folder. They initiate Emacs within the project directory and start GHCi, the interactive Haskell environment. The presenter also discusses the need for a main module and an entry point, hinting at the structure of Haskell projects and the importance of the main function.
π Defining the Abstract Syntax Tree (AST) for JSON
The presenter outlines the process of defining an AST for JSON, which includes various JSON value types such as null, boolean, numbers, strings, arrays, and objects. They decide to simplify the parser by only supporting integers and not floats, and discuss the challenges of implementing a map without using third-party dependencies. The presenter also mentions the intention to derive interfaces like 'Show' and 'Eq' for the JSON types.
π Deep Dive into Parser Combinators in Haskell
The presenter delves into the concept of parser combinators, explaining how they can be used to create small, composable parsers that can be combined to parse more complex structures. They introduce the idea of a 'parser' type that takes a string and returns a value along with the remaining input. The presenter also discusses error handling in parsers, considering the use of 'Maybe' for simplicity.
π Implementing a Character Parser in Haskell
The presenter begins coding by implementing a basic character parser, which takes a single character as input and returns it wrapped in a 'Maybe' type. They demonstrate the use of Haskell's 'holes' feature for guided development and pattern matching to handle different input scenarios. The video illustrates the fundamental steps in creating a parser in Haskell and the importance of managing the remaining input for subsequent parsers.
π Understanding Parser Composition and Applicative Functors
The presenter discusses the concept of parser composition, introducing the idea of turning a list of parsers into a single parser using the 'sequenceA' function from the 'Applicative' typeclass. They explain the need to prove that the parser is an 'Applicative' functor, which includes implementing 'fmap' and 'pure' functions, and demonstrate the use of 'sequenceA' to combine parsers.
π Implementing Functor and Applicative Instances for Parsers
The presenter works on implementing the 'Functor' and 'Applicative' instances for the parser type. They define 'fmap' for the parser, allowing functions to be applied within the parser context. Then, they begin implementing 'Applicative' by defining the 'pure' function, which creates a parser that always returns a given value, ignoring the input.
π Advancing JSON Parser with Alternative Instances
The presenter advances the JSON parser by implementing 'Alternative' instances, allowing for the creation of parsers that can try multiple options and return the first successful result. They discuss the 'empty' function as a parser that always fails and the '(<|>)' function for combining parsers, which is crucial for implementing boolean values in JSON.
π’ Parsing JSON Numbers with Custom Parser Combinators
The presenter tackles the parsing of JSON numbers by implementing a custom parser combinator that applies a predicate to a sequence of characters. They introduce the 'spanP' function, which parses characters as long as the predicate holds, and use it to parse sequences of digits, converting them into integers and wrapping them into JSON number values.
π Implementing JSON String Parsing
The presenter moves on to parsing JSON strings, utilizing applicative functor operations to create a parser that expects a string literal enclosed in double quotes. They discuss the limitations of their implementation, noting that it does not support escape characters for simplicity, and demonstrate how to wrap the parser's output in a JSON string value.
π’ Parsing JSON Arrays with Recursive Descent
The presenter explains how to parse JSON arrays by creating a recursive parser that expects a sequence of JSON values enclosed in square brackets and separated by commas. They introduce the concept of 'many' to handle multiple elements and discuss the challenges of parsing nested structures within the JSON array.
π Crafting JSON Object Parser
The presenter tackles the parsing of JSON objects, which involve key-value pairs enclosed in curly braces. They discuss the structure of JSON pairs and the need to parse string literals, colons, and separators. The presenter outlines the steps to combine these elements into a parser for JSON objects.
π Debugging and Testing the JSON Parser
The presenter tests the JSON parser with a complex real-world JSON data from a schedule page. They encounter issues with parsing due to the lack of support for escaped quotes and work through debugging the parser to handle the real data. The presenter demonstrates the parser's ability to extract specific information from the JSON data.
π Conclusion and Reflection on the JSON Parser Implementation
In the conclusion, the presenter reflects on the process of implementing the JSON parser from scratch in Haskell. They discuss the challenges and learnings from the experience, emphasizing the educational value of the exercise. The presenter also highlights the simplicity of creating a basic parser using Haskell's typeclasses and combinators.
Mindmap
Keywords
π‘JSON
π‘Haskell
π‘Parser Combinators
π‘Abstract Syntax Tree (AST)
π‘Functor
π‘Applicative
π‘Alternative
π‘Monad
π‘Recursion
π‘Maintainability
Highlights
Introduction to implementing a JSON parser in Haskell using only the base library.
Explanation of the simplicity of implementing parsers in Haskell.
The idea for the project was born during a boat development stream.
Demonstration of creating a folder and starting a Haskell project.
Introduction to the concept of parser combinators.
Explanation of the benefits of not using third-party dependencies for parsers.
Starting GHCi and setting up the initial Haskell file.
Defining the abstract syntax tree (AST) for JSON in Haskell.
Discussion on the limitations of using only the base library for parsing JSON.
Introduction to the concept of records in Haskell and their use in defining parsers.
Explanation of how to create a parser type in Haskell.
Demonstration of implementing a parser for a single character.
Introduction to the concept of 'holes' in Haskell for guided implementation.
Explanation of how to parse a sequence of characters using parser combinators.
Demonstration of implementing a parser for JSON null values.
Introduction to the concept of applicative functors in Haskell.
Explanation of how to implement a parser for JSON boolean values.
Demonstration of combining parsers to parse more complex JSON structures.
Introduction to the concept of 'traversable' and 'applicative' in Haskell.
Explanation of how to implement a parser for JSON strings.
Demonstration of implementing a parser for JSON arrays.
Introduction to the concept of 'alternative' in Haskell for parser combinators.
Explanation of how to implement a parser for JSON objects.
Demonstration of parsing a real-world JSON file using the custom Haskell parser.
Conclusion and summary of the entire process of implementing a JSON parser from scratch in Haskell.
Transcripts
Browse More Related Video

Understanding parser combinators: a deep dive - Scott Wlaschin
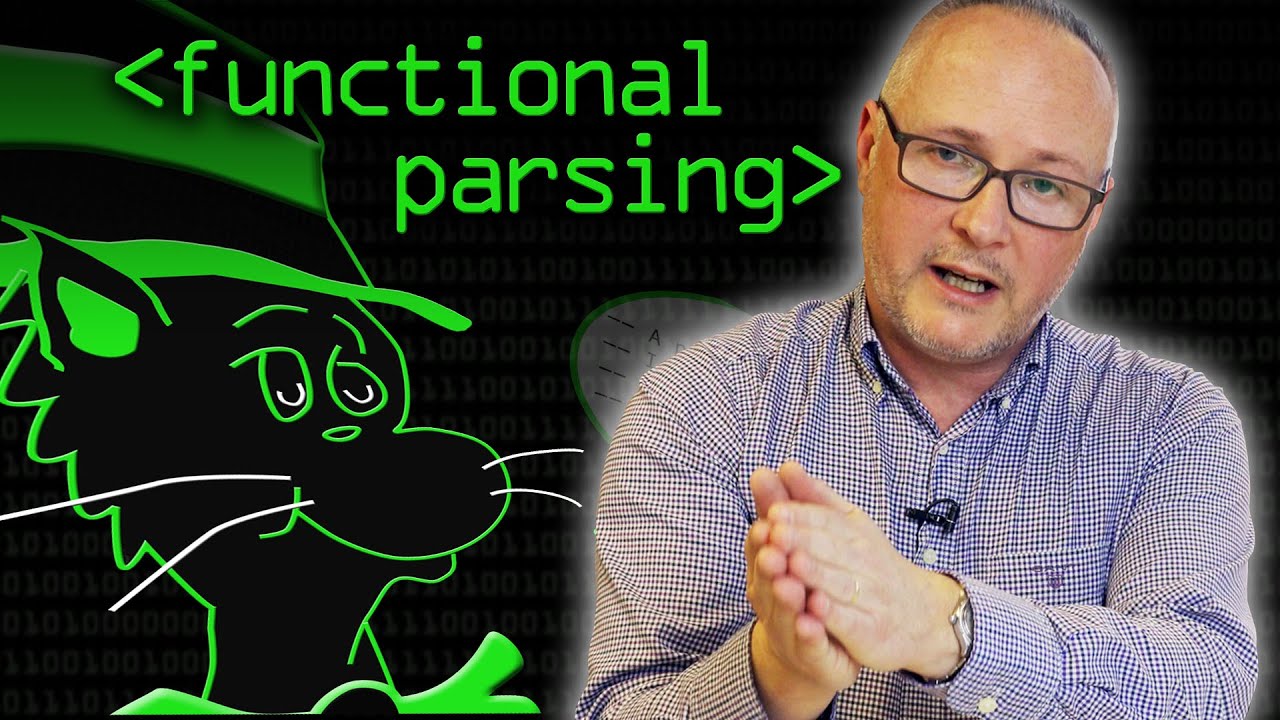
Functional Parsing - Computerphile
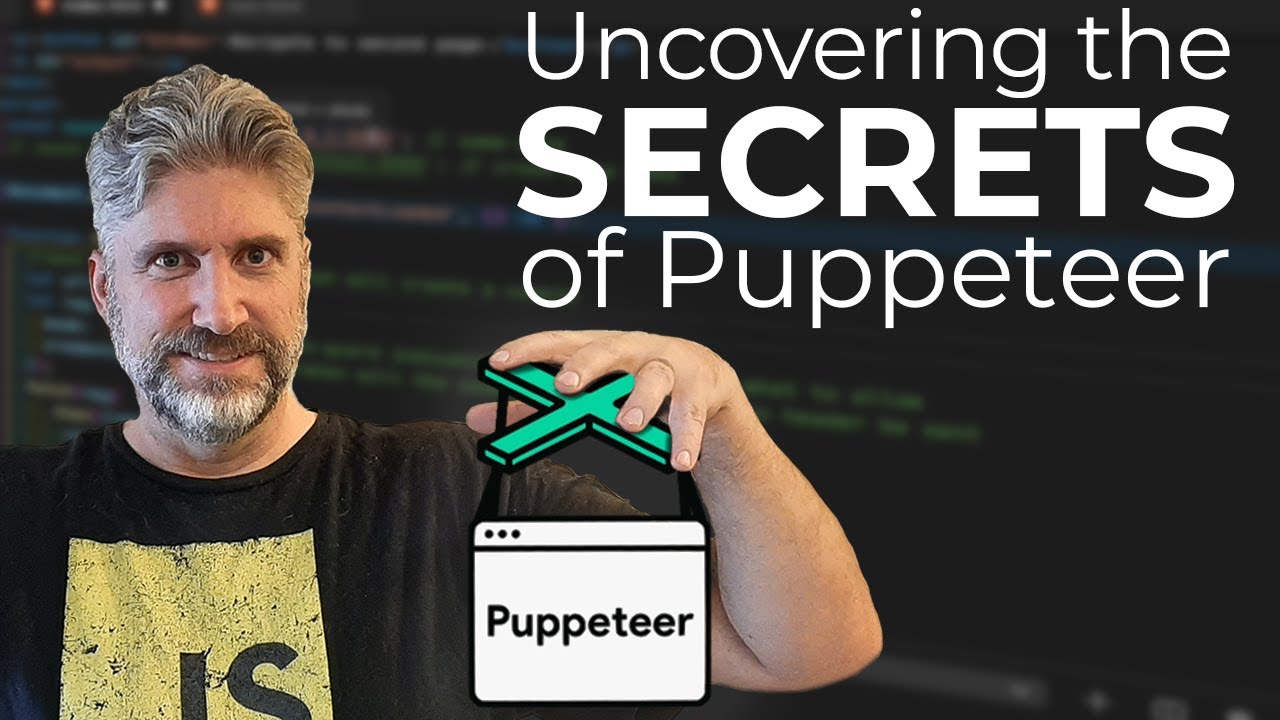
Puppeteer: Headless Automated Testing, Scraping, and Downloading

ParseHub Tutorial: Pagination (no 'next' button)

Functional Programming & Haskell - Computerphile

Lambda Calculus - Fundamentals of Lambda Calculus & Functional Programming in JavaScript
5.0 / 5 (0 votes)
Thanks for rating: