creating python modules 1 / Caesar cipher
TLDRThe video introduces Caesar cipher encryption in Python. It explains ASCII characters, ord() and chr() methods to convert between characters and decimal numbers. It then step-by-step builds a Caesar cipher function using these methods, taking plaintext and key inputs to shift characters by the key amount. It creates a CaesarCipher class with encrypt and decrypt methods, demonstrating shuffling plaintext by various keys and retrieving the original plaintext. Overall, it walks through implementing a basic substitution cipher for text encryption and decryption in Python using character ordinal values.
Takeaways
- π The lesson introduces the basics of the ASCII table, explaining it as the American Standard Code for dealing with English letters.
- π Each English character, both uppercase (A-Z) and lowercase (a-z), is represented by a unique decimal number in the ASCII standard.
- π The tutorial elaborates on how each uppercase letter from A (65) to Z (90) and each lowercase letter from a (97) to z (122) corresponds to ASCII decimal values.
- π The lesson demonstrates Python's 'ord' function, which converts a character to its ASCII decimal equivalent.
- π The counterpart 'chr' function is explained, showing how it converts ASCII decimal values back to characters.
- π An example encryption using a Caesar cipher is walked through, illustrating character-by-character encryption.
- π The video details the process of creating a simple encryption module in Python, focusing on the Caesar cipher methodology.
- π It explains the encryption formula where the cipher text is obtained by adding a key value to the plain text and applying modulo 26.
- π A decryption method is demonstrated, reversing the encryption process to retrieve the original plain text.
- π The lesson concludes by integrating these functions into a class structure, allowing for encapsulation of the Caesar cipher methods.
Q & A
What is the purpose of the ASCII table in Python programming?
-The ASCII table is used to represent English characters as numbers, allowing for character manipulation in algorithms, such as encryption and decryption processes.
How many characters are there in the English alphabet representation in the ASCII table?
-There are 52 characters representing the English alphabet in the ASCII table, including 26 uppercase (A-Z) and 26 lowercase (a-z) letters.
What is the decimal ASCII value for the uppercase letter 'A'?
-The decimal ASCII value for the uppercase letter 'A' is 65.
What Python method is used to convert a character to its ASCII decimal equivalent?
-The 'ord()' method is used to convert a character to its ASCII decimal equivalent.
How do you convert an ASCII decimal value back to its character representation in Python?
-The 'chr()' method is used to convert an ASCII decimal value back to its character representation.
What basic formula is used in the Caesar cipher algorithm discussed in the script?
-The basic formula used is 'cipher text = (plain text + key) mod 26', where 'plain text' is the input text, 'key' is the shift amount, and 'mod 26' ensures the result wraps around the alphabet.
How does the script handle encryption of a word using the Caesar cipher?
-The script encrypts a word by shifting each character by a specified key value according to the Caesar cipher algorithm, then converting the shifted values back to characters.
What is the purpose of using 'mod 26' in the Caesar cipher algorithm?
-Using 'mod 26' ensures that the character shifting wraps around the alphabet correctly, preventing index overflow and maintaining the encryption within the alphabet's bounds.
How can you decrypt a message encrypted with the Caesar cipher according to the script?
-To decrypt a message, you use the inverse of the encryption process, subtracting the key from the cipher text and applying 'mod 26' to find the original plain text.
Why is it important to convert the encryption algorithm into a class structure as mentioned in the script?
-Converting the encryption algorithm into a class structure organizes the code better, encapsulates functionality, and makes it reusable and easier to manage for different encryption and decryption tasks.
Outlines
π Introduction to ASCII and Caesar Cipher in Python
The lesson begins with an introduction to the ASCII table, explaining its significance as the American Standard Code for Information Interchange that represents English alphabetic characters, among others, with decimal values (e.g., 'A' is 65, 'B' is 66 up to 'Z' being 90 for uppercase, and 'a' to 'z' being 97 to 122 for lowercase). The focus then shifts to utilizing Python's 'ord' and 'chr' functions to convert characters to their ASCII values and vice versa. This foundation leads to an exploration of the Caesar cipher encryption method, using a simple algorithm that shifts characters by a specified key value. Through Python code examples, the process of encrypting a plain text (e.g., 'Gaza') by shifting each character's ASCII value and then converting these values back to characters to form the cipher text is demonstrated. The key concept of modular arithmetic ('mod 26') is introduced to ensure the shifts wrap around the alphabet correctly.
π Implementing and Deciphering Caesar Cipher
Building on the encryption foundation, this section details the implementation of a Caesar cipher in Python, focusing on a function that encrypts plain text (e.g., 'Gaza') by shifting its characters according to a given key. The narrative then transitions to the decryption process, which reverses the encryption by shifting the characters back using the negative value of the key. This is exemplified through a Python function that takes the cipher text and the key as inputs and returns the original plain text. The discussion culminates in the creation of a Python class encapsulating the encryption and decryption methods, making the Caesar cipher implementation more structured and reusable. The class, named after the cipher, provides a clear, concise way to employ the algorithm, highlighting the ease of encoding and decoding messages using this ancient but still illustrative cryptographic technique.
Mindmap
Keywords
π‘ASCII table
π‘Python module
π‘ord function
π‘chr function
π‘Encryption
π‘Caesar cipher
π‘Decryption
π‘Plain text
π‘Cipher text
π‘Key
Highlights
Introducing first lesson in Python course on Ceasar Cipher encryption
Explaining ASCII table - mapping letters to decimal numbers
Demonstrating ord() and chr() functions to convert between letters and ASCII values
Encrypting word 'Gaza' by shifting letters using key
Creating CesarCipher class with encrypt and decrypt methods
Showing class works to encrypt and decrypt words in upper and lower case
Transcripts
Browse More Related Video
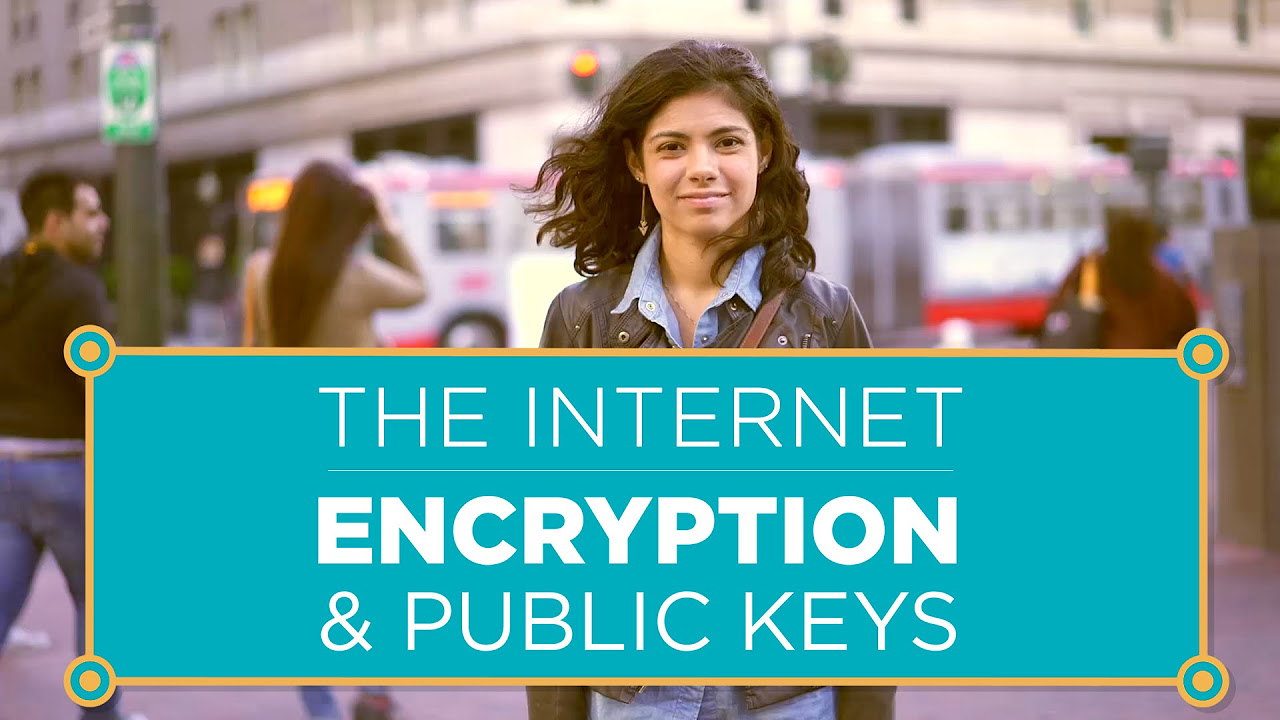
The Internet: Encryption & Public Keys
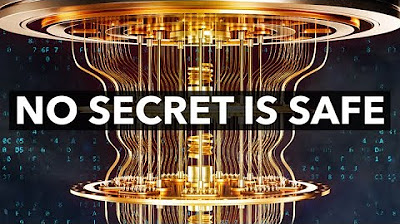
How Quantum Computers Break The Internet... Starting Now

Stem and Leaf Plots

Second Derivative of Parametric Equations with Example
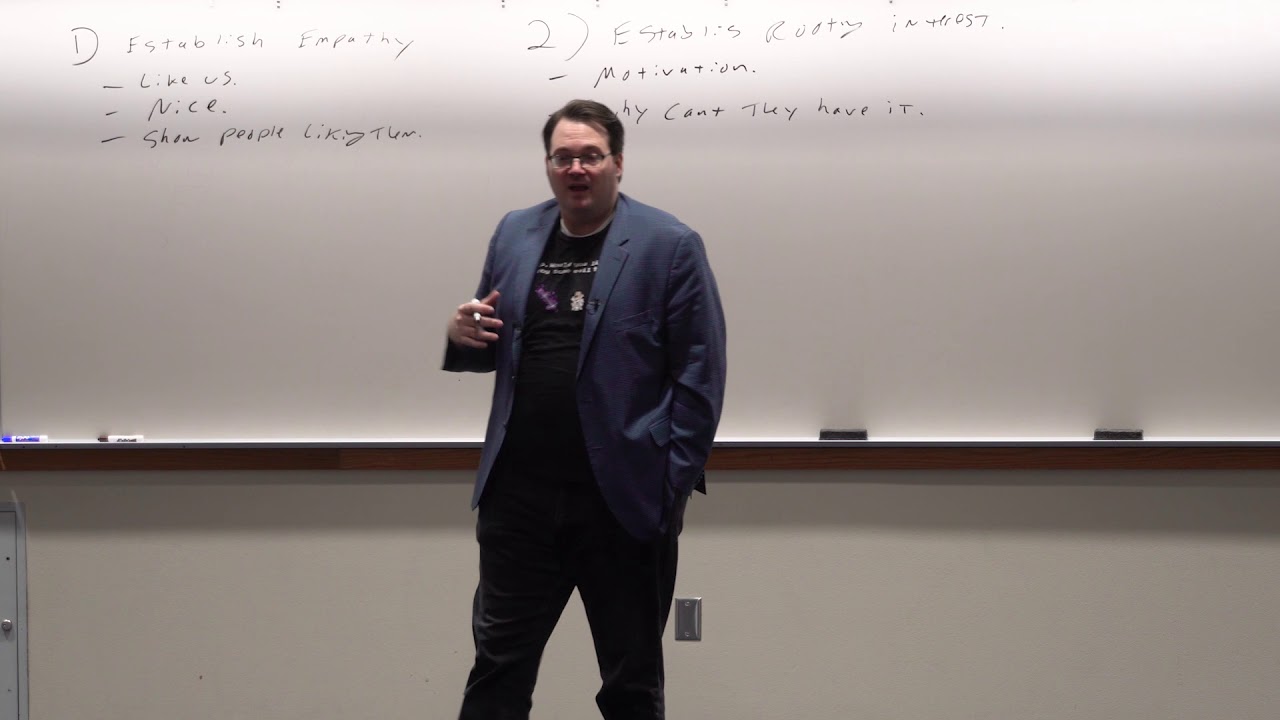
Lecture #9: Characters β Brandon Sanderson on Writing Science Fiction and Fantasy
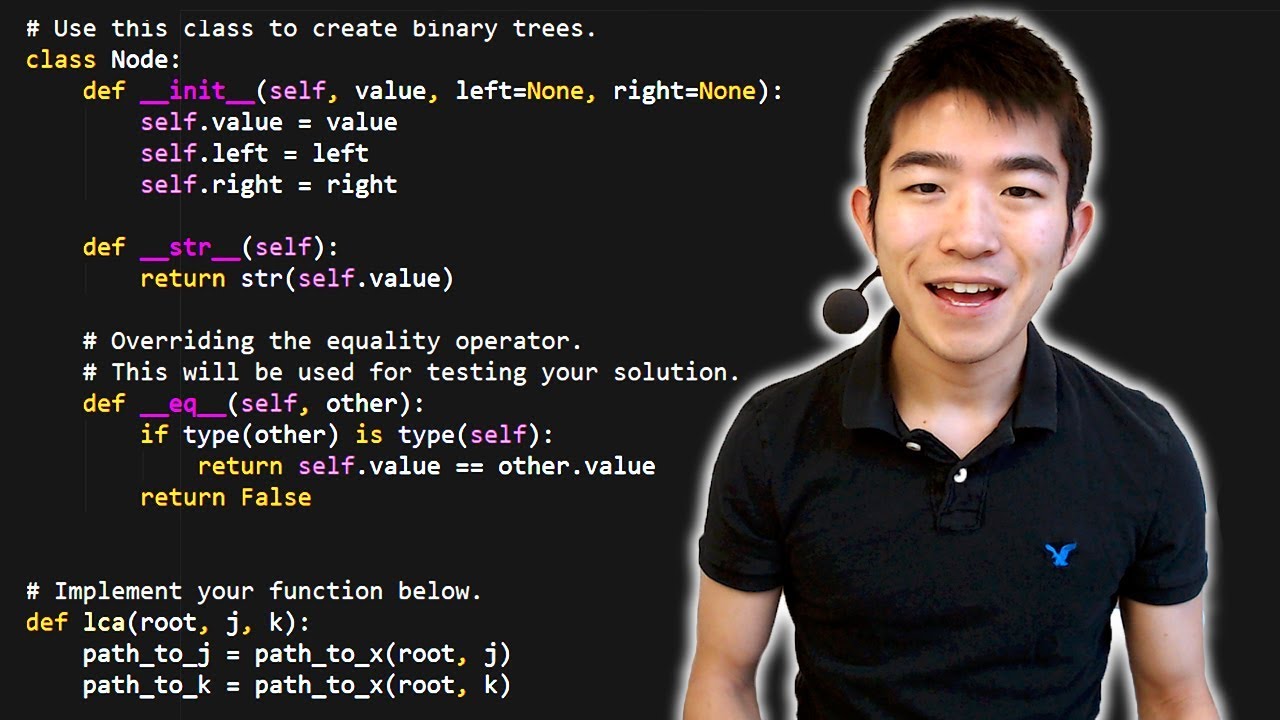
Python Tutorial for Absolute Beginners #1 - What Are Variables?
5.0 / 5 (0 votes)
Thanks for rating: