An Introduction to Procs, Lambdas and Closures in Ruby
TLDRThis video script offers an introductory exploration into Ruby's procs, lambdas, and closures. It explains the concept of blocks as nameless functions, the creation and usage of procs as anonymous functions that can be passed around, and the distinction between procs and lambdas, particularly in their parameter handling and return behavior. The script also delves into closures, demonstrating how they maintain references to local variables at the time of their definition, with examples to illustrate the concepts. The tutorial is designed to provide a foundational understanding, empowering viewers to explore further through deeper tutorials and practical application.
Takeaways
- π The video introduces the concepts of procs, lambdas, and closures in Ruby, providing a basic understanding of how they work and relate to each other.
- π Blocks in Ruby are nameless functions that can be passed around and executed in different contexts, often used in loops or iterators like the `each` method.
- π The `yield` keyword is used to invoke a block passed to a method, and `block_given?` can be used to check if a block was passed.
- π The `Proc` object is a named or anonymous function that can be represented as an object, passed around, and called like a method.
- π The `Proc.new` method can be used to create a proc, and if no block is explicitly passed, it will implicitly use the block from the current scope.
- π― Lambdas are similar to procs but enforce arity, meaning they require the exact number of arguments they expect, unlike procs which can accept any number.
- π The return behavior in lambdas and procs differs; a return in a lambda returns from the method it was called in, while a return in a proc returns from where it was defined.
- π Closures in Ruby are anonymous code blocks or functions that maintain references to local variables at the time of their definition, allowing them to access and modify those variables later.
- π Ruby closures are unique in that they keep a reference to the variables rather than storing their values, meaning the values can change before the proc is called, affecting the closure's behavior.
- π The video provides practical examples and demonstrations to illustrate the concepts, including creating custom methods that accept blocks and using procs and lambdas in different scenarios.
Q & A
What are the main topics covered in the video?
-The video covers an introduction to blocks, procs, lambdas, and closures in Ruby.
What is a block in Ruby?
-A block in Ruby is essentially a nameless function or a segment of code that can be passed around and executed in different contexts, often in loops or iterators.
How can you create a method in Ruby that accepts a block?
-You can create a method in Ruby that accepts a block by using the `yield` keyword within the method. This allows the method to execute the block passed to it.
What is the difference between a proc and a block?
-A proc is an object that represents a block of code that can be passed around and executed later. Blocks are anonymous functions that are typically used within methods and can be passed to other methods using `yield` or converted into a proc using the `&` operator.
How can you check if a block was given to a method?
-You can check if a block was given to a method using the `block_given?` method in Ruby.
What is the purpose of the `Proc.new` method?
-The `Proc.new` method is used to create a new proc object. It can take a block of code as an argument, or if called without a block, it will implicitly use the block from the current scope.
How does the `lambda` differ from a `proc`?
-A `lambda` is similar to a `proc`, but it enforces arity, meaning it expects the exact number of arguments it was defined with. Additionally, a `lambda` will return from the context it is called from, unlike a `proc` which returns from where it was defined.
What is a closure in Ruby?
-A closure in Ruby is a proc object that maintains references to local variables that were present and being used at the time of its definition. This allows the proc to access and modify those variables even after they have gone out of scope.
How can you dynamically define function behavior using closures?
-You can dynamically define function behavior using closures by creating a method that generates a lambda with a specific behavior based on the arguments passed to the method. The lambda captures the local variables in its closure, allowing it to use them in its execution.
What is the significance of the `source_location` method in Ruby procs?
-The `source_location` method in Ruby procs provides information about the file and line number where the proc was defined. This can be useful for debugging or creating testing libraries that need to reference the source code of the proc.
Outlines
π Introduction to Ruby's Procs, Lambdas, and Closures
The script begins with an introduction to Ruby's programming constructs: procs, lambdas, and closures. The instructor provides a brief overview of these concepts and mentions that the tutorial will be a part of a larger course called 'Ruby Reloaded.' The focus is on the basic understanding of blocks in Ruby, which are nameless functions that can be passed around and executed in different contexts. The explanation includes practical examples, such as using the 'each' method on arrays and creating custom methods that accept and utilize blocks.
π Exploring Blocks and Iterators in Ruby
This paragraph delves deeper into blocks as iterators in Ruby, demonstrating how they can be used with methods like 'each' and how to create custom iterator methods. The script introduces the 'yield' keyword, which is used to execute a block within a method. It also explains the 'block_given?' method to check for the presence of a block. The instructor provides an example of 'random_each', a custom method that iterates over an array in a random order, showcasing the power and flexibility of blocks in Ruby.
π Understanding Procs and Their Creation
The script moves on to discuss procs, which are essentially anonymous functions or blocks of code that can be represented as objects in Ruby. It explains how to create procs using the 'Proc.new' method and the shorthand 'proc'. The paragraph highlights the difference between passing a block to a method and passing a proc as an object, with examples demonstrating how procs can be stored in variables and called like functions. The instructor also touches on the ability to pass multiple procs to methods due to their object nature.
π Advanced Proc Usage and Ruby Tricks
This section covers more advanced uses of procs, including the implicit creation of a proc from the current scope using 'Proc.new' without explicitly passing a block. It also discusses different ways to call or run a proc, such as using the 'call' method, dot notation, square brackets, and the case equality operator. The script includes a clever trick using procs within case statements, which allows for abstracting conditional logic into proc objects for dynamic execution.
π¦ Diving into Lambdas and Their Distinct Behavior
The script introduces lambdas as a type of proc object with specific behaviors, such as arity enforcement, where lambdas require the exact number of parameters they are defined with. It contrasts this with procs, which default to nil for missing arguments. The paragraph also explains the difference in return semantics between lambdas and procs, where a return within a proc attempts to exit the context in which it was defined, potentially causing an error, whereas a return within a lambda exits the lambda itself.
π The Concept of Closures in Ruby
This paragraph explains closures in Ruby, which are similar to procs but maintain references to local variables at the time of their definition. The script provides examples of closures in action, showing how they capture the state of local variables even if their values change after the closure is created. It also demonstrates how closures can be used to dynamically generate behavior, such as creating multiplier functions on the fly.
π Conclusion and Further Exploration
The final paragraph wraps up the introduction to blocks, procs, and closures in Ruby. It emphasizes the importance of understanding these concepts to navigate more complex Ruby code and encourages viewers to explore the Ruby documentation for further insights. The instructor offers to create more advanced videos on request and invites feedback from the audience, highlighting the aim to provide a foundational understanding before delving into more obscure cases.
Mindmap
Keywords
π‘Procs
π‘Lambdas
π‘Closures
π‘Blocks
π‘Yield
π‘Block_given?
π‘Case Equality Operator
π‘Anonymous Functions
π‘Method Definitions
π‘Monkey Patching
π‘Return Semantics
Highlights
Introduction to procs, lambdas, and closures in Ruby, providing basic understanding and practical examples.
Explanation of blocks in Ruby as nameless functions that can be passed around and used in loops and iterations.
Demonstration of using the 'each' method and blocks to iterate over arrays in Ruby.
The concept of creating custom methods in Ruby that accept and utilize blocks.
Using 'yield' to pass control to a block within the current method context.
Error handling with 'block_given?' to check for the presence of a block in a method.
Creating a 'random_each' method to iterate over arrays in random order using blocks.
The distinction between procs and blocks, and how to pass multiple procs as method parameters.
Creating and using proc objects with 'Proc.new' and the implicit capture of the current block.
Different ways to call or run a block in Ruby, including 'call', '[]', and '=== operators.
Using procs in case statements for abstracting conditional logic.
Differences between lambdas and procs, especially in terms of arity and parameter enforcement.
Behavior of 'return' within procs and lambdas, and its impact on the surrounding method context.
Recommendation to explore the Ruby documentation for proc class and its methods.
Introduction to closures in Ruby, which maintain references to local variables at the time of their definition.
Demonstration of closures capturing local variable changes and reflecting them when called.
Creating dynamic function behavior using closures to encapsulate variable states.
Discussion on the unique behavior of Ruby's closures compared to other languages regarding variable capture.
Transcripts
Browse More Related Video

Calculus 3 Lecture 15.1: INTRODUCTION to Vector Fields (and what makes them Conservative)
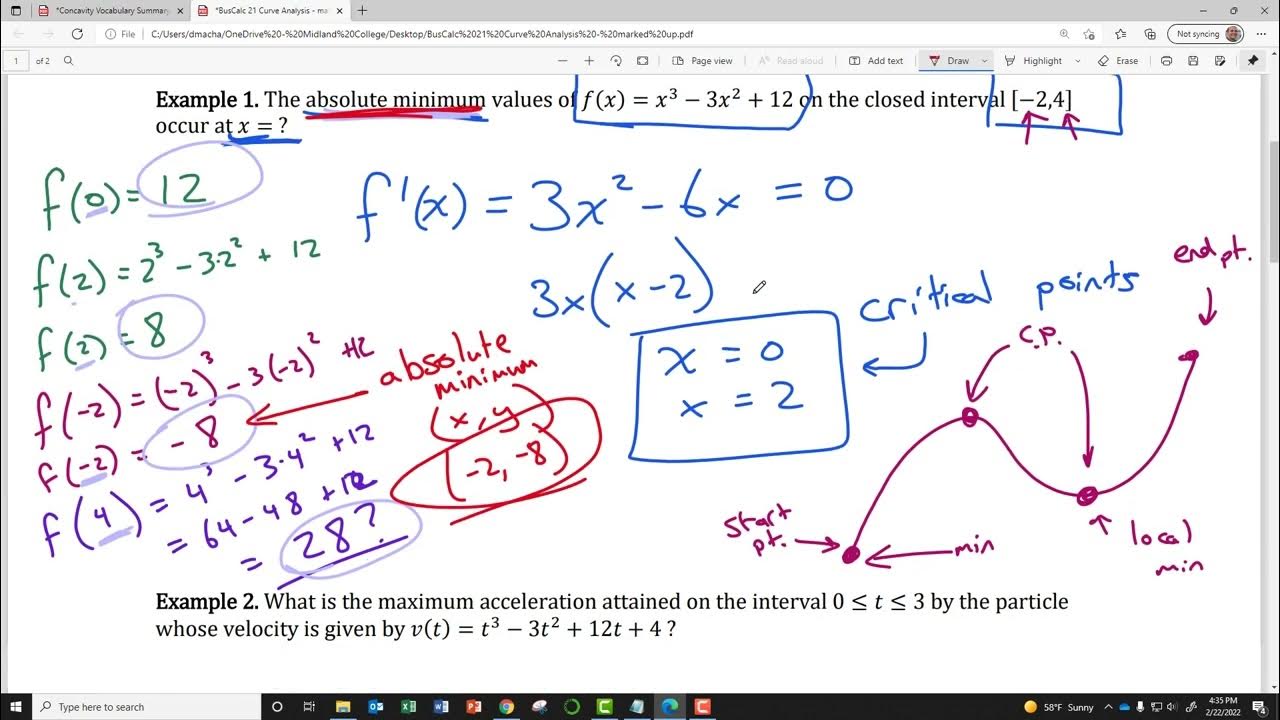
BusCalc 21 Curve Analysis
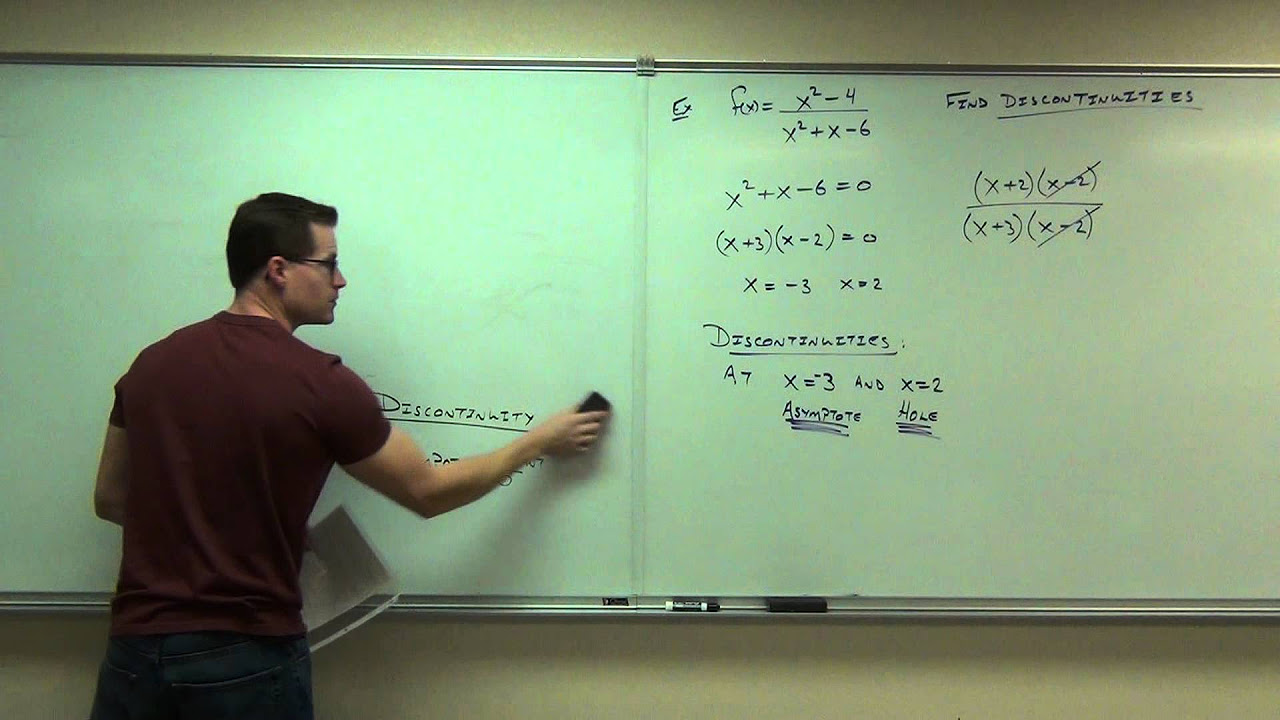
Calculus 1 Lecture 1.4: Continuity of Functions
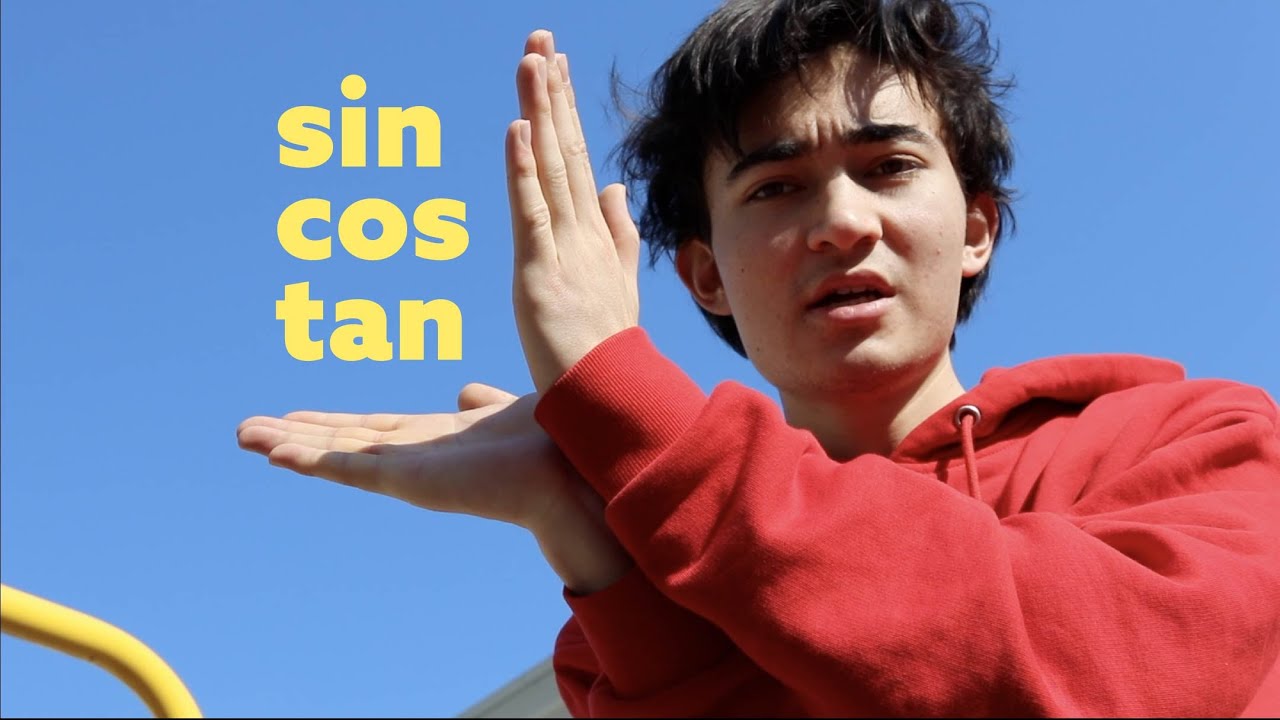
What does Sin, Cos, Tan actually mean? Trigonometry explained for Beginners!

How Derivatives Arise from Limits β Topic 50 of Machine Learning Foundations

Calculus - Lesson 6 | What are Functions? | Don't Memorise
5.0 / 5 (0 votes)
Thanks for rating: