Understanding parser combinators: a deep dive - Scott Wlaschin
TLDRIn this talk, Scott Lott introduces the concept of parser combinators, demonstrating how they can be used to build complex parsers from simple ones in functional programming. Using F# as an example, he covers basic combinators, error handling, and even constructs a JSON parser, emphasizing the power of composition in functional programming.
Takeaways
- π The talk by Scott Lott introduces the concept of parser combinators, aiming to demystify the code and make it less intimidating for learners.
- π Parser combinators are recipes for creating parsers that can be combined to form more complex parsers, emphasizing the power of composition in functional programming.
- π» Scott uses F# for code examples but notes that the concepts apply to most programming languages, excluding older ones like COBOL.
- π The talk covers building a simple parser, understanding combinators, and using them to construct more complex parsing logic, including a JSON parser.
- π οΈ Parser combinators allow writing parsers directly in the preferred programming language, avoiding the need for separate lexing and parsing stages.
- π The benefits of using parser combinators include ease of use, the ability to create DSLs quickly, and enhancing understanding of functional composition.
- π Scott demonstrates the creation of basic parsers and combinators, such as 'and then', 'orElse', and 'map', which are fundamental in building more complex parsers.
- π The talk also covers advanced combinators like 'many', 'many1', 'optional', and 'between', which are used for parsing repeated elements or elements within delimiters.
- π Error handling in parsers is improved by labeling parsers for clearer messages and incorporating line and column information for easier debugging.
- π’ The JSON parser example shows how to break down the JSON specification into smaller parsing tasks and then combine them into a full parser for JSON values.
- π The entire parser library, including JSON parsing, is demonstrated to be quite concise, highlighting the efficiency and power of parser combinators in building parsers.
Q & A
What is the main topic of Scott Lotion's talk?
-The main topic of Scott Lotion's talk is understanding parsers and combinators, specifically using F# for code examples.
Why is the syntax with strange symbols in parser combinator code intimidating to newcomers?
-The syntax with strange symbols like vertical bars and angle brackets with dots can be intimidating because it is unfamiliar and looks complex, making it difficult for newcomers to understand at first glance.
What is a parser combinator library?
-A parser combinator library is a set of functions that can be combined to create parsers. It allows for the construction of a parsing recipe that can match patterns in text, and these recipes can be combined to form more complex parsers.
Why are parser combinators preferred over traditional parsing techniques like lex and yacc?
-Parser combinators are preferred because they are written in the programmer's favorite language, eliminating the need for a separate lexing stage and preprocessing. They are also interactive and can be used to quickly create Domain Specific Languages (DSLs).
What is the basic concept behind a parser combinator?
-The basic concept behind a parser combinator is to create a recipe that, when run later, will match a specific pattern in the input. Combining these recipes allows for the creation of more complex parsers from simpler ones.
How does Scott Lotion demonstrate the creation of a simple parser in his talk?
-Scott Lotion demonstrates the creation of a simple parser by starting with a function that matches a single character (e.g., 'a'). He then builds upon this by making the character matchable dynamic and adding error handling to improve the parser's functionality.
What are the benefits of using immutable inputs and outputs in functional programming?
-Using immutable inputs and outputs in functional programming ensures that if parsing fails, another parser can be applied to the same input without worrying about the file pointers moving or the input being altered, maintaining consistency and predictability.
What is the purpose of the 'and then' combinator in parser combinator libraries?
-The 'and then' combinator is used to chain parsers in sequence. It runs the first parser, and if it succeeds, it takes the remaining input and runs the second parser, combining their results if both succeed.
How does the 'or else' combinator work in parser combinator libraries?
-The 'or else' combinator allows for alternative parsing paths. If the first parser fails, it takes the original input and tries the second parser. It returns the result of the second parser if it succeeds, otherwise, it returns the failure of the second parser.
What is the significance of the 'map' combinator in parser combinator libraries?
-The 'map' combinator is used to transform the output of a parser. If the parser succeeds, it applies a function to the result, changing it into something else, such as converting a string of digits into an integer.
Outlines
π Introduction to Parser Combinators
Scott Lotion introduces the concept of parser combinators, emphasizing their role in simplifying the process of writing parsers. He mentions his website and the availability of code and slides, setting the stage for a fast-paced talk covering a lot of ground in a short time. The goal is to demystify the intimidating code often associated with parser combinators and to show how they can be used in any programming language, except for COBOL. The talk will cover building a simple parser, understanding combinator libraries, and eventually creating a JSON parser using these techniques.
π Understanding Parser Combinators
The speaker delves into the basics of parser combinators, explaining them as recipes for creating parsers. He discusses the advantages of using parser combinators, such as writing parsers in your favorite programming language and the absence of a need for pre-processing. The talk introduces the concept of composition in functional programming and how it applies to building parsers from smaller components. The speaker also demonstrates a simple parser for matching a character and discusses the importance of immutability in functional programming.
π Building a Basic Parser
Scott Lotion demonstrates how to build a basic parser that matches a specific character, explaining the process of creating a function that returns true or false based on whether the character matches. He then enhances the parser to be more flexible by allowing any character to be matched and discusses the importance of error messages in parsing. The speaker introduces the concept of union types to handle different return types in functional programming and shows how to compile a parser that returns a character and the remaining input or an error message.
π Advanced Parser Techniques
The speaker introduces advanced techniques for building parsers, such as returning a function from a function to handle unknown input. He explains how to create a parser that returns another function, which can then be run with an input stream to perform the actual parsing. This approach allows for the creation of more complex parsers by combining simpler ones. The speaker also demonstrates how to run a parser with an input and how to handle the success or failure of the parsing process.
π Combining Parsers with Combinators
Scott Lotion discusses the concept of combinators, which are functions that combine parsers to create new ones. He introduces three basic combinators: 'and then', 'or else', and 'map'. The 'and then' combinator chains parsers in sequence, the 'or else' allows for alternative parsing options, and the 'map' transforms the output of a parser. The speaker provides code examples and demonstrates how these combinators can be used to build more complex parsing logic, such as parsing strings and integers.
π Complex Parser Construction
The speaker demonstrates how to construct more complex parsers by combining basic ones. He introduces the concept of reducing a list of parsers using an operator, such as 'and then' or 'or else', to create a single parser. This allows for the creation of parsers that can match a sequence of characters or choose between multiple options. The speaker also shows how to create a parser for strings by mapping each character to a parser and using the 'sequence' combinator to combine them.
π Enhancing Parsers with Labels and Error Handling
Scott Lotion discusses how to improve parser error messages by labeling parsers, which helps in providing more descriptive error messages when parsing fails. He also introduces the concept of tracking line and column numbers to enhance error reporting. The speaker demonstrates how to modify the input object to include line and column information, which can then be used to provide more detailed error messages, making it easier for users to understand where parsing errors occur.
π Building a JSON Parser
The speaker begins building a JSON parser by defining the structure of a JSON value using a choice type in F#. He then starts parsing simple JSON literals like 'null', 'true', and 'false'. The speaker uses helper operators and symbols to create parsers for these literals and discusses the importance of labels for improving error messages. The talk moves on to parsing strings, breaking down the process into smaller components and combining them to form a complete parser for JSON strings.
π’ Parsing JSON Numbers and Objects
Scott Lotion continues building the JSON parser by tackling the parsing of numbers. He breaks down the number parsing into components such as an optional sign, an integer part, a fractional part, and an exponent part. The speaker demonstrates how to combine these components using combinators to create a parser for JSON numbers. He also discusses the parsing of JSON objects, showing how to handle nested structures and the use of labels to improve error messages.
π Finalizing the JSON Parser and Demonstrating Its Use
The speaker finalizes the JSON parser by combining all the individual parsers for different JSON value types into a single parser. He demonstrates the parser's ability to handle complex JSON structures, including strings, numbers, objects, arrays, booleans, and null values. The speaker also shows how the parser provides helpful error messages when encountering unexpected characters or structures. The talk concludes with a summary of the power of parser combinators and their role in functional programming.
π Conclusion and Resources
In conclusion, Scott Lotion emphasizes the power of treating functions as objects and the benefits of using parser combinators in functional programming. He highlights the ability to build complex parsers from simple ones and the ease of combining them into larger programs. The speaker provides information on where to find the code and offers assistance for those interested in F# consulting. He encourages attendees to approach him with any questions and reminds them to fill out feedback forms.
Mindmap
Keywords
π‘Parser Combinators
π‘Functional Programming
π‘Composition
π‘JSON Parser
π‘F#
π‘Immutable Data
π‘DSL (Domain-Specific Language)
π‘Parsing
π‘Error Handling
π‘Type Safety
π‘Railroad Diagrams
Highlights
Introduction to parser combinators and their use in understanding code.
The goal of the talk is to demystify parser combinator code.
Using F# for code examples, but concepts apply to most programming languages.
Definition of a parser combinator library and its role in creating parsing recipes.
Explanation of how parsers are combined to create more complex parsers.
Advantages of parser combinators over traditional parsing techniques like Lex and Yacc.
Demonstration of a simple parser for matching a character.
Introduction of a more flexible parser that can match any character.
Discussion on the importance of immutability in parser inputs and outputs.
Introduction of union types to handle different return values in parsers.
Transformation of a simple parser into a higher-order function.
Explanation of how to run a parser with an input stream.
Introduction to basic combinators like 'and then', 'or else', and 'map'.
Demonstration of combining parsers using 'and then' and 'or else'.
Explanation of how to use 'map' to transform parser outputs.
Introduction to more complex combinators like 'reduce' and 'sequence'.
Demonstration of creating a parser for strings using the 'sequence' combinator.
Discussion on improving parser error messages with named parsers.
Introduction to building a JSON parser using parser combinators.
Explanation of how to parse JSON literals like 'null', 'true', and 'false'.
Demonstration of parsing JSON strings and handling escaped characters.
Introduction to parsing JSON numbers and handling different parts of a number.
Final demonstration of a complete JSON parser in action.
Conclusion on the power of parser combinators and their practical applications.
Transcripts
Browse More Related Video

JSON Parser 100% From Scratch in Haskell (only 111 lines)
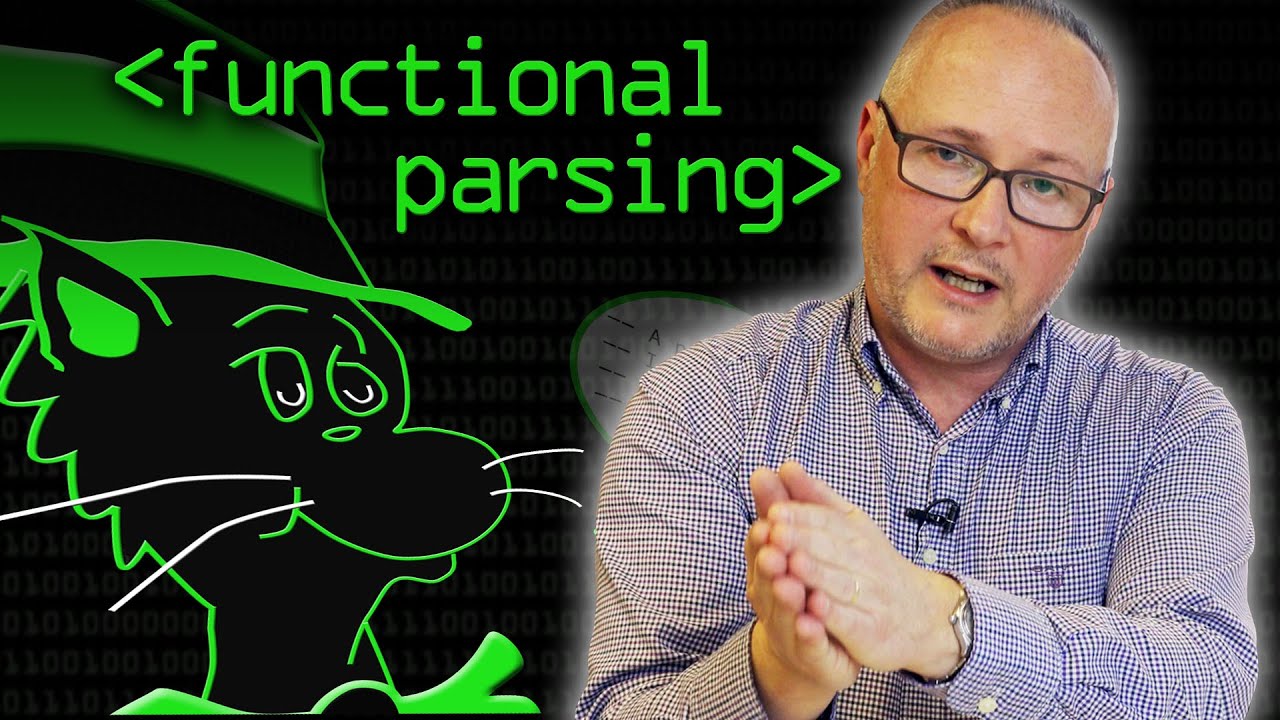
Functional Parsing - Computerphile

Lambda Calculus - Fundamentals of Lambda Calculus & Functional Programming in JavaScript

Introduction to Combinatory Logic βΒ #SoME2
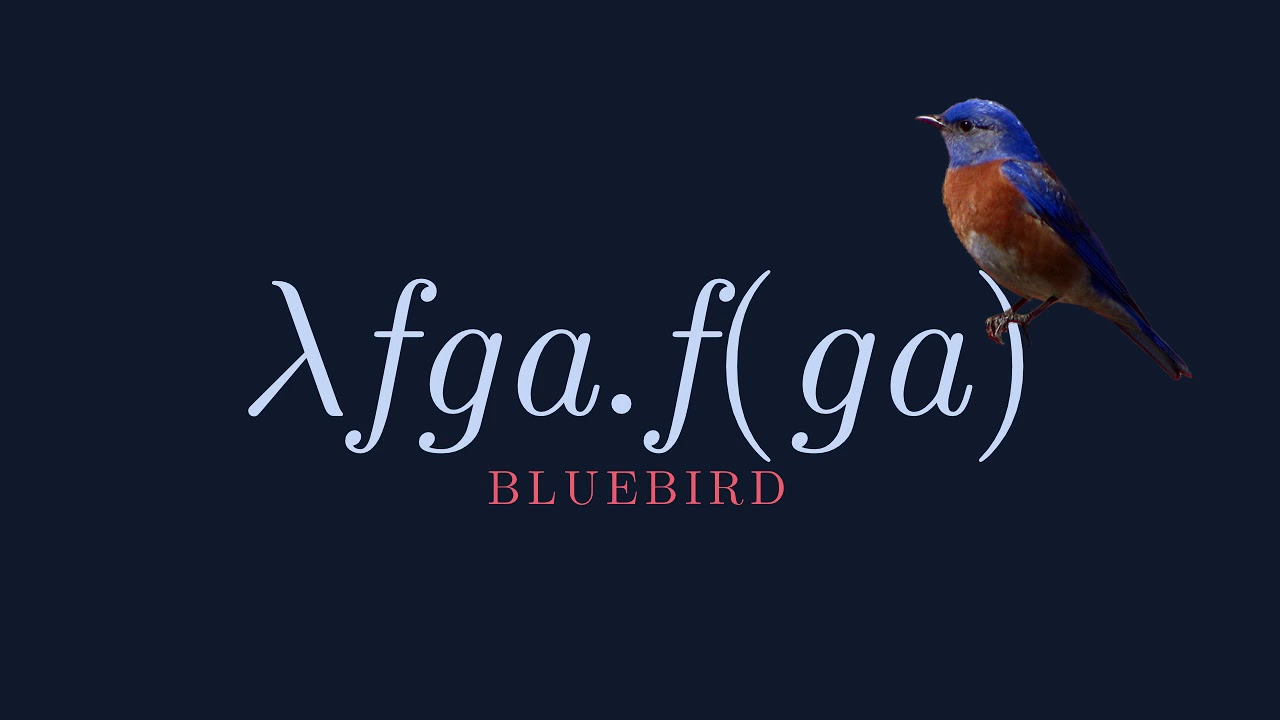
A Flock of Functions: Combinators, Lambda Calculus, & Church Encodings in JS - Part II
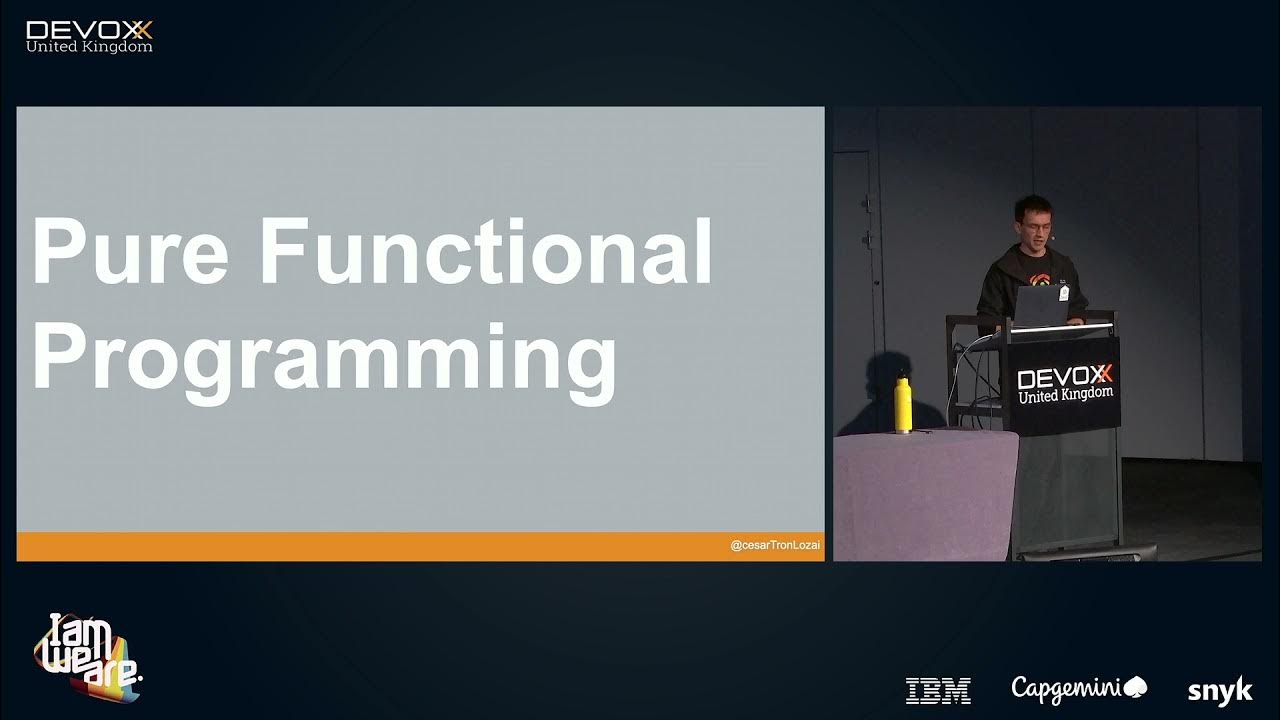
No Nonsense Monad & Functor - The foundation of Functional Programming by CΓ©sar Tron-Lozai
5.0 / 5 (0 votes)
Thanks for rating: