Jump-Starting Lambda Programming
TLDRStuart Marks from the JDK group introduces lambda expressions in Java 8, emphasizing their role in treating code as data and parameterizing behavior. He illustrates how lambdas, combined with new JVM and library changes, enable a more flexible and productive programming style, suitable for a wide range of tasks from everyday coding to parallel programming. The presentation showcases the transformation of a simple example, demonstrating the power of lambdas in creating more expressive and maintainable code.
Takeaways
- π Lambda expressions in Java 8 allow for the treatment of code as data, enabling parameterization of behavior which can transform how programs are written.
- π Java 8 introduces functional interfaces, which are interfaces with a single method, facilitating the use of lambda expressions for behavior parameterization.
- π The presentation by Stuart Marks and his colleague provides a gentle introduction to lambda, contrasting with the more information-dense talks by Brian Goetz.
- π Lambda is not just a language feature; it involves changes to the JVM and class libraries, enabling a new programming style that is both flexible and can increase productivity.
- π§ Lambda expressions can be used to simplify everyday programming tasks as well as complex ones, such as writing highly parallel programs.
- π The script demonstrates the evolution from using simple values to parameterizing behavior using lambda expressions, which can greatly reduce boilerplate code.
- π§ The concept of 'predicates' is introduced as a special type of functional interface that takes an object and returns a boolean, useful for conditions like eligibility checks.
- π The script uses examples of 'mappers' and 'blocks', other types of functional interfaces, to illustrate how different operations can be parameterized and chained.
- π The introduction of the 'stream' API in Java 8 supports creating a pipeline of operations on a collection of objects, with the ability to run these pipelines in parallel.
- π The script covers the use of lambda expressions to refactor code, moving from imperative style with loops and conditions to a more declarative style using streams and lambdas.
- π When using parallel streams, care must be taken to ensure thread safety and to avoid side effects, as the order of execution is not guaranteed.
Q & A
What is the main focus of Stuart Marks' presentation?
-The main focus of Stuart Marks' presentation is to introduce lambda expressions in Java, explaining how they allow for the parameterization of behavior and how they can be used to improve programming practices.
What is a lambda expression in the context of programming?
-A lambda expression is an anonymous function that allows you to treat code as data. It enables the parameterization of behavior, which can be passed around and used in different contexts.
Why is lambda being added to Java 8?
-Lambda is being added to Java 8 to allow for the parameterization of behavior, in addition to values and types. This feature enhances the flexibility and productivity of Java programming by enabling a different programming style.
What is a functional interface in Java?
-A functional interface in Java is an interface that contains exactly one abstract method. It is used to pass behavior as a method argument, which is a key feature enabled by lambda expressions.
How does lambda affect the way programs are written?
-Lambda allows for the parameterization of behavior, which can transform the way programs are written by enabling more flexible and expressive code. It reduces the need for boilerplate code and can simplify complex operations like filtering, mapping, and reducing collections.
What is the difference between expression lambdas and statement lambdas?
-Expression lambdas are lambdas that consist of a single expression, while statement lambdas are lambdas that consist of multiple statements enclosed in braces. Expression lambdas are typically used when a single value needs to be returned, whereas statement lambdas are used when no return value is expected.
What is the purpose of the 'stream' method in Java 8?
-The 'stream' method in Java 8 is used to create a stream of objects from a collection or an array. It serves as the starting point for a pipeline of operations that can be performed on the elements of the collection in a declarative manner.
How can lambda expressions be used to improve parallel programming in Java?
-Lambda expressions, combined with the new stream API, allow for easy parallelization of operations. By simply changing a sequential stream to a parallel stream, developers can leverage multi-threading to improve the performance of their programs without having to manage the threads manually.
What is the 'prime directive against non interference' in the context of lambda expressions?
-The 'prime directive against non interference' refers to the rule that lambda expressions used in parallel streams must not have side effects, must not interfere with the source of the objects, and must not have order dependencies. This ensures that parallel execution does not lead to unexpected behavior.
How can lambda expressions be used to simplify the handling of collections in Java?
-Lambda expressions can be used with the new stream API to perform complex operations on collections in a simplified manner. Operations like filtering, mapping, and reducing can be expressed in a concise and readable way, reducing the need for explicit loops and conditional statements.
Outlines
π Introduction to Lambda Expressions in Java
Stuart Marks introduces the concept of lambda expressions in Java, explaining them as anonymous functions that allow treating code as data. He contrasts this introductory presentation to more complex ones by Brian Goetz, focusing on the basics of lambda, its integration in Java 8, and its impact on programming style. Lambda expressions enable parameterizing behavior, which is a significant shift from Java 7's capabilities. The talk promises a practical exploration of lambda through an example program, demonstrating its application in everyday tasks and complex programming scenarios like parallel programming.
π Use Cases for Lambda: Filtering and Selecting Data
The paragraph delves into practical use cases of lambda expressions for filtering and selecting data based on certain criteria. It uses the example of a 'Person' object and a list of persons to illustrate how lambda can be used to select individuals based on attributes like age and sex. The discussion includes scenarios like selecting eligible drivers, voters, and individuals of legal drinking age, highlighting the flexibility of lambda for various programming tasks. It also touches on the problem of duplicated code and the solution of parameterizing values to streamline the process.
π Enhancing Flexibility with Parameterized Methods
This section discusses the enhancement of programming flexibility through parameterized methods using lambda expressions. It explains how to refactor methods to accept parameters that define the behavior of the method, such as age limits for different use cases. The paragraph also addresses the complexity introduced by meta-information and the challenges of value parameterization when every value is potentially legal. The solution presented is to use lambda expressions to parameterize behavior instead of values, leading to cleaner and more maintainable code.
π€ Functions and Functional Interfaces in Java
The speaker introduces the concept of functions in Java, distinguishing them from Java's traditional approach to method invocation. Functions are defined as pieces of computation that take parameters and return values. The paragraph explains the notation used to describe functions and provides examples of different kinds of functions. It also discusses 'functional interfaces' β interfaces with a single method, which are key to utilizing lambda expressions in Java. The concept of a 'predicate' is highlighted as a common case for functions that take an object and return a boolean.
π οΈ Practical Application of Lambda Expressions
The paragraph demonstrates the practical application of lambda expressions in Java by refactoring the robocalling example. It shows how to use lambda expressions to replace anonymous inner classes, making the code more concise and easier to understand. The use of lambda expressions allows the caller to parameterize behavior directly within the method call, which is more efficient than creating separate methods or classes for each behavior. The paragraph also emphasizes the importance of functional interfaces in enabling this feature of Java 8.
π Leveraging Lambda for Streamlined Code
This section explores how lambda expressions can be used to streamline code by eliminating boilerplate code associated with anonymous inner classes. It discusses the concept of 'expression lambdas' and 'statement lambdas,' showing how they can be used to create more expressive and flexible code. The paragraph also explains how lambda expressions are converted into instances of functional interfaces by the Java compiler, which performs type inference to determine the types of parameters.
π§ Refactoring Code with Functional Interfaces
The speaker continues to refactor the example code, introducing functional interfaces like 'Block' and 'Mapper' to further parameterize behavior. The paragraph explains how these interfaces can be used to create more generic and reusable code. It also discusses the concept of a 'pipeline' of operations, where a stream of objects is filtered, mapped, and then processed by a block of code. The paragraph emphasizes the flexibility and power of this approach, allowing for easy rearrangement and extension of code.
π Introducing Stream API and Parallel Processing
The paragraph introduces the Stream API in Java, which represents a stream of values and allows for the creation of a pipeline of operations that can be executed in parallel. It explains how to convert a list into a stream and how to apply filter, map, and for-each operations within a pipeline. The paragraph also discusses the ease of introducing parallelism into the pipeline by simply changing a stream call to a parallel stream, highlighting the simplicity of writing parallel programs with lambda expressions.
π Considerations for Parallel Programming
This section discusses the considerations that need to be taken into account when writing parallel programs using lambda expressions and the Stream API. It introduces the 'Prime Directive Against Non Interference,' which states that lambda expressions must not have side effects or dependencies on the order of execution. The paragraph also mentions the importance of using thread-safe data structures when writing parallel code and provides a recap of the new features introduced in Java 8 for functional programming and parallelism.
π Wrapping Up and Inviting Questions
The final paragraph wraps up the presentation by summarizing the key points about lambda expressions and the new APIs introduced in Java 8. It emphasizes the power and flexibility that lambda brings to Java, allowing for expressive and powerful programming, as well as the ease of writing parallel programs. The speaker also provides references for further information, including talks by Brian Goetz and resources related to the lambda project. The paragraph concludes by inviting questions from the audience.
Mindmap
Keywords
π‘Lambda
π‘Functional Interface
π‘Type Inference
π‘Anonymous Function
π‘Parameterization of Behavior
π‘Stream API
π‘Parallel Processing
π‘Behavior Parameterization
π‘Pipeline Operations
π‘Predicate
π‘Mapper
Highlights
Introduction to lambda in Java 8 as an anonymous function allowing code to be treated as data.
Lambda enables parameterization of behavior in addition to values and types, impacting program flexibility.
Java 8 introduces lambda as a programming language feature for higher productivity and a fluent programming style.
Lambda is not just a language change; it involves changes to the JVM and class libraries.
Demonstration of transforming a simple program using lambda to improve and adapt it for various use cases.
Explanation of how lambda can be used for everyday programming tasks as well as for complex, parallel programming.
Discussion on the evolution from value parameterization to behavior parameterization using lambda.
Introduction of functional interfaces as key components for lambda expressions in Java.
The concept of predicates as a special case of functional interfaces for boolean evaluations.
Rewriting existing code examples to utilize lambda expressions for more concise and readable code.
The importance of type inference in lambda expressions to maintain Java's statically typed nature.
Overview of the new Java APIs for streams and pipeline operations in conjunction with lambda.
How lambda expressions can be used to create parallel processing pipelines with ease.
The 'Prime Directive against Non Interference' when writing parallel programs with lambda to avoid side effects.
Introduction to the standard set of functional interfaces in Java.util.function for use with lambda.
Explanation of stream operations like filter, map, and forEach, and their use in creating expressive and flexible code.
The potential for using lambda expressions to simplify the writing of parallel programs and the considerations involved.
Recap of lambda's role in enabling powerful programming constructs and the new Java APIs that support them.
Invitation for the audience to try out the lambda features in the early access builds and report any bugs.
Final Q&A session addressing concerns about type inference, method call overhead, and thread pool management.
Transcripts
Browse More Related Video
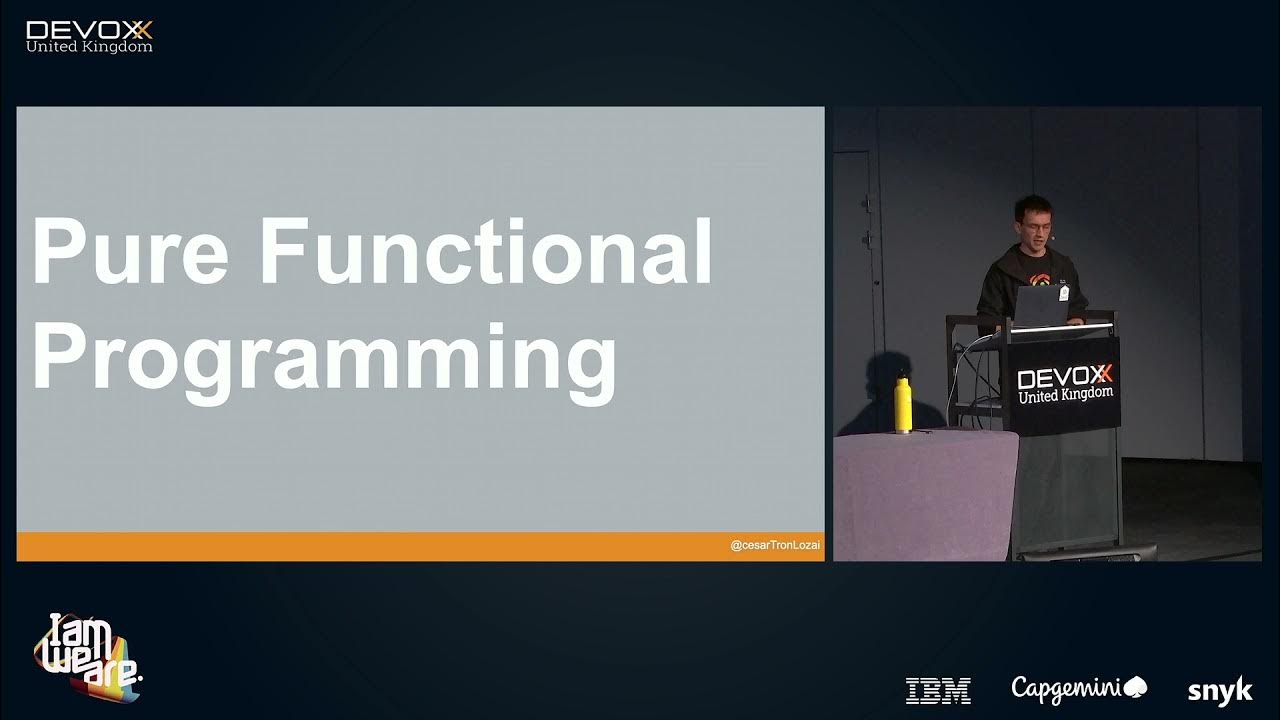
No Nonsense Monad & Functor - The foundation of Functional Programming by CΓ©sar Tron-Lozai

The Road to Lambda
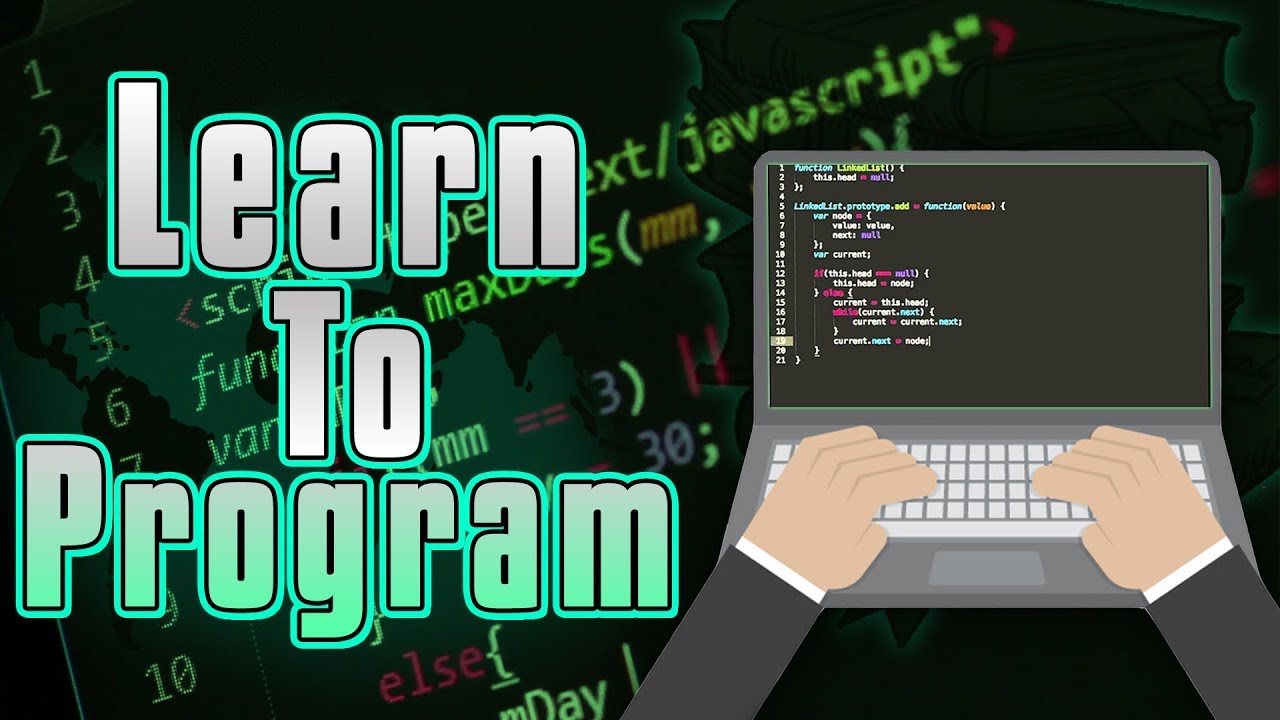
How To Learn Programming for BEGINNERS! (2022/2023)

Understanding parser combinators: a deep dive - Scott Wlaschin
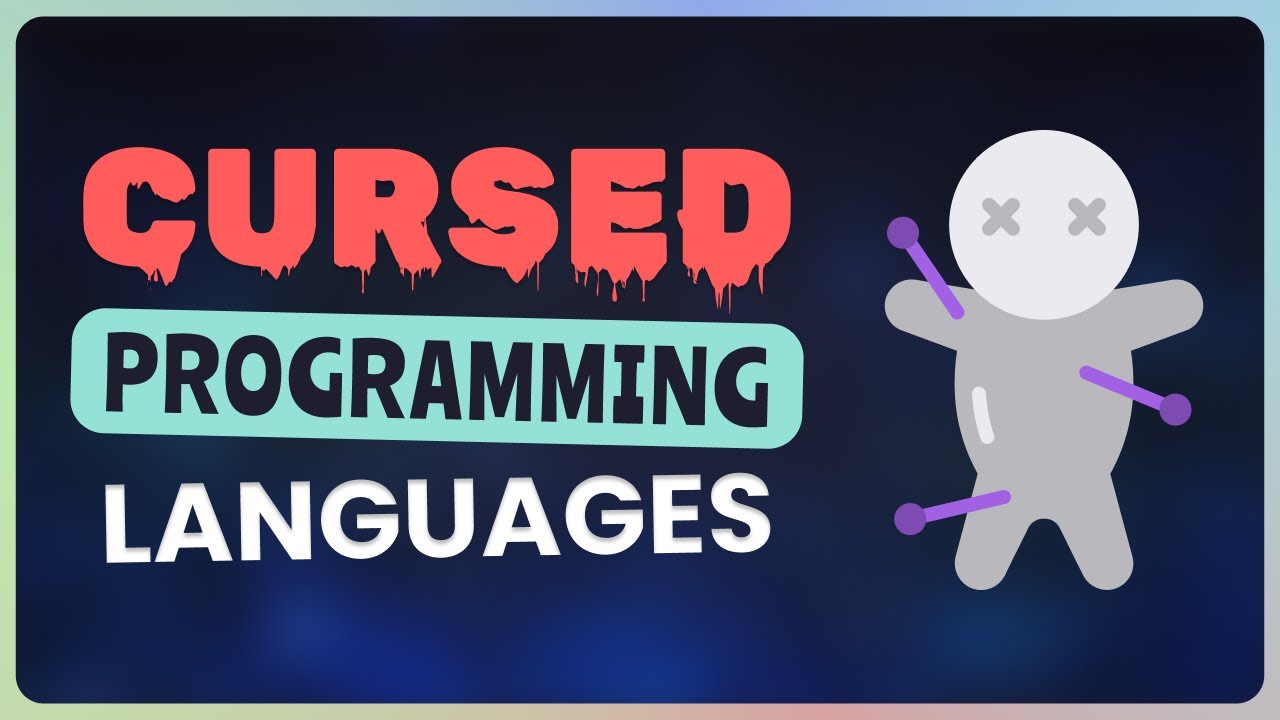
"Hello, World" in 5 CURSED languages that no one should use
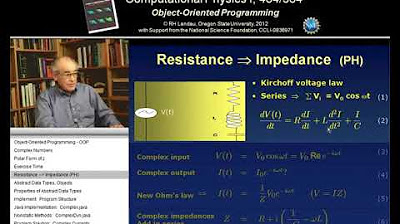
- Object Oriented Computing (OOP)
5.0 / 5 (0 votes)
Thanks for rating: