Master React In 2024 (Complete Guide)
TLDRThe video script introduces the fundamentals of JavaScript, emphasizing variables, functions, if statements, and loops as the core building blocks. It suggests that while classes are less commonly used, understanding their purpose is beneficial. The script then transitions into discussing React, highlighting its ease of setup and the use of hooks, reusable components, and props. It also touches on state management and the cross-platform capabilities of React, including its use for web and mobile applications through React Native and Next.js. The speaker encourages learning React over other frameworks due to its popularity and job market demand.
Takeaways
- ๐ Start with JavaScript basics: variables, functions, if statements, and loops are fundamental to understanding JavaScript.
- ๐ In React, the 'dot map' method is commonly used to render items and components multiple times, unlike traditional for loops.
- ๐ Building a basic webpage with JavaScript involves HTML for structure, CSS for styling, and JavaScript for interactivity.
- ๐ง JavaScript is used for responsive design, such as handling screen size changes and implementing interactive elements like hamburger menus.
- ๐ Learn React fundamentals, including hooks (useEffect, useReducer), and the concept of reusable components.
- ๐ Reusable components in React allow for efficient code by using props to change the component's appearance and functionality without repeating HTML.
- ๐ฏ Start with React before exploring other frameworks, as it is widely used and recommended for its ease of setup and community support.
- ๐ง Understand the modern practice of using functional components over classes in React, which simplifies the development process.
- ๐ Use React's hooks (like useState and useEffect) for state management and lifecycle control, which are essential for dynamic web applications.
- ๐ฑ Leverage React Native to apply the knowledge gained from building web applications to create cross-platform mobile apps.
- ๐ Explore Next.js for advanced React web application development, combining the power of React with server-side rendering and other advanced features.
Q & A
What are the basic fundamentals of JavaScript mentioned in the transcript?
-The basic fundamentals of JavaScript mentioned are variables, functions, if statements, and loops. Classes are noted as less essential for beginners.
What is the significance of the 'map' method in React?
-The 'map' method in React is used to render items, such as cards or components, multiple times. It's a key feature for handling lists and dynamic content in React applications.
How does the speaker describe the use of JavaScript in website development?
-The speaker describes using JavaScript for making websites interactive, such as handling screen size changes, implementing hamburger menus, and manipulating the Document Object Model (DOM).
Why does the speaker recommend React over other frameworks?
-The speaker recommends React because it is easy to set up, has a large community, is widely used, and allows for cross-platform development with React Native for mobile apps. They also mention the usefulness of React Hooks and reusable components.
What is the role of 'props' in React components?
-In React, 'props' (short for properties) are used to pass data from a parent component to a child component, making it possible to create dynamic and reusable components.
What is the difference between functional components and class components in React?
-Functional components are simply functions that return JSX, while class components are more complex and are part of the older practice. Modern React development favors functional components with hooks for state and lifecycle management.
How does the speaker suggest learning JavaScript and React?
-The speaker suggests learning by reading through documentation, starting with the basics, and then applying those concepts through building projects. They also recommend focusing on one framework (React) before exploring others.
What are some of the React-specific concepts mentioned in the transcript?
-Some React-specific concepts mentioned include React Hooks (like `useEffect`, `useMemo`), conditional rendering, state management, and the use of the 'map' method for listing and rendering dynamic content.
Why is it beneficial to learn React for web and mobile development?
-Learning React is beneficial because it allows for the creation of both web and mobile applications using a single set of skills. With React Native, developers can build cross-platform applications efficiently.
What is the speaker's opinion on the necessity of learning about classes in JavaScript?
-The speaker believes that learning about classes in JavaScript is not as crucial for beginners, as one can learn how JavaScript works and understand the purpose of classes without needing to dive deep into them initially.
What does the speaker suggest for someone feeling overwhelmed with learning JavaScript frameworks?
-The speaker suggests not to overwhelm oneself with learning multiple frameworks at once. Instead, focus on learning React first, as it is widely used and can be applied to both web and mobile development, making it a practical choice for beginners.
Outlines
๐ Introduction to JavaScript Fundamentals
This paragraph introduces the basic concepts of JavaScript, emphasizing the importance of understanding variables, functions, if statements, and loops. It suggests that while classes are part of the language, they are not as essential for beginners. The speaker also highlights the use of JavaScript in React, particularly with the dot map method for rendering components multiple times, and the limited use of traditional loops in favor of React's features.
๐จ Building with React: Hooks and Components
The speaker discusses the ease of setting up React and the utility of React hooks, such as useEffect and useMemo. It emphasizes the concept of reusable components in React, which allows for efficient code by avoiding duplicate HTML. The paragraph also touches on the use of props to pass dynamic data to components and the modern practice of using functional components over classes.
๐ง Creating Reusable Components in React
This section delves deeper into the creation of reusable components in React, demonstrating how to define a card component with props and use it multiple times in an application. It explains the flexibility of changing component properties without altering the underlying code, showcasing the power of props in React. The speaker also briefly mentions the importance of learning about JavaScript objects and DOM manipulation.
๐ The Power of React for Web and Mobile Development
The speaker advocates for learning React over vanilla JavaScript, noting the demand for React developers in the job market. It outlines the benefits of conditional rendering, the use of arrays and the map function, and state management in React. The paragraph also introduces React Native, highlighting the ability to use React for both web and mobile app development, and mentions Next.js as a tool for enhancing web applications.
Mindmap
Keywords
๐กJavaScript
๐กReact
๐กVariables
๐กFunctions
๐กIf Statements
๐กClasses
๐กLoops
๐กReact Hooks
๐กReusable Components
๐กProps
Highlights
JavaScript basics include variables, functions, if statements, and loops.
Classes in JavaScript are not as essential for beginners to learn initially.
React is a popular framework for JavaScript and is recommended for beginners over other frameworks.
In React, the map method is commonly used for rendering list items and components multiple times.
React hooks are a fundamental part of React, allowing for state management and function components.
Reusable components in React are a powerful feature, allowing for efficient code reuse without duplicating HTML.
Props in React are used to pass data to components, making them dynamic and reusable.
Functional components are preferred over class components in modern React practices.
React makes it easy to set up and use libraries like React Query for additional functionality.
TypeScript can be used with React for added type safety and structure.
Understanding objects and DOM manipulation is important for JavaScript developers.
Conditional rendering in React allows for dynamic content based on state or props.
Arrays and the map function are key concepts in both JavaScript and React.
State management is a crucial aspect of React applications, handled through hooks like useState and useReducer.
React Native extends React's capabilities to build cross-platform mobile applications.
Next.js is a framework that works with React to build server-rendered web applications.
Learning React can lead to job opportunities as it is in high demand for web development.
Transcripts
Browse More Related Video
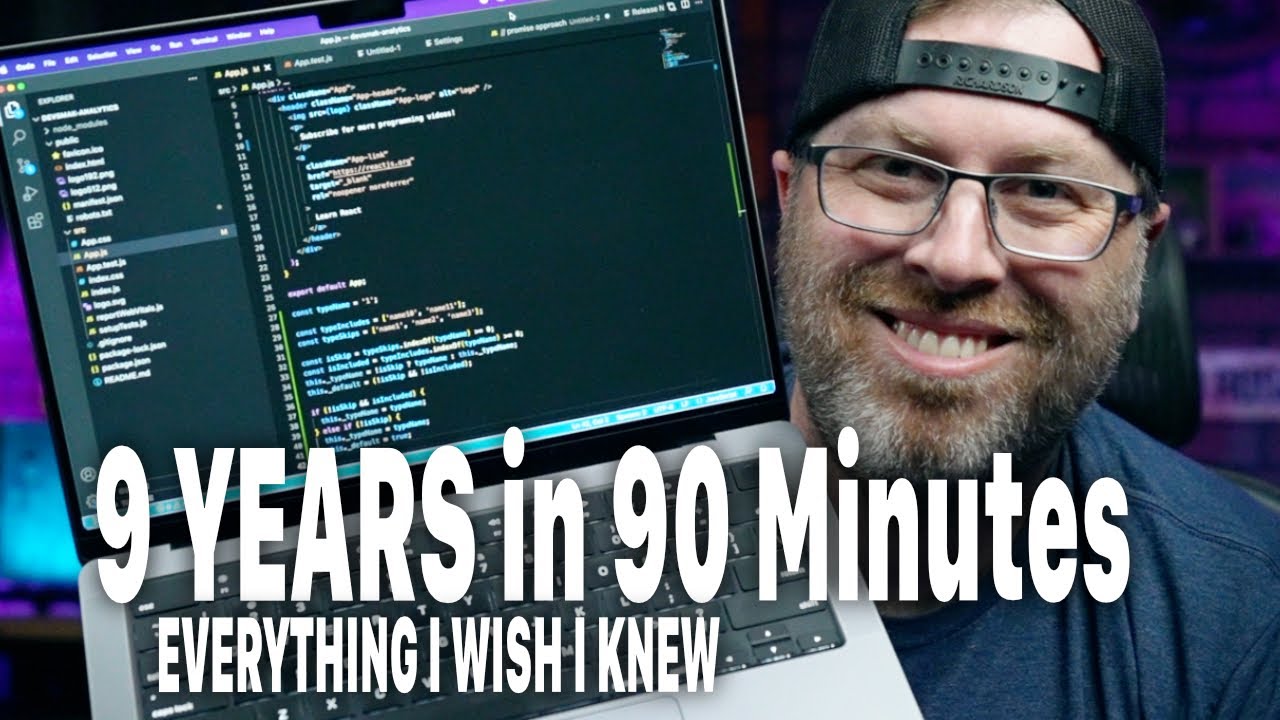
Learn Web Development And ACTUALLY Get A Job In 2023 | Ultimate Guide
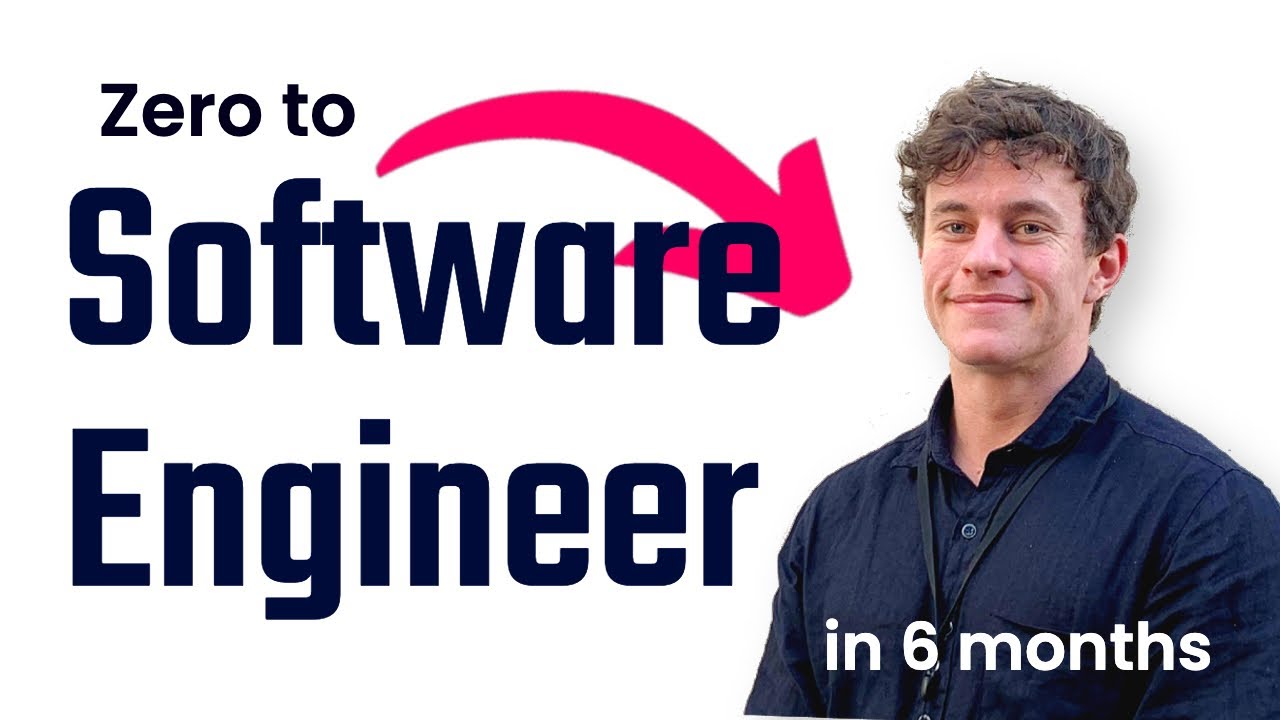
How I learned to Code in 6 MONTHS & Got a Job Offer (Self-Taught)
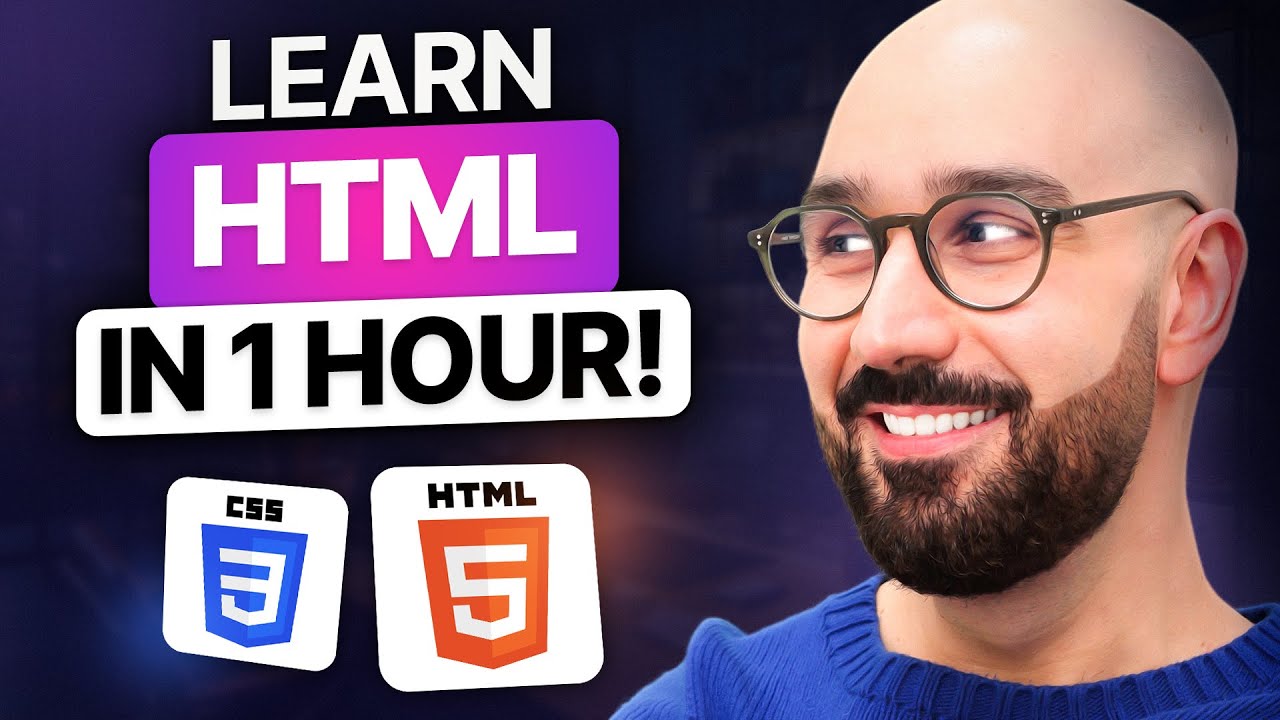
HTML Tutorial for Beginners: HTML Crash Course

Elements and atoms | Atoms, compounds, and ions | Chemistry | Khan Academy

FIRED For Using React?? | Prime Reacts

๐ฉโ๐ป Python for Beginners Tutorial
5.0 / 5 (0 votes)
Thanks for rating: