Michael Caisse: Lambda Functions
TLDRThe transcript captures a detailed session on C++ lambda expressions, delving into their syntax, usage, and comparison with functors. It covers capture clauses, return types, and scope, highlighting practical examples and common pitfalls. The speaker discusses the versatility of lambdas in replacing traditional function objects and their potential performance implications. The session also touches on the evolving role of libraries like Boost in light of lambda integration and the importance of reevaluating standard algorithms for lambda compatibility.
Takeaways
- π Lambda expressions are powerful tools in C++ for creating anonymous functions that can capture and manipulate variables within their scope.
- π The speaker emphasizes the importance of understanding lambda expressions, especially in the context of the C++ standard library and algorithms.
- π§ Lambdas can simplify code by reducing the need for named functions or functors, allowing for more concise and readable code in certain scenarios.
- π― The session aims to demystify lambda expressions and provide practical insights into how they can be utilized effectively in everyday programming tasks.
- π οΈ The speaker discusses the syntax and components of lambda expressions, including the capture clause, parameters, and the return type.
- π Lambda expressions can be used with standard algorithms to perform operations on containers, offering a more streamlined approach compared to traditional functors.
- π The script highlights the need for good code style when demonstrating concepts, even though it might occasionally sacrifice strict adherence to best practices for clarity.
- π€ The use of lambda expressions can lead to more maintainable and flexible code, especially when dealing with complex operations that would otherwise require extensive setup.
- π The discussion includes common pitfalls and misconceptions about lambda expressions, such as their scope, capture behavior, and interaction with other language features.
- π The session also touches on performance considerations, suggesting that lambda expressions may offer performance benefits in certain cases due to better optimization by compilers.
- π The impact of lambda expressions on the role of existing libraries like Boost is considered, with the potential for lambdas to simplify and replace some uses of these libraries.
Q & A
What is the main topic of the session described in the transcript?
-The main topic of the session is the use of lambda expressions in C++ and how they can simplify library usage and code readability.
Why might someone find lambda expressions intimidating at first?
-Some might find lambda expressions intimidating because they involve more complex language features and can be used in group work or on language committees where issues may arise during implementation.
What is the purpose of the 'capture' in a lambda expression?
-The 'capture' in a lambda expression is used to store values or references from the surrounding context that the lambda needs to access when it is executed.
What is the difference between a functor and a lambda expression?
-A functor is an object that can be called like a function and has an 'operator()' method, whereas a lambda expression is an anonymous function that can be defined inline and has a more concise syntax.
Why might the speaker prefer to use a lambda expression over a functor in certain situations?
-The speaker might prefer a lambda expression because it can be more concise, easier to write, and can capture variables from the surrounding scope without needing to explicitly define them as with a functor.
What is the significance of the 'mutable' keyword in the context of lambda expressions?
-The 'mutable' keyword allows the lambda expression to modify variables that it has captured by value, enabling changes to the state of the captured variables.
What is the role of 'std::function' in the context of lambda expressions?
-'std::function' is a polymorphic wrapper that can hold any callable object, such as a lambda expression, function pointer, or functor, and allows it to be called as if it were a normal function.
What is the 'Y combinator' mentioned in the transcript, and how is it related to lambda expressions?
-The 'Y combinator' is a functional programming concept that allows for the creation of recursive functions. In the context of lambda expressions, it is used to create a lambda that calls itself recursively.
What is the 'closure object' mentioned in the transcript?
-A 'closure object' is an unnamed object created when a lambda expression is evaluated. It behaves like a function object and can be called with parameters, similar to a functor.
How can lambda expressions be used to improve the locality of code?
-Lambda expressions can be used to write small, inline functions where they are called, improving locality by reducing the need for separate function definitions and improving readability.
What are some potential performance considerations when using lambda expressions compared to traditional loops?
-In theory, there should be no performance difference between well-optimized lambda expressions and traditional loops. However, in practice, compilers may have better optimization strategies for traditional loops, and lambdas may introduce additional overhead due to their more complex nature.
Outlines
π Introduction to Lambda Expressions
The speaker begins by introducing the topic of lambda expressions, discussing their utility in simplifying code and their frequent use in standard libraries. They express some initial apprehension due to ongoing language committee work, which might affect the discussion. The speaker also mentions their intention to demonstrate the use of lambda expressions in their own coding practices, with a focus on practical application rather than theoretical aspects.
π Understanding Lambda Expressions and Functors
This paragraph delves into the comparison between functors and lambda expressions, highlighting their similarities and differences. The speaker discusses the structure of lambda expressions, including the capture clause, parameters, and return types. They also provide examples of how lambda expressions can be used in place of functors to create more concise and readable code, while also pointing out potential issues with using lambda expressions, such as increased compile time and the need for careful management of state.
π Deep Dive into Lambda Expression Components
The speaker provides an in-depth look at the components of lambda expressions, explaining the syntax and semantics of each part. They discuss the lambda introducer, capture clause, and the declaration within the lambda expression. The explanation includes how a lambda expression is converted into a closure object and how this object behaves like a function object. Practical examples are given to illustrate the use of lambda expressions in various scenarios, including passing parameters by value and reference.
π Exploring Capture Mechanisms in Lambdas
This section focuses on the capture mechanism in lambda expressions, illustrating how to capture variables by value or by reference within the lambda's scope. The speaker provides examples of capturing different combinations of variables and the implications of each method. They also discuss the use of the 'mutable' keyword, which allows for modification of captured variables within the lambda expression.
π Lambda Expressions and Return Types
The speaker discusses the rules governing the return types of lambda expressions. They explain how the return type is determined when it is omitted and the conditions under which a lambda can be converted into a function pointer. The paragraph also touches on the use of 'auto' for type inference and the importance of matching the return type of all expressions within the lambda for successful type deduction.
π Capturing by Value vs. Reference
In this segment, the speaker explores the nuances of capturing by value versus by reference in lambda expressions. They provide examples to demonstrate the behavior of captured variables and how they can be modified or remain constant within the lambda's scope. The discussion also includes the implications of capturing the same variable in different ways within nested lambda expressions.
π― Scope and Lifetime of Captured Variables
The speaker discusses the scope and lifetime of variables captured in lambda expressions. They explain the rules for capturing variables, including the restrictions on redefining captured variables within the lambda's body. The paragraph also addresses the issue of capturing static variables and the lifetime of objects within the lambda's scope, emphasizing the importance of understanding the scope to avoid undefined behavior.
π Practical Applications of Lambda Expressions
This section showcases practical applications of lambda expressions, including their use in algorithms and callbacks. The speaker provides examples of how lambda expressions can be used to create concise and readable code, particularly in scenarios involving complex operations on container elements. They also discuss the advantages of using lambda expressions over traditional functors in terms of locality and ease of use.
π Conclusion and Further Exploration
In the concluding segment, the speaker summarizes the key points discussed in the presentation and encourages further exploration of lambda expressions. They highlight the versatility and power of lambda expressions in modern C++ programming and suggest that attendees revisit standard algorithms and other areas where lambda expressions could be applied effectively.
Mindmap
Keywords
π‘Lambda Expressions
π‘Functors
π‘Capture Clause
π‘Closure Object
π‘Return Type
π‘Mutable
π‘Function Objects
π‘Algorithms
π‘Runtime Policies
π‘Locality of Expression
π‘Performance
Highlights
Discussion on simplifying libraries with lambda expressions and their frequent use.
Lambda expressions can be intimidating due to their complexity and role in group work and language committees.
The use of lambda expressions in client's codebase to streamline operations and improve readability.
Comparison between functors and lambda expressions, highlighting their similarities and differences.
Explanation of lambda expression components, including the capture clause and parameter list.
Demonstration of how lambda expressions can be used to create compact and efficient code.
The importance of understanding lambda expression scope and how it affects variable capture.
Different capture defaults in lambda expressions, such as capturing by value or by reference.
The impact of mutable keyword in lambda expressions and its role in modifying captured variables.
How lambda expressions handle return types and the rules governing them.
Practical examples of lambda expressions in algorithms and their optimization potential.
The role of lambda expressions in callbacks and runtime policies, enhancing flexibility.
Comparison of lambda expressions with traditional functors in terms of performance and use cases.
The influence of lambda expressions on the design and utility of existing libraries.
Discussion on the future of lambda expressions in C++ and their potential for parallelism.
Final thoughts on the versatility and practical applications of lambda expressions in modern C++ development.
Transcripts
Browse More Related Video
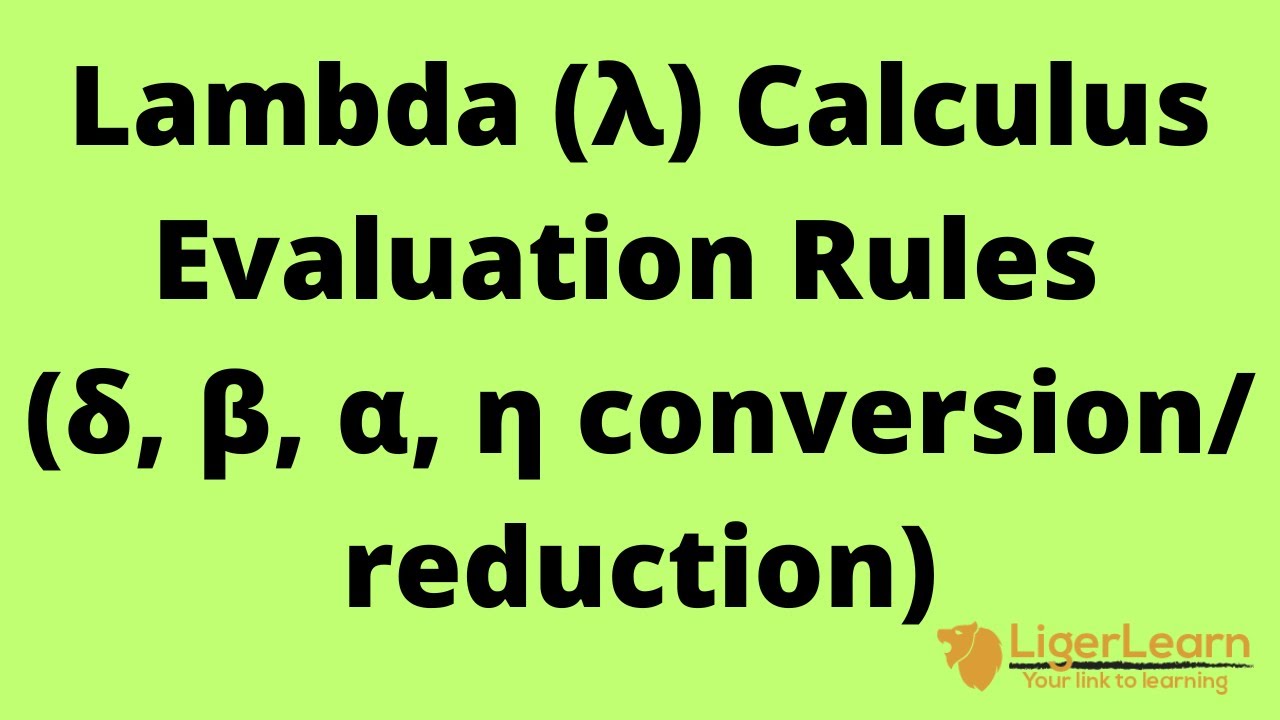
Lambda (Ξ») calculus evaluation rules (Ξ΄, Ξ², Ξ±, Ξ· conversion/reduction)
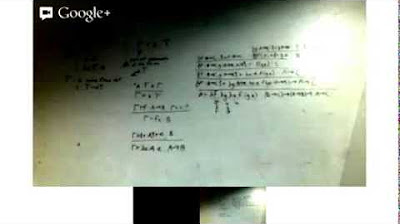
Meeting #5: Lambda Calculus Workshop

Martin Hyland: "Algebra & Diagrams in the Lambda Calculus"
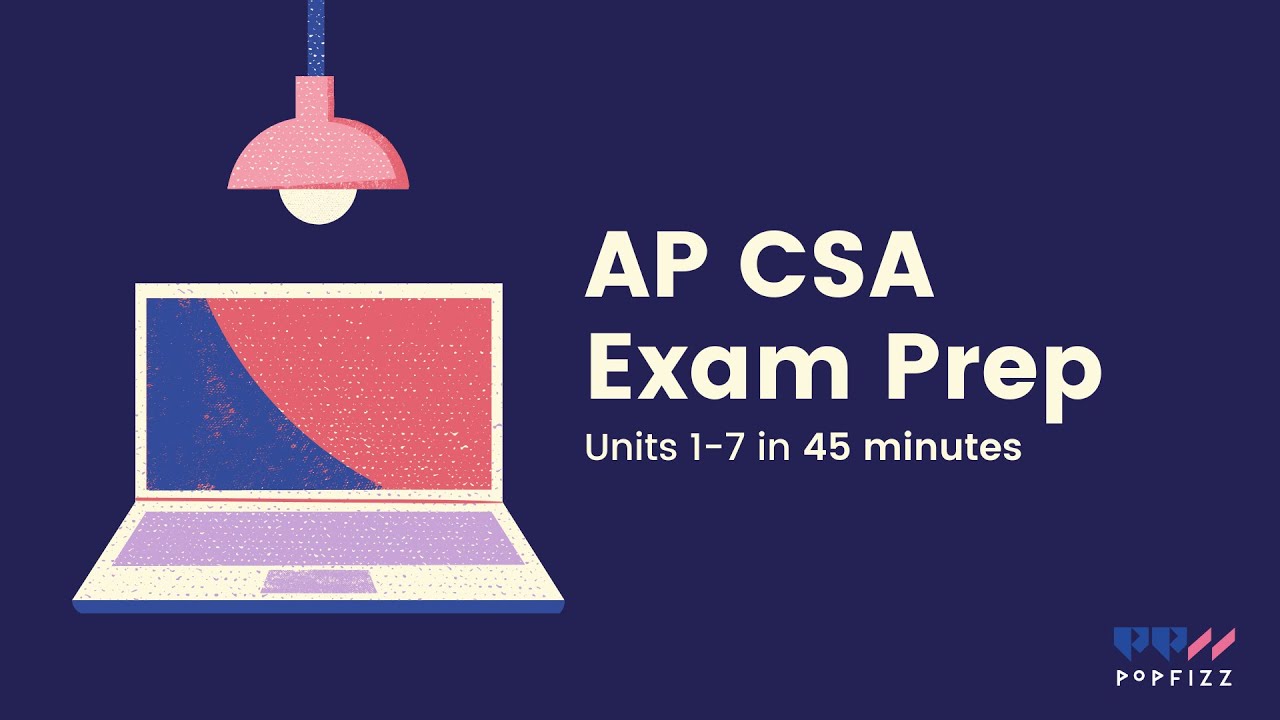
AP CSA Review in 45 minutes (Units 1-7)
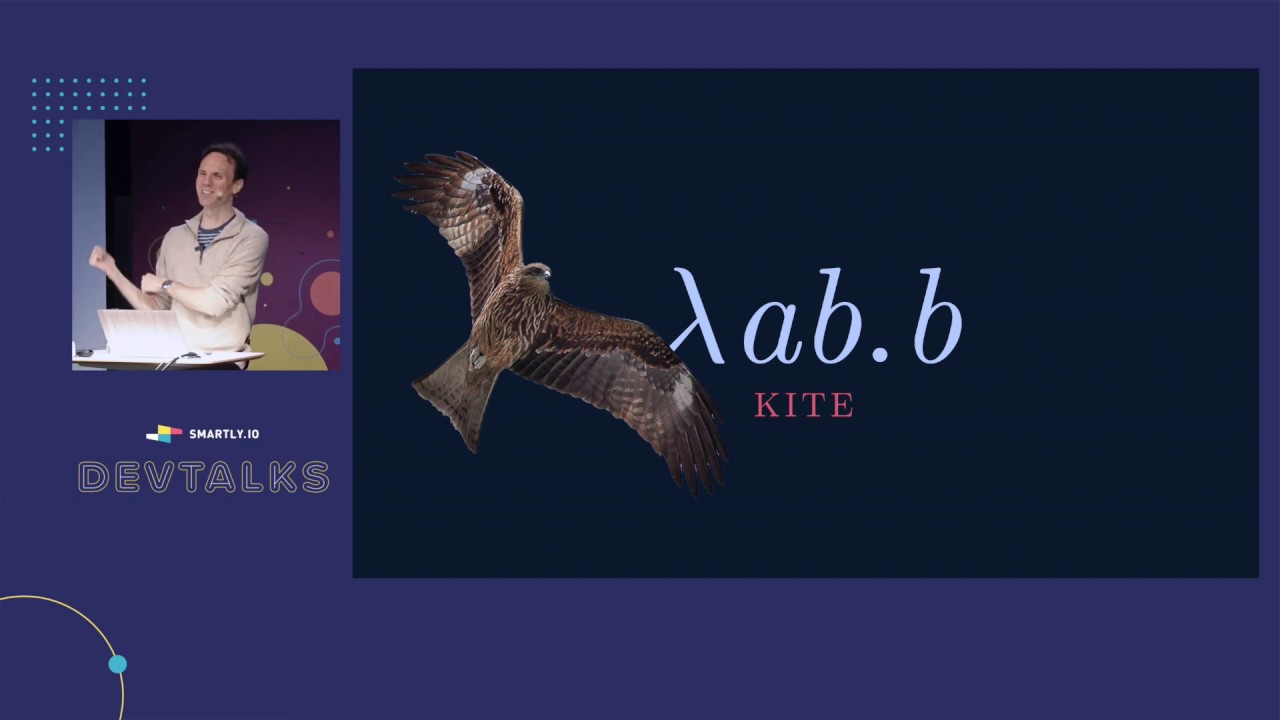
A Flock of Functions: Lambda Calculus and Combinatory Logic in JavaScript | Gabriel Lebec @ DevTalks
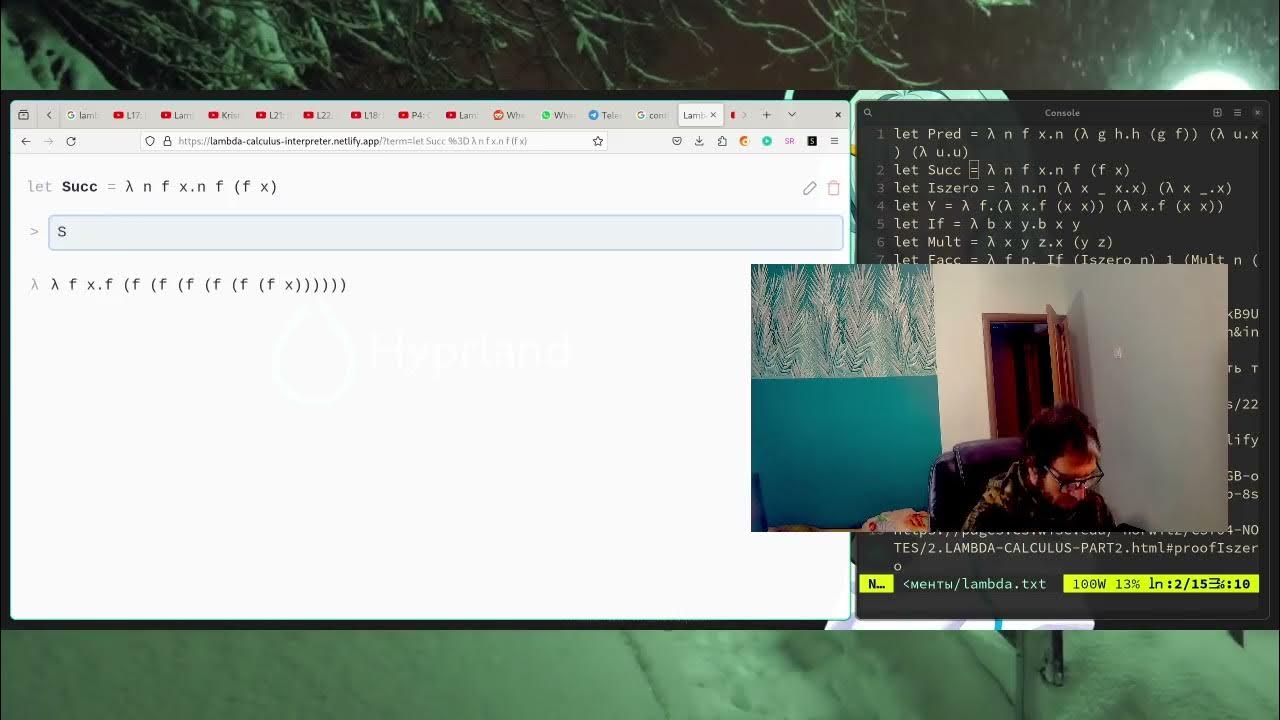
Lambda Calculus Calculator
5.0 / 5 (0 votes)
Thanks for rating: