What is a Monad? - Computerphile
TLDRThis script explores the concept of monads in programming, introduced in mathematics in the 1960s and rediscovered in computer science in the 1990s. It uses Haskell to demonstrate how monads, specifically the Maybe monad, can handle effects like failure in a pure programming language, emphasizing the importance of monads in modern programming languages.
Takeaways
- π Monads are a mathematical concept from the 1960s that were rediscovered in computer science in the 1990s, offering a new perspective on programming with effects.
- π‘ The presenter introduces monads using Haskell, a programming language, to demonstrate how they can be used to handle effects like division by zero safely.
- π’ The script defines a simple data type `Expr` in Haskell to represent expressions that can be either an integer value (`Val`) or a division of two sub-expressions (`Div`).
- π The example of evaluating expressions is used to illustrate how monads can manage potential failures, such as division by zero, without crashing the program.
- π οΈ A safe division function `safediv` is introduced, which returns a `Maybe Int` to handle the possibility of division by zero, where `Nothing` represents failure and `Just` represents a successful result.
- π The concept of a monad is explained as a type constructor (like `Maybe`) and two functions (`return` and a sequencing operator) that allow for chaining operations that may fail.
- π The script shows how to abstract common patterns in code, such as handling `Maybe` values, into a monadic structure, simplifying the code and making it more readable.
- π The use of `do` notation in Haskell is introduced as a syntactic sugar that simplifies the writing of monadic code, making it more intuitive and easier to understand.
- π The idea of monads is applicable to various effects in programming, not just failure handling, but also input/output, mutable state, environment reading, logging, and non-determinism.
- π The importance of monads in programming languages is highlighted, emphasizing their role in supporting pure programming with effects, explicit effect usage in types, and the ability to write functions that work for any effect (effect polymorphism).
Q & A
What is the main concept discussed in the script?
-The main concept discussed in the script is monads, a programming concept that originated in mathematics and was later adopted in computer science, particularly in programming languages like Haskell, to handle effects in a more structured way.
Why were monads invented in mathematics and later rediscovered in computer science?
-Monads were invented in mathematics in the 1960s as a way to handle certain types of mathematical structures. They were rediscovered in computer science in the 1990s because they provide a powerful way to model computations with effects, such as input/output, in a pure functional programming context.
What programming language is used in the script to illustrate the concept of monads?
-The programming language used in the script to illustrate the concept of monads is Haskell, a purely functional programming language known for its expressiveness and strong static typing.
What is the purpose of the 'Maybe' type in Haskell?
-The 'Maybe' type in Haskell is used to handle computations that may fail, such as division by zero. It encapsulates a value that could either be 'Just' a value, indicating success, or 'Nothing', indicating failure, thus providing a way to avoid runtime errors.
What is the 'safediv' function in the script and what does it do?
-The 'safediv' function is a safe version of the division operator defined in the script. It takes two integers and returns a 'Maybe Int'. If the second integer (the divisor) is zero, it returns 'Nothing' to indicate failure; otherwise, it returns 'Just' the result of the division.
What is the issue with the original evaluator program for expressions?
-The issue with the original evaluator program is that it may crash during execution if it attempts to divide by zero. This is because the program does not handle the case where the divisor is zero, which leads to a runtime error.
What is the 'do' notation used for in Haskell?
-The 'do' notation in Haskell is syntactic sugar that simplifies the process of writing monadic code. It allows programmers to write sequences of actions in a more readable and less verbose way, abstracting away the explicit case analyses and handling of monadic values.
What is the significance of the 'return' function in the context of monads?
-The 'return' function in the context of monads is used to lift a pure value into a monadic context. It takes a value of any type and wraps it in a monadic value, such as 'Just' for the 'Maybe' monad, providing a bridge from pure to impure computations.
What are the benefits of using monads for programming with effects?
-Monads provide a uniform framework for handling different kinds of effects in programming, support pure programming with effects, make the use of effects explicit in the types, and allow for writing functions that work with any effect, promoting code reusability and maintainability.
Why is the term 'monad' considered difficult or intimidating by some programmers?
-The term 'monad' is considered difficult or intimidating by some programmers because it is a mathematical term that may not be familiar to those without a background in category theory. Additionally, the concept itself can be abstract and challenging to grasp, especially for those new to functional programming.
How does the script address the learning curve associated with monads?
-The script acknowledges the learning curve and the potential difficulty in understanding monads due to their mathematical origins and terminology. It suggests that despite the initial complexity, understanding monads is essential as they are fundamental to programming languages like Haskell, and it encourages learning the proper terms to honor the origins of the concept.
Outlines
π Introduction to Monads in Programming
The first paragraph introduces the concept of monads, which originated in mathematics in the 1960s and were later rediscovered in computer science in the 1990s. The speaker emphasizes the importance of monads in programming languages, particularly in the last 25 years. The focus is on programming with effects using a simple example in Haskell. The example involves writing a function to evaluate expressions, specifically those involving integer values and division. The speaker assures that even those unfamiliar with Haskell will be able to follow along as everything will be explained step by step. The initial step is defining a data type for expressions using Haskell's `data` keyword, with two constructors: `Val` for integer values and `Div` for division of sub-expressions.
π Safe Division and Maybe Type in Haskell
The second paragraph delves into the problem of division by zero in the context of the expression evaluation example. To address this, the speaker introduces a safe division function called `safediv`, which returns a `Maybe Int` type. This function checks if the divisor is zero and returns `Nothing` if so, or `Just` the result of the division otherwise. The `Maybe` type in Haskell is used to handle potential failures, such as division by zero, by allowing for a value to either be present (`Just`) or absent (`Nothing`). The speaker then modifies the expression evaluator to handle these `Maybe` values, ensuring that the program does not crash and instead returns a well-defined result.
π Abstracting Patterns with Monads
In the third paragraph, the speaker identifies a common pattern in the safe evaluator program: the repeated use of case analysis on `Maybe` values. To simplify the program, the speaker abstracts this pattern into a definition, introducing a sequencing operator that allows for chaining operations that might fail. This operator checks if the first operation succeeds (`Just`) and, if so, applies a function to the result. If the operation fails (`Nothing`), the entire sequence fails. This abstraction leads to a cleaner and more concise program, making it easier to understand and maintain.
π‘ The Maybe Monad and Its Benefits
The fourth paragraph explains the concept of the Maybe monad, which consists of the `Maybe` type constructor and two functions: `return` and the sequencing operator. The speaker discusses the benefits of using monads, such as providing a uniform framework for programming with effects, supporting pure programming with effects, making the use of effects explicit in the types, and enabling effect polymorphism. The speaker also mentions the importance of monads in programming language development and suggests further reading in their book 'Programming in Haskell' for a deeper understanding.
π Learning Monads and Their Role in Haskell
The final paragraph addresses the challenges faced by learners when encountering monads and other abstract concepts in Haskell. The speaker argues for the importance of using proper terminology like 'monad' and credits mathematicians for their original discovery. They acknowledge the difficulty these terms can pose for learners but encourage embracing the terminology. The speaker also mentions the availability of libraries in Haskell that implement various monads, reducing the need for programmers to define their own. The paragraph concludes by highlighting the built-in support for monads in Haskell and the potential for using multiple monads in a single program.
Mindmap
Keywords
π‘Monads
π‘Haskell
π‘Effects
π‘Maybe Type
π‘Safe Division
π‘Evaluator
π‘Recursion
π‘Do Notation
π‘Type Constructors
π‘Effect Polymorphism
Highlights
Monads were invented in mathematics in the 1960s and rediscovered in computer science in the 1990s.
Monads provide a new way of thinking about programming with effects.
The concept will be explored using Haskell and simple expression evaluation.
A datatype for expressions is defined using Haskell's data keyword.
Expressions can be either an integer value or a division of two sub-expressions.
The Expr datatype is constructed with Val and Div constructors.
Examples are given to illustrate how mathematical expressions are represented in Haskell.
An evaluator function is introduced to compute the integer value of expressions.
The evaluator handles two cases: integer values and divisions.
Division by zero is addressed by introducing a safe division function.
The safediv function returns Maybe an integer to handle potential failures.
The Maybe type is used to represent computations that might fail.
The evaluator is rewritten to handle failures using the Maybe type.
The program's verbosity is reduced by abstracting common patterns.
The Maybe monad is introduced as a way to handle computations that can fail.
Monads provide a uniform framework for programming with effects.
Monads support pure programming with effects in languages like Haskell.
Effects are made explicit in the types in monadic programming.
Effect polymorphism allows writing functions that work for any effect.
Haskell has libraries for programming with monadic constructs.
The term 'monad' can be challenging for learners due to its mathematical origin.
Transcripts
Browse More Related Video
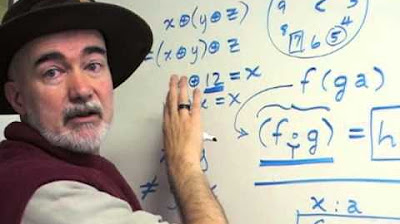
Brian Beckman: Don't fear the Monad
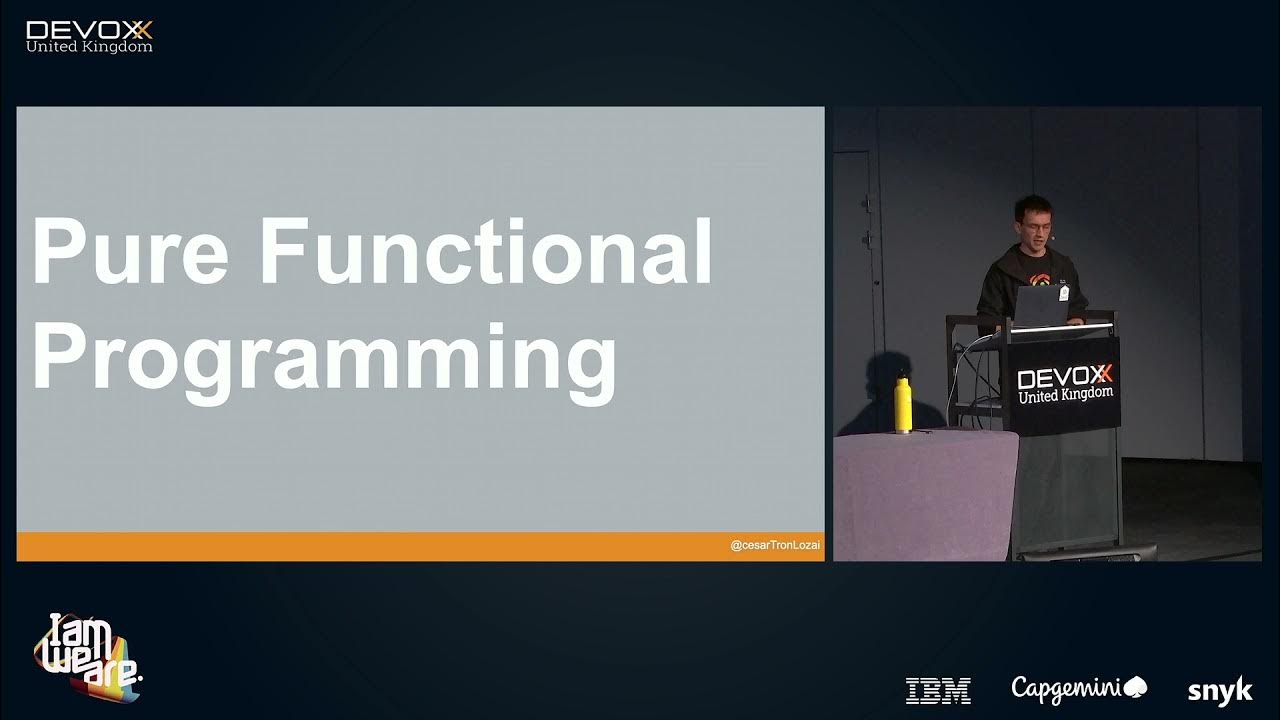
No Nonsense Monad & Functor - The foundation of Functional Programming by CΓ©sar Tron-Lozai
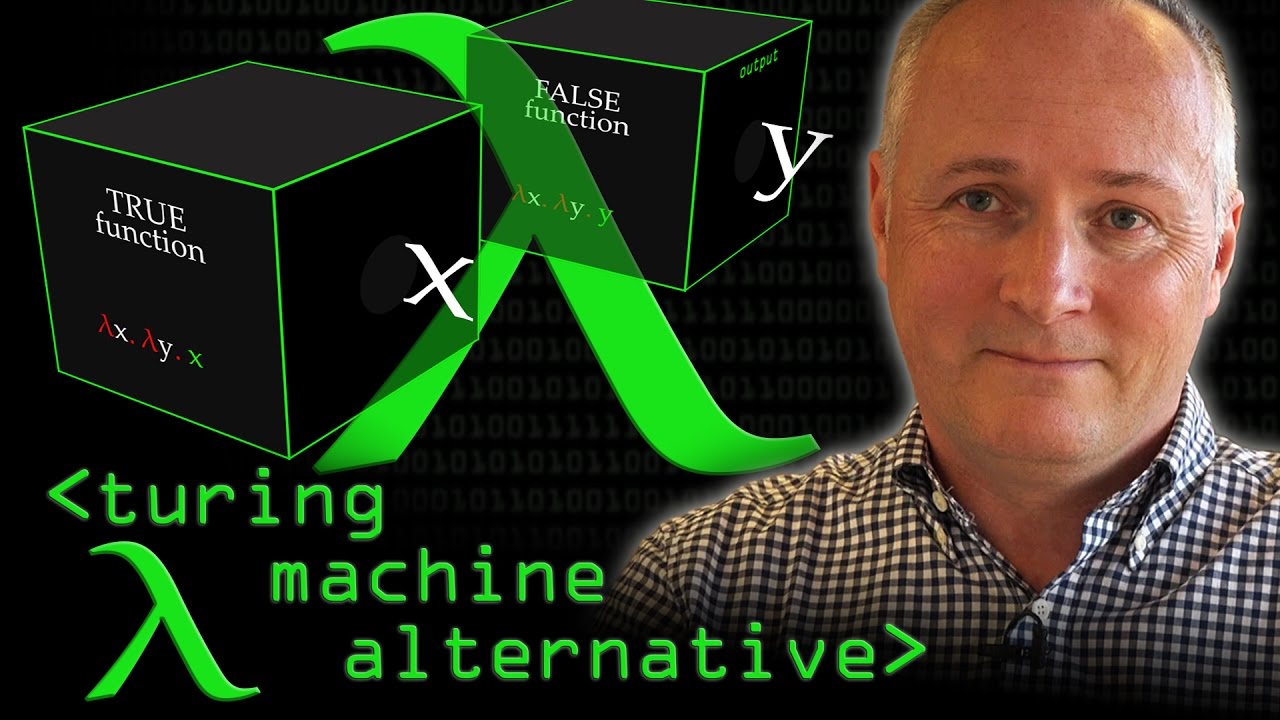
Lambda Calculus - Computerphile

2.1 Computing Basics
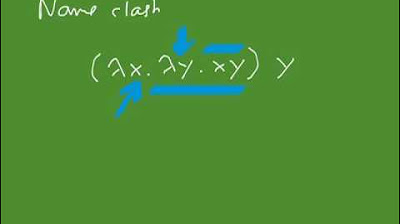
The Lambda Calculus, part 1 1 Syntax and semantics
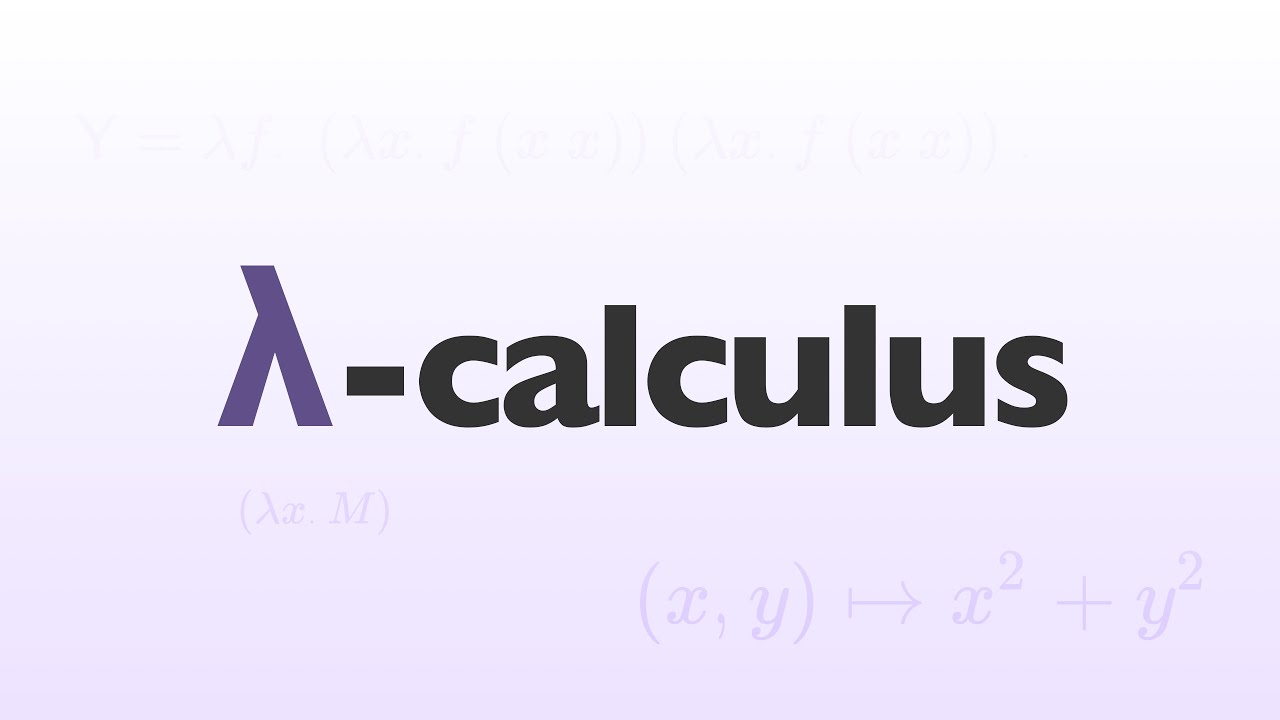
Lambda Calculus: The foundation of functional programming, and the simplest programming language
5.0 / 5 (0 votes)
Thanks for rating: