Variables in Java ✘【12 minutes】
TLDRThis video tutorial offers an engaging introduction to variables in Java, explaining the concept as placeholders for values similar to algebraic variables. It covers various data types, including boolean, byte, short, integer, long, float, double, char, and string, detailing their storage capacity and usage. The presenter teaches how to declare, assign, and initialize variables, emphasizing the distinction between primitive and reference data types. With examples and clear explanations, the video simplifies complex programming concepts, making them accessible to beginners.
Takeaways
- 📌 Variables in Java are placeholders for values and behave like the values they contain, similar to algebraic variables.
- 🔢 Java supports various data types including boolean, byte, short, int, long, float, double, char, and string, each with specific use cases and memory sizes.
- 👉 Boolean variables hold true or false values and require one bit of memory, making them suitable for binary decisions like a light switch program.
- 🔢 The size and range of numeric data types increase from byte (-128 to 127), to short (-32,000 to 32,000), to int (just under 2 billion to just over 2 billion), to long (just under -9 quintillion to just over 9 quintillion).
- 📉 Float and double data types are used for fractional numbers, with float having less precision (up to 6-7 digits) and double offering more precision (up to 15 digits).
- 📝 When assigning values to float variables, the value must be followed by an 'f', whereas for double variables, no suffix is needed.
- 📖 The char data type uses two bytes of memory to store a single character, letter, or ASCII value, and requires single quotes for assignment.
- 📝 Strings are reference data types that can store sequences of characters, such as words or sentences, and are created with double quotes.
- 💡 Primitive data types (boolean, byte, short, int, long, float, double, char) store data directly and use less memory, while reference data types (like string) store an address and use more memory.
- 🛠️ Variables are created in Java by declaring the data type, assigning a value, or initializing with a value in one step.
- 🖥️ Demonstrating variable usage includes printing to the console, where variables behave as their contained values, and string concatenation can be performed with variables.
Q & A
What is a variable in Java?
-A variable in Java is a placeholder for a value and it behaves as the value that it contains. It can store different types of data such as numbers, words, sentences, and boolean values.
Can you explain the concept of data types in Java?
-In Java, there are eight primitive data types (boolean, byte, short, int, long, float, double, char) and a special reference data type called String. Each data type has a specific size and purpose, determining what kind of values can be stored within variables of that type.
What are the two values that a boolean data type can hold?
-A boolean data type can hold only two values: true or false.
What is the size of a boolean data type in Java?
-A boolean data type in Java has a size of one bit.
How many primitive data types are there in Java and what are they?
-There are eight primitive data types in Java: boolean, byte, short, int, long, float, double, and char.
What is the difference between a float and a double in Java?
-A float in Java can store a fractional number with up to six to seven digits of precision, while a double has more precision and can store a fractional number up to 15 digits.
How should you assign a value to a float variable in Java?
-When assigning a value to a float variable in Java, you need to follow the value with the letter 'f'.
What is the purpose of the char data type in Java?
-The char data type in Java is used to store a single character, letter, or ASCII value using two bytes of memory.
What is the difference between primitive and reference data types in Java?
-Primitive data types in Java (like boolean, byte, etc.) store data directly and use less memory, while reference data types (like String) store an address and can hold more complex data structures, using more memory.
How do you create a variable in Java?
-To create a variable in Java, you first declare the data type and then the variable name, followed by a semicolon. You can then assign a value to the variable, or combine declaration and assignment in one step called initialization.
Can you provide an example of how to initialize an integer variable in Java?
-An example of initializing an integer variable in Java would be: `int x = 123;`
What is the largest number that can be stored in an integer variable in Java?
-An integer variable in Java can store numbers up to just under 2 billion.
How do you handle extremely large numbers in Java?
-For extremely large numbers, you would use the long data type in Java, and you need to follow the number with a capital 'L' when assigning it to a long variable.
What is the difference between a char and a String in Java?
-A char in Java is a primitive data type that stores a single character, while a String is a reference data type that stores a sequence of characters, such as words or sentences.
How do you print the value of a variable to the console in Java?
-To print the value of a variable to the console in Java, you use the `System.out.println()` method followed by the variable name without quotes.
Outlines
📚 Introduction to Java Variables
This paragraph introduces the concept of variables in Java. It compares variables to placeholders for values, similar to algebraic variables, but with the added capability to store various data types beyond just numbers. The video aims to teach viewers about the different data types available in Java, such as boolean, byte, short, int, long, float, double, char, and string. It emphasizes the importance of declaring the data type when creating a variable and provides a basic overview of each data type, including the memory size and range of values they can hold. The paragraph also touches on the distinction between primitive and reference data types, setting the stage for a deeper dive into variables in subsequent parts of the video.
🔢 Understanding Data Types and Variable Creation
This paragraph delves deeper into the specifics of Java's data types, explaining the range and memory usage of numeric types like byte, short, int, long, float, and double. It clarifies that while bytes and shorts are less commonly used due to their limited range, ints are more convenient for beginners. The explanation continues with how to declare, assign, and initialize variables in Java. The paragraph provides examples of creating integer and long variables, and demonstrates how to print their values to the console. It also covers the use of string concatenation to combine text and variables in print statements, offering practical examples to illustrate the concepts.
📝 Advanced Data Types and Variable Manipulation
The final paragraph of the script discusses advanced data types such as floats, doubles, booleans, chars, and strings. It explains the use of floats for fractional numbers and the convention of appending 'f' to float literals. Doubles are highlighted for their higher precision and the absence of the need for an 'f' suffix. Booleans are introduced as variables that can only hold true or false values. Chars are described as variables that store a single character, with an example given using the '@' symbol. Strings are explained as reference data types that can store sequences of characters, and the paragraph demonstrates how to declare, assign, and manipulate string variables, including string concatenation. The video concludes by offering the code in the comments for viewers to follow along and encourages viewers to like, comment, and subscribe for more content.
Mindmap
Keywords
💡Variable
💡Data Type
💡Boolean
💡Byte
💡Short
💡Integer
💡Long
💡Float
💡Double
💡Char
💡String
💡Primitive vs. Reference Data Types
💡Declaration
💡Assignment
💡Initialization
💡String Concatenation
Highlights
Introduction to variables in Java programming.
A variable is a placeholder for a value in programming, similar to algebraic variables.
Variables in Java can store different data types, not just numbers.
Explanation of Java's eight primitive data types and the special reference data type called String.
Boolean data type can only hold true or false values.
Byte data type can store integer numbers between -128 to 127.
Short data type uses two bytes and can hold larger numbers than a byte.
Integer data type uses 4 bytes and can store numbers up to just over 2 billion.
Long data type uses 8 bytes and can store extremely large numbers.
Float data type can store fractional numbers with less precision than double.
Double data type offers more precision and can store up to 15 digits after the decimal point.
Difference between float and double when assigning values, with the need for an 'f' for floats.
Char data type uses two bytes and stores a single character or ASCII value.
String data type is a reference type that stores sequences of characters.
Explanation of the difference between primitive and reference data types.
Process of creating a variable in Java: declaration, assignment, and initialization.
Demonstration of creating and using integer, long, float, double, boolean, char, and string variables.
Using variables in print statements and string concatenation.
Storing large numbers with the long data type and the convention of appending 'L'.
Using boolean variables to store true or false values.
Creating and displaying character variables with the char data type.
Working with string variables and performing string concatenation.
Conclusion and invitation to get a copy of the code from the comments section.
Transcripts
Browse More Related Video
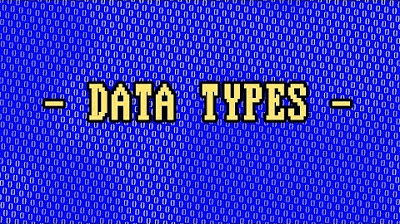
What Are Data Types?
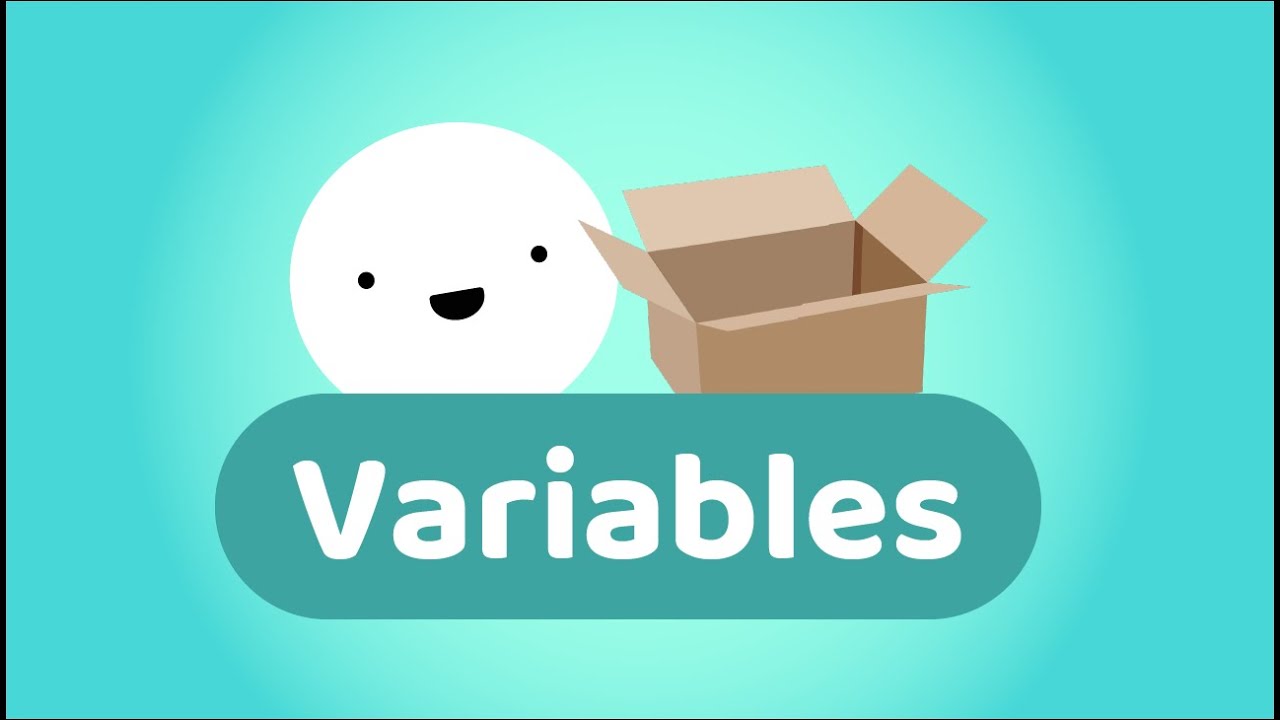
Coding Basics: Variables | Programming for Beginners |
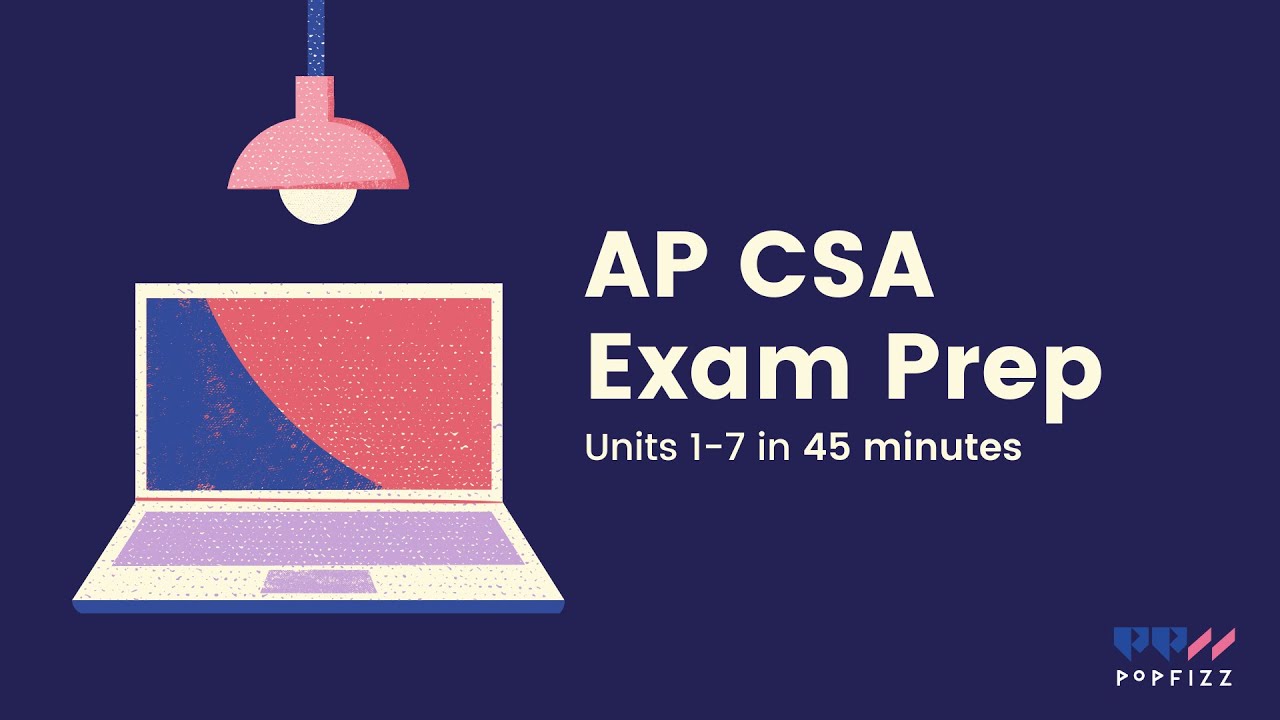
AP CSA Review in 45 minutes (Units 1-7)

Descriptive statistics and data visualisation. An introduction to statistics and working with data
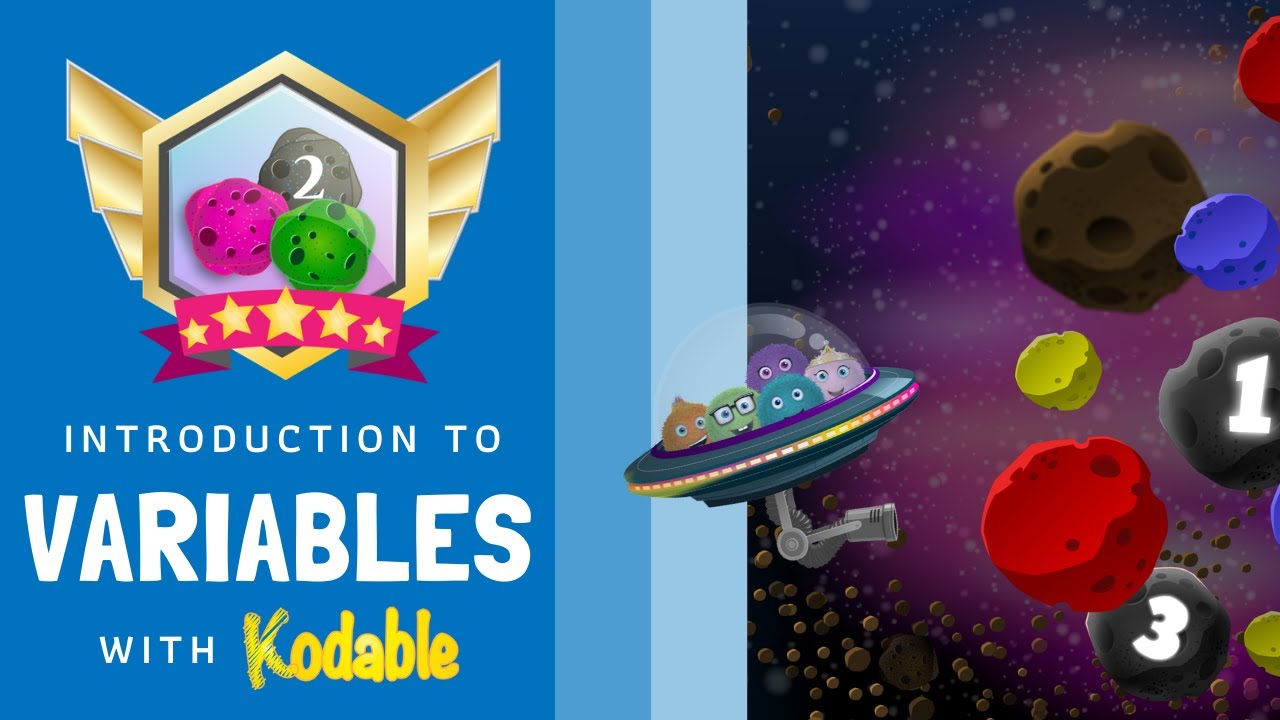
What are Variables? Coding for Kids | Kodable

Variables and Types of Variables | Statistics Tutorial | MarinStatsLectures
5.0 / 5 (0 votes)
Thanks for rating: